Arrow functions In javaScript (=>)

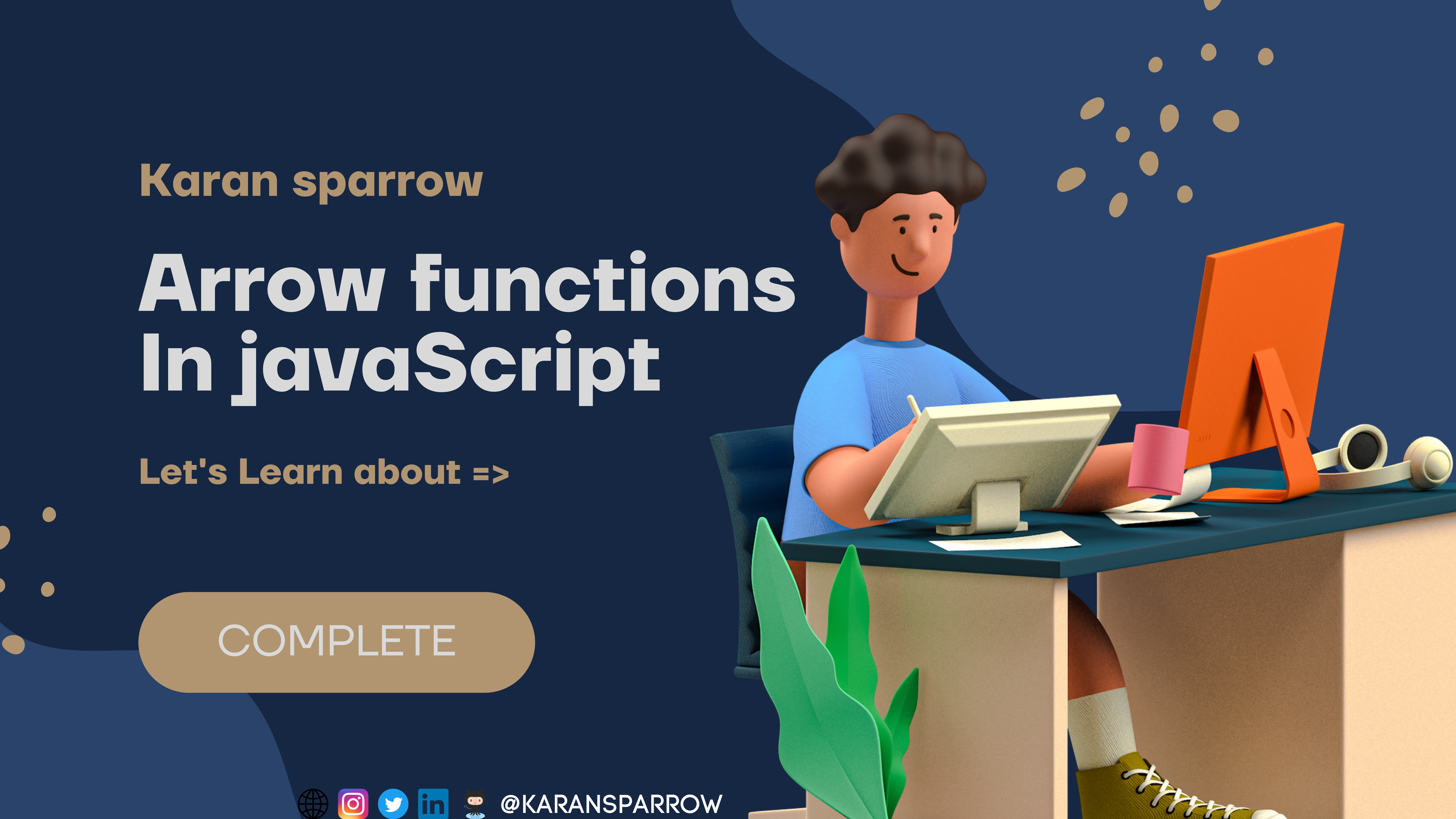
Arrow functions In javaScript. That is a different syntax for writing Javascript functions.
function myFunc(){
//code here
}
A typical javascript function looks like this. Using the keyword function. You may also notice a syntax where var myFunc equals function.
Const myFunc = ( ) => {
//code here
}
An arrow function now looks like this. I've put it in a constant and then on the right side of the equals sign. That is the syntax for the arrow function. There is no list of arguments here, but it could hold some arguments and then an arrow. The function body is followed by an equal sign and a greater than sign. The arrow function syntax is slightly shorter than the standard syntax because it omits the function keyword, which is a significant advantage. It solves a lot of the problems you used to have.
Javascript contains this keyword. If you've worked with javascript before, you're probably aware that this keyword does not always refer to what you might expect it to refer to while writing your code. When used within an arrow function, it will always keep its context and will not change unexpectedly at runtime. Let's take a look at that arrow function syntax. In actuality.
function sayHello(greet) {
console.log(greet)
}
sayHello('hello everyone')
Let's make a simple function, sayHello (greet)
Of course, we can output console log greet and pass greet as an argument.
We get hello everyone if I call sayHello('hello everyone').
The equivalent of the arrow function is to save it as a constant named sayHello. It could also be made using let. SayHello as a constant if you intend to re-assign this variable.
const sayHello = (greet) => {
console.log(greet)
}
sayHello('hello everyone't)
Remember to include this arrow between the list of arguments and the function body. If you run this now, you will still see hello everyone. So it behaves exactly the same way here.
This keyword thing is interesting. It becomes significant when you add functions to objects. First and foremost, let me show you some alternatives to this syntax in terms of the argument list. To be more specific. If you only get one argument, as we do here, you can save time by removing the parentheses around it.
const sayHello = greet => {
console.log(greet)
}
sayHello('hello everyone't)
However, that is only true for one argument, not for more or less. You will still get the same result here. If you had a function that didn't take any arguments. This is not the correct syntax.
const sayHello = () => {
console.log(greet)
}
sayHello('hello everyone't)
You must use an empty pair of parentheses, as shown above. If we do this now, we will still see hello everyone. So this works if you have multiple arguments. Parentheses are also required.
const sayHello = day, night => {
console.log(greet)
}
sayHello('hello everyone't)
So day and night will not work. This must be enclosed in parentheses.
const sayHello = (day, night) => {
console.log(greet)
}
sayHello('have a nice day', 'good night')
You can now output , day, and night as arguments here. So these are two different syntaxes for arguments that you might encounter. There is another option. Regarding the function body.
So to the right of the arrow. Obviously, many functions simply return something.
So, say we want to multiply something and we have a number as an argument.
const multi = (number) => {
return number *2
}
console.log(multi(3))
Now we want to return the number multiplied by two. Obviously, we can console log the results of multiplying. So, what is the result of multiplying by three? Now run this, and we should see 6, which we do.
If all you do in your function body is return and there is no other code in there, you can omit the curly braces and write this in one line, but you must also omit the return keyword. This is a very condensed version of how to write this function.
const multi = (number) => number * 2;
console.log(multi(3))
It becomes a little shorter if we use the shortcut of removing the parentheses around the single argument. And what this now does is return the result of this code. We simply omit the return keyword and must omit it. And we have a very short and concise way of writing a function that accepts one or more arguments and returns something. So, clearing and running still yield 6. So that's all the syntax you'll see, and the arrow function in general will be used a lot. You saw the different syntaxes. You are not required to remember all of them. Just be aware that there are various syntaxes, and if we use them, they will quickly return to your mind, and you will understand why we use a particular syntax.
Thank you for reading!
Check out my profiles on these platforms.
Subscribe to my newsletter
Read articles from Karan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Karan
Karan
Hey, I'm Karan! A passionate Fullstack developer from India