Data Structure: Single Linked List

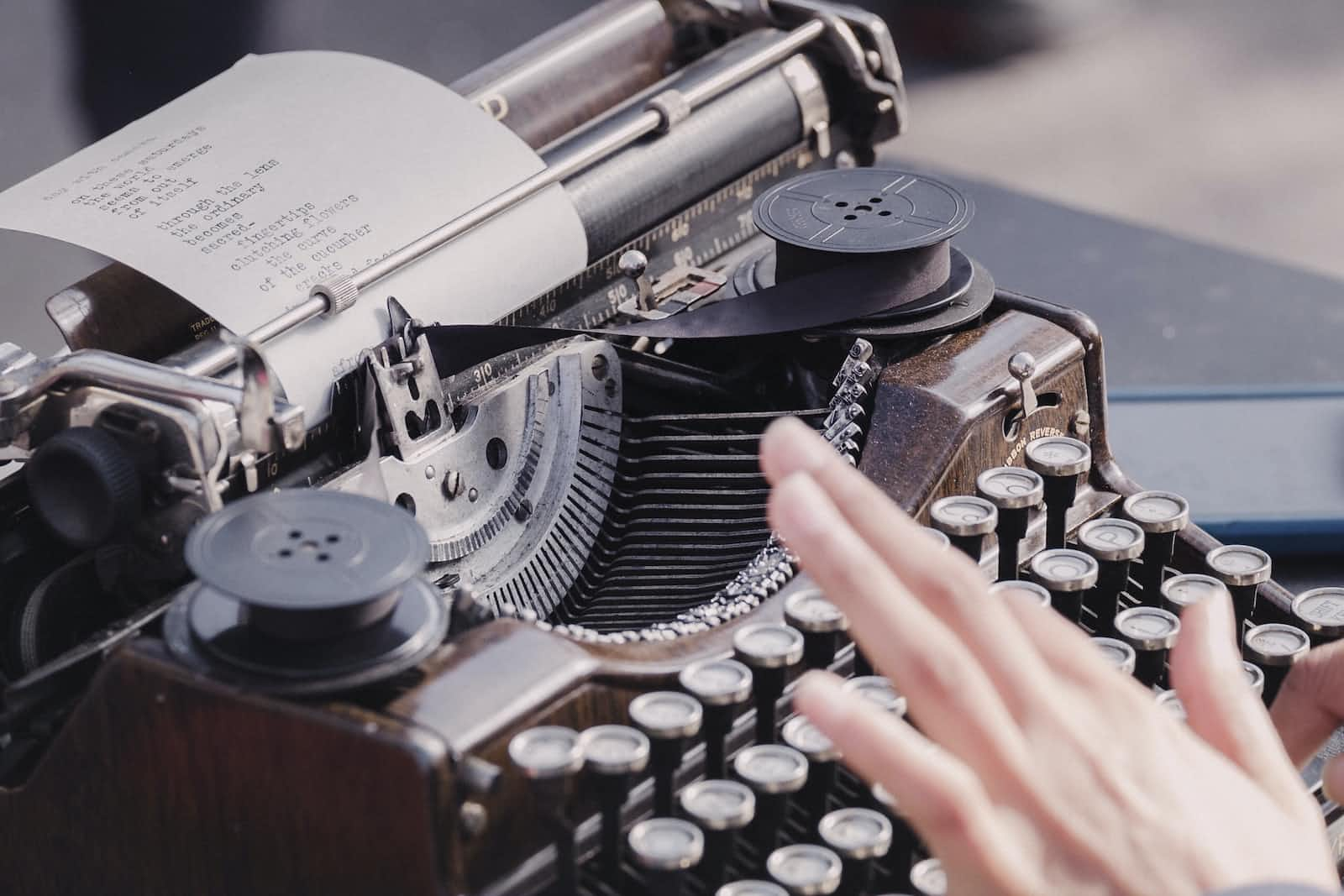
Before jumping into the singly linked list, let's see an overview of linked lists.
"A linked list is a linear data structure in which elements are not stored in contiguous memory.The elements in the linked list are linked using pointers".
Here, elements are nothing but nodes, where each node has two parts :
Singly Linked list: It is the same as a linked list, where nodes can be traversed unidirectionally; therefore, we cannot traverse the list in the reverse direction.
Complexities -> O(n)
O(n)
Basic operation:
1. Insertion :
insertNode(data: number) {
const node = new Nodes();
node.data = data;
node.next = this.head;
this.head = node;
}
Above code will insert the node from beginning, so:
- Allocate memory for new node
- Store data
- Change next of new node to point to head
- Change head to point to recently created node
2. Deletion :
deleteNode(position: number) {
let current = this.head;
for(int i=2; i< position; i++) {
if(current ->next!=NULL) {
current = current ->next;
}
}
current ->next = current ->next->next;
}
- Traverse to element before the element to be deleted
- Change next pointers to exclude the node from the chain
3. Traverse:
traverse() {
let current = this.head;
while (current != null) {
console.log(current.data);
current = current.next;
}
}
Displaying the contents of a linked list is very simple. We keep moving the current node to the next one and display its contents. When current is NULL, we know that we have reached the end of the linked list so we get out of the while loop.
Subscribe to my newsletter
Read articles from Aravind directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
