Control statements-In JavaScript.
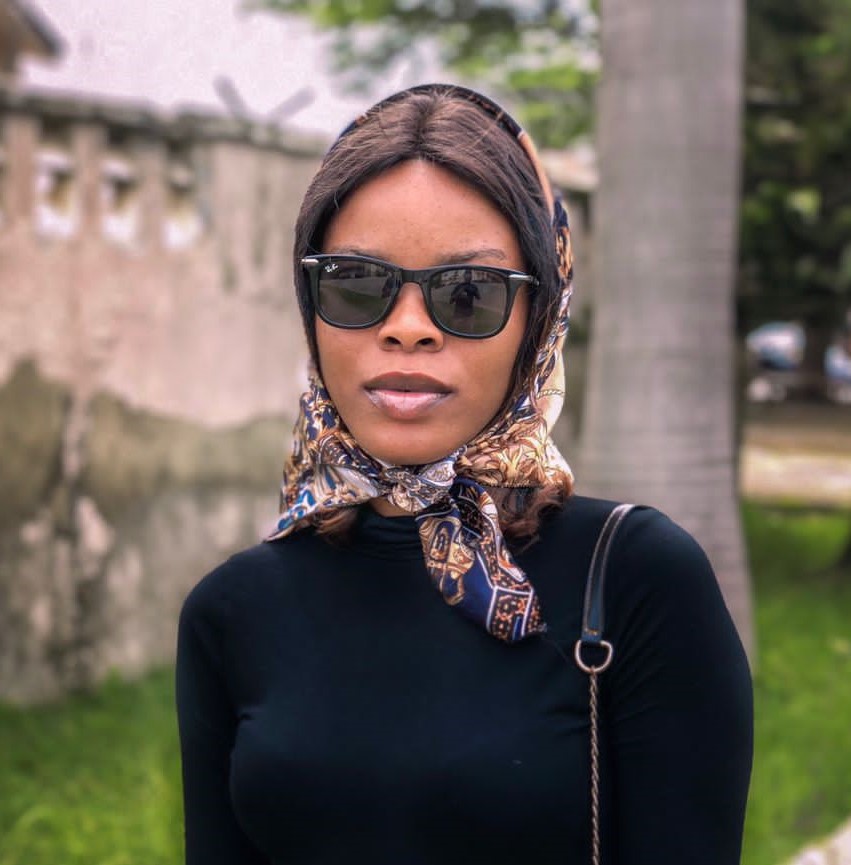
Table of contents
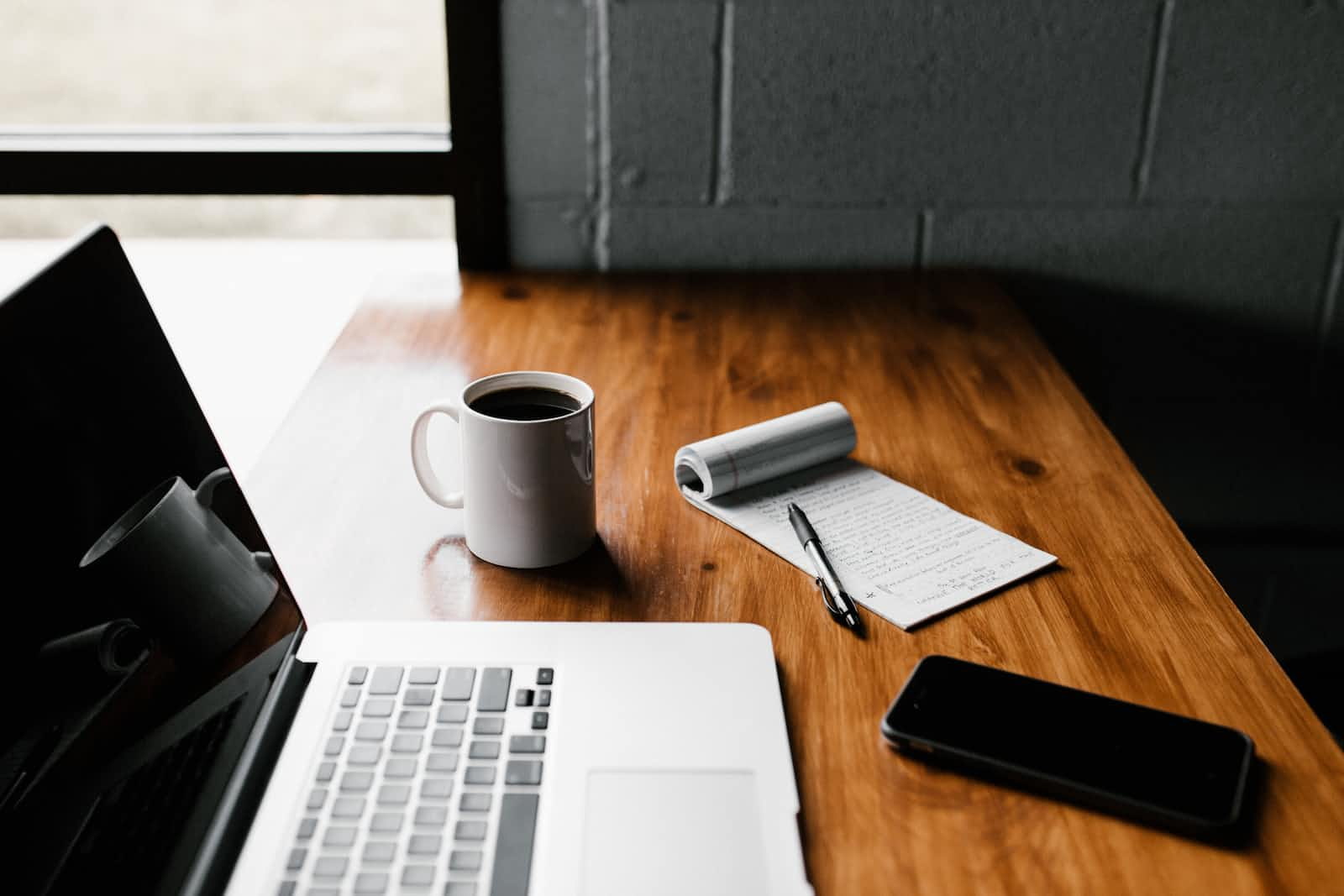
Hello guys! In this article, we will be talking about Control-Statements in JavaScript. Before we get into the juicy details of how fun and how useful it is to have these statements. Let's get the basic definition of what control flow is in any other programming language.
DEFINITION
Control flow is the order in which the computer executes statements in a script. Therefore, a JavaScript control statement is used to control the flow of the program based on the specified condition.
Brief Explanation
Code is run in the order of top to bottom, unless the computer runs across structures that change the control flow, such as conditionals and loops. In this article, we will be focused on the Conditionals.
JavaScript Statements
- if statement
- else if statement
- else statement
if statement
if statement is used to specify a block of JavaScript code to be executed if a condition is true.
SYNTAX
if(condition){
//Run this block of Code
}
else if statements
else if statement is used to execute two blocks of codes depending on the condition. If condition-1 is true then if block will execute otherwise else if block will execute.
SYNTAX
if (condition){
//Run this block of code
} else if(condition){
//Run this block of code
}
else statements
If else statement is used to execute one block of code depending upon the condition. If condition1 is true then the block of code for statements1 will be executed, else if condition2 is true then the block of code for statements2 is executed, and so on. Now, you can have as many else if statements as you want to signify the conditions you are laying out. If no condition is true, then else block of statements will be executed.
SYNTAX
if(condition1){
//Run this block of JavaScript Code
}
else if(condition2){
////Run this block of JavaScript Code
}
else if(condition3){
//Run this block of JavaScript Code
}
else{
//Do this
}
Example
const greeting();
function time(){
if (time < 10) {
greeting = "Good morning";
}
else if (time < 15 ){
greeting = "Good day"
}
else if (time < 20) {
greeting = "Good afternoon";
}
else {
greeting = "Good evening";
}
}
JAVASCRIPT SWITCH STATEMENTS
There is also an interesting Control statement in JavaScript known as the SWITCH STATEMENT. My personal and absolute favorite. the switch statement is used to specify many alternatives blocks of code to be executed. The switch statement is also used to perform different actions based on different conditions.
SYNTAX
It starts with the keyword switch followed by parentheses, Inside the parentheses, we have the condition in which the code will be executed, and the cases (conditions) We want each code to run by.
switch(condition) {
case x:
// code block
break;
case y:
// code block
break;
default:
// code block
break;
}
I'm certain the syntax looks funny.... but I promise it's very easy to understand Just like the If statements, it's simply saying: in case x: check if the condition is true and then Run this block of code it starts with the keyword switch rather than the if So we've
switch (condition){
case x :
//Run this block of code
break;
}
The break keyword at the end of every case simply means break out of this switch block after running in this condition. When JavaScript reaches a break keyword, it breaks out of the switch block. This will stop the execution inside the switch block. It is not necessary to break the last case in a switch block, which is the default block. The block breaks (ends) there anyway.
Now I know I am clouding you with a lot, but I promise you just read through the article again and you will begin to understand it.
The default keyword
This keyword is simply like the else statement
So basically, I'm saying after all these cases and nothing matches (Which will probably be rare) fall back to this default.
For more resources on JavaScript Control Statements Visit https://www.w3schools.com/js/js_switch.asp, https://developer.mozilla.org/en-US/docs/Glossary/Control_flow
Hope this helps
Happy Coding
Subscribe to my newsletter
Read articles from Heavenlydawn Gabriel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
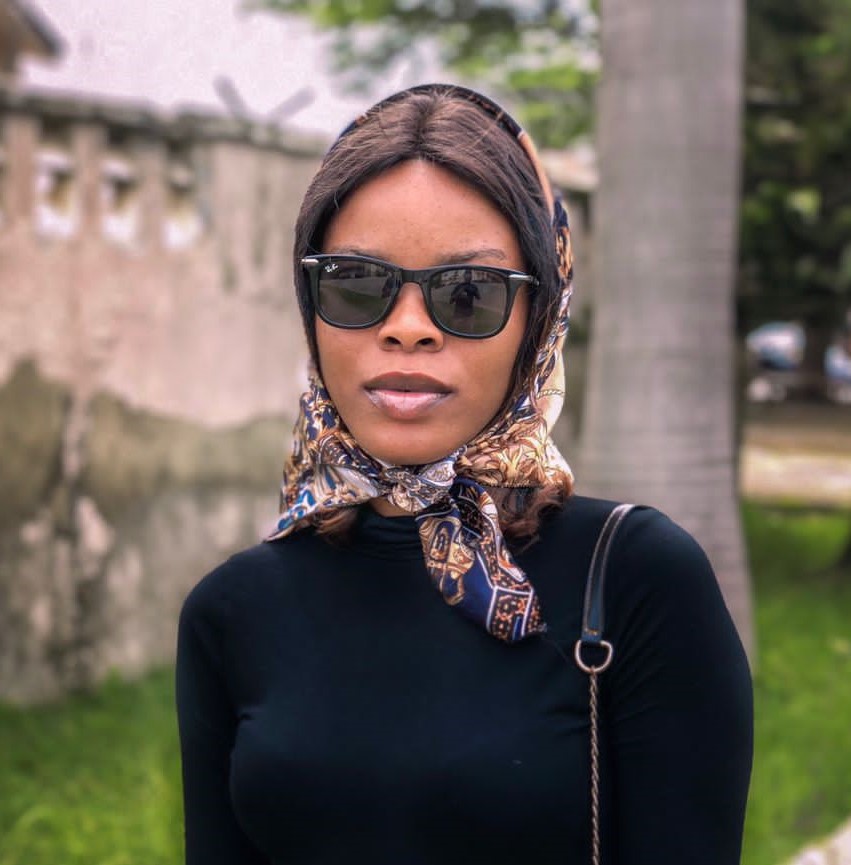