Type Script Concepts
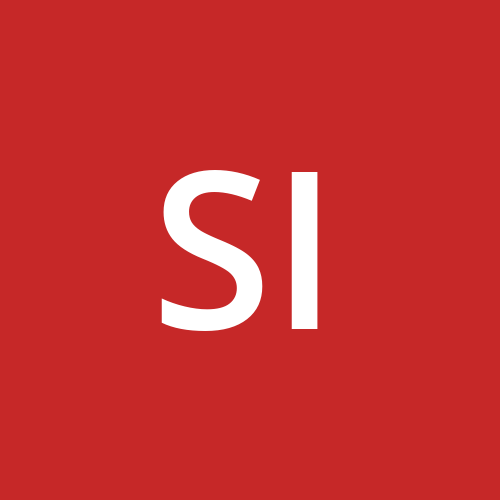
2 min read
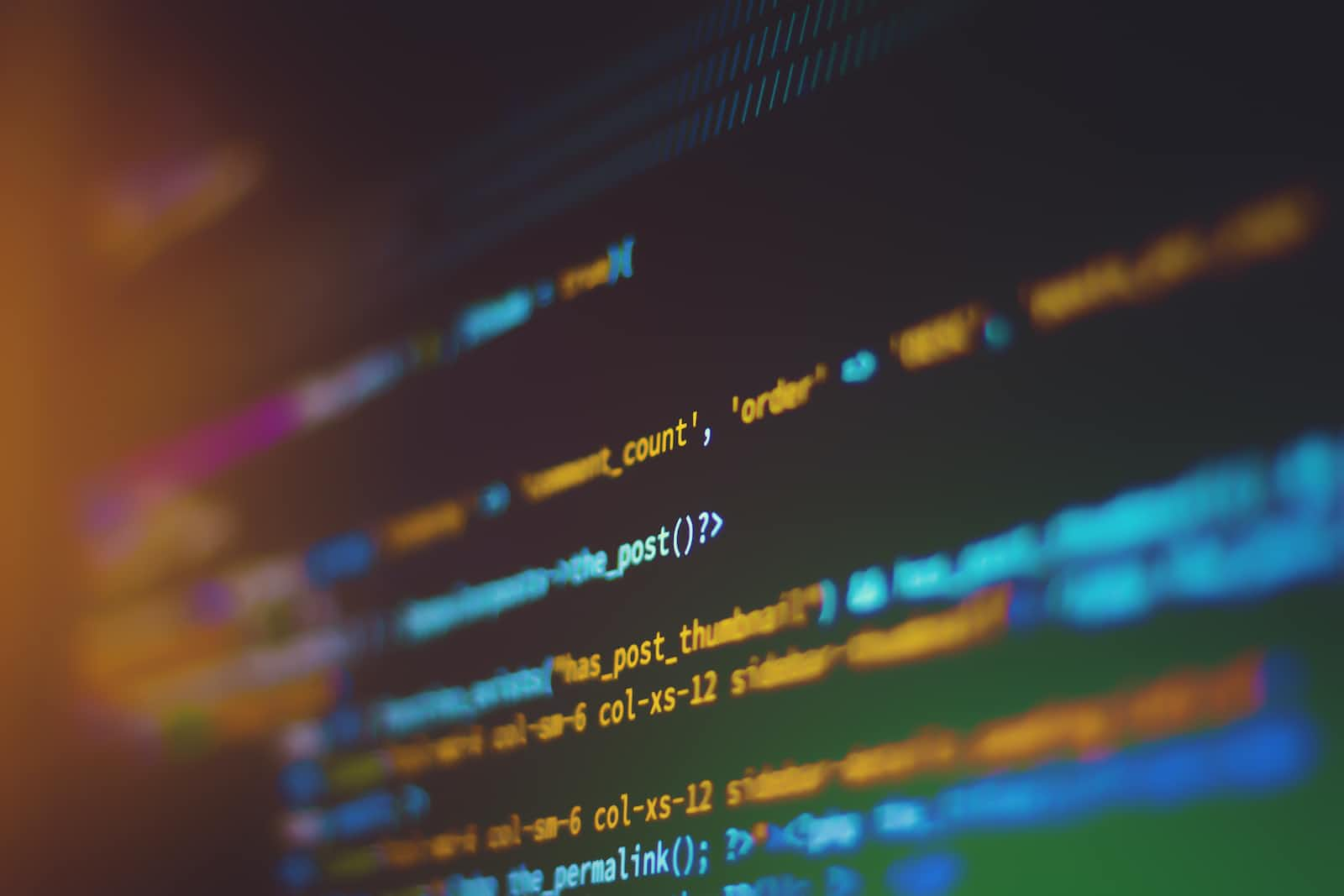
Type Script Introduction
- Superset of JavaScript, JavaScript that Scales.
- Perfect for big project and production grade applications.
- Saves you from lot of errors
Typescript Installation
- Similar to normal react app installation
npx create-react-app app-name --template typescript
- to add type script to an existing React application
npm install --save typescript @types/node @types/react @types/react-dom @types/jest
Types and declaring in application
- Mention type at the time of declaration.
let name:string;
let age:number;
let isAdult:boolean;
name="ravi";
age=25;
isAdult=true;
- Cannot assign number to name it's type error
name=5; //error
Array of string and number
let hobbies:string[];
hobbies = ['book reading', 'cycling', 'swiming'];
let ages:number[];
ages = [25,35,45,55];
Tuple
let role :[number,string];
role=[5,'ravi'];
role=[5,5]; //error
Object
let person:object;
- Above is not recommended way to create because types inside object are not clear
type Person = {
name : string;
age ?: number;
}
//or
interface Person{
name:string;
age?:number;
}
let person:Person = {
name:'ravi',
age:25; //optional
}
Union
- Multiple type for one variable
let age : number | string;
age = 'twenty'; //fine
age = 20 //fine
Function
let printAge:(age:number)=> void;
printAge = (age)=>{
console.log(age);
}
Type: any and unknown
- Any type is not recommended
let age:any; // any type string or number etc
- Unknown is recommended
let age:unknown;
0
Subscribe to my newsletter
Read articles from srinivasa ravi Idury directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
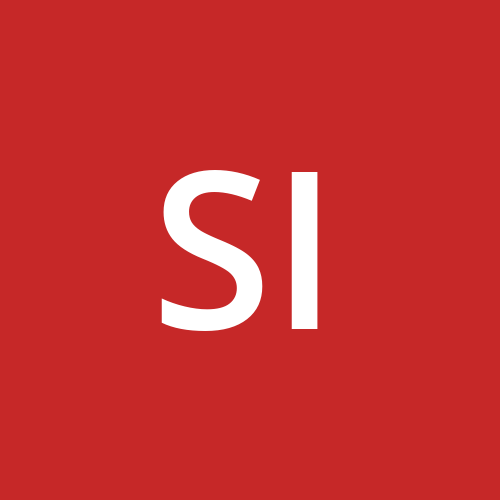