Why loops? ๐คAnd loops in Java.
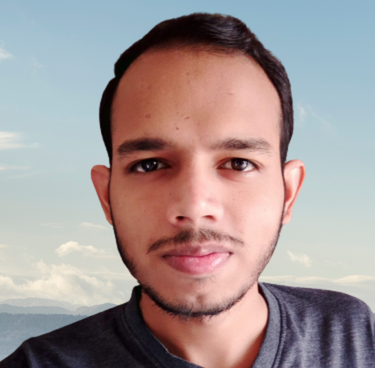
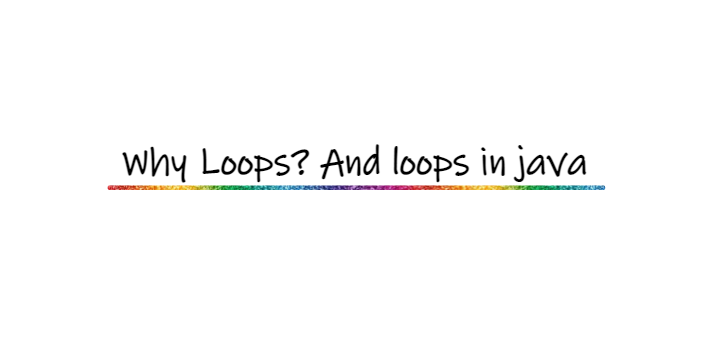
Let's say I tell you to print something like this.
*
Then the simplest way to do that would be this
System.out.println("*");
But my friend if I want you to print something like this
*
*
You will definitely do this
System.out.println("*");
System.out.println("*");
Now I want you to print the star 1000 times. Then using the System.out.println("*");
is not a good option.
A great person said that -
A good code is code that does not repeat itself.
That leads to the creation of loops so that we can do the repetitive work efficiently, with a little amount of coding.
General inputs in the case of loops are ->
- Initialization: It is the initial condition which is executed once when the loop starts. Here, we can initialize the variable, or we can use an already initialized variable. It is an optional condition.
- Condition: It is the second condition which is executed each time to test the condition of the loop. It continues execution until the condition is false. It must return boolean value either true or false. It is an optional condition.
- Increment/Decrement: It increments or decrements the variable value. It is an optional condition.
- Statement: The statement of the loop is executed each time until the second condition is false.
Different types of loops in Java :
- for loop in Java
for ( initialization ; condition ; increment/ decrement ) {
// code to be executed
statement
}
test code
for ( int i = 0 ; i < 5 ; i++ ){
System.out.println("*");
}
test code output
*
*
*
*
*
- while loop in Java
while ( condition ){
//code to be executed / statement
increment / decrement statement
}
test code
int i = 0 ;
while ( i < 5 ) {
System.out.println("*");
i++;
}
test code output
*
*
*
*
*
- do while Loop in Java
do{
//code to be executed / statement
increment / decrement statement
}while (condition);
int i = 0 ;
do {
System.out.println("*");
i++;
} while ( i < 5 );
test code output
*
*
*
*
*
Hope it was help full, see you in the next Blog. Till then , bye bye ๐.
Subscribe to my newsletter
Read articles from Niraj Kumar Gangale directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
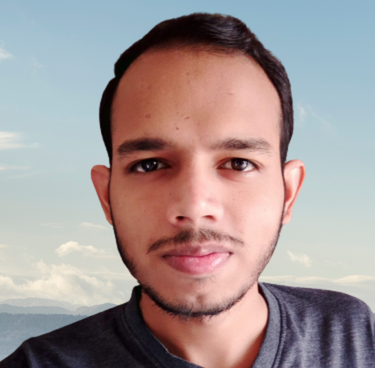
Niraj Kumar Gangale
Niraj Kumar Gangale
it's Secret shhhh!!! ๐ถ