State in React - Declarative vs. Imperative Programming

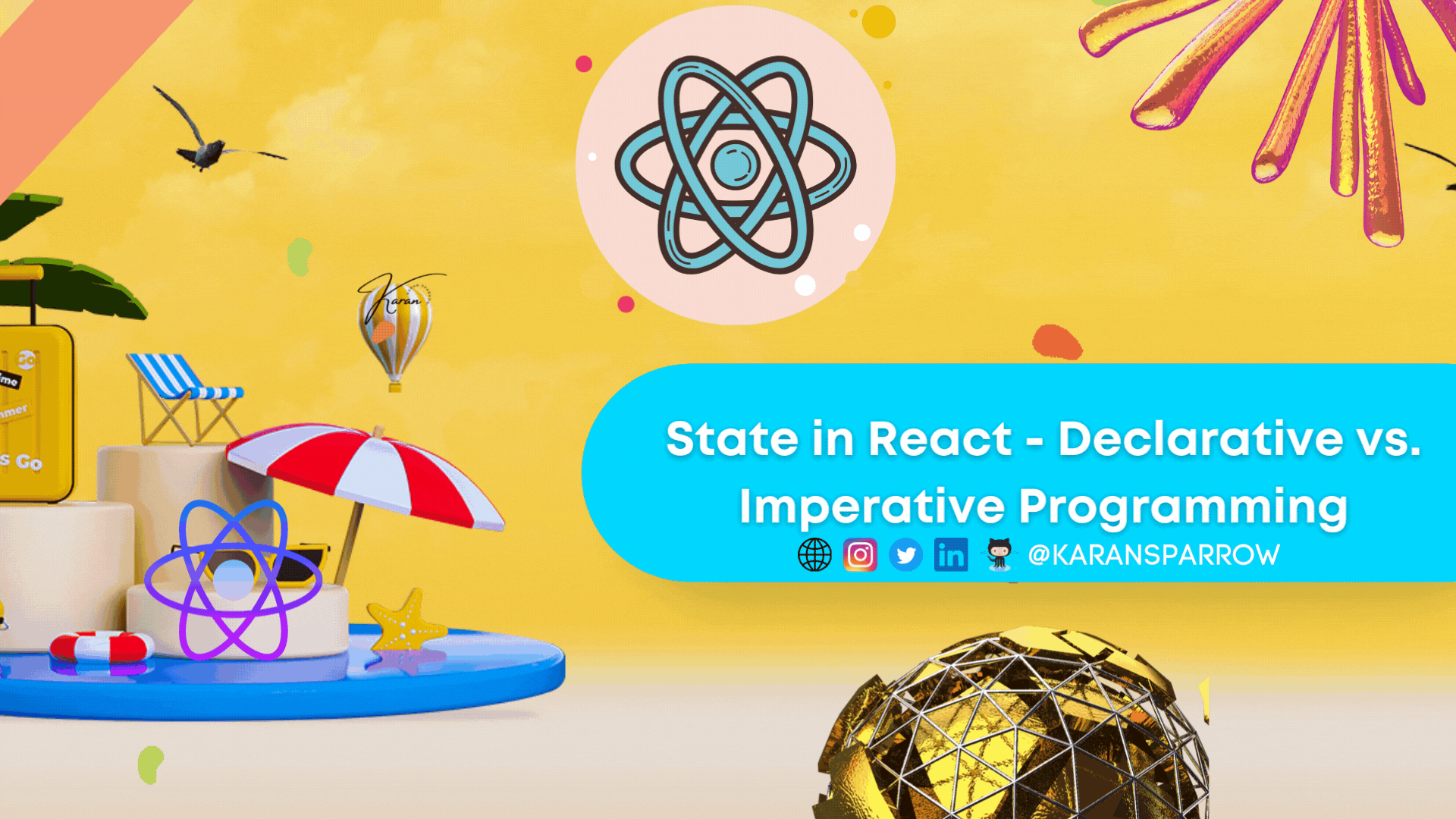
How can we make our apps more interactive? To do that we must first understand the concept of state. And this is a fundamental concept in how React works.
This type of equation best summarises it. So the idea is that the UI or user interface that someone visiting your website sees is determined by the state of your app. Allow me to explain this further with an analogy.
Consider the difference between ice and water. Aren't they basically the same thing? You could think of them as the same React component, but their appearance will change depending on the temperature. As the temperature rises, ice melts into water. When the temperature drops, the water condenses back into ice.
In this case, you could consider the ice to be the user interface. And, depending on the value of that state, if the temperature is minus 10 degrees in both Fahrenheit and Celsius, you'd see ice.
But if we change the state and the temperature to, say, 60 degrees, our ice will turn into water. As a result, changes in the user interface reflect changes in the state. How is this accomplished in code? We would most likely keep track of a variable, say temperature, and define how our user interface would change more frequently depending on the different values of that variable.
import React from "react";
function App() {
return <p> Hello</p>;
}
export default App;
Let's look at how we might do this in code. Here's a simple app component that contains only a single paragraph element and displays the word 'Hello.' So, if we have a variable called strike and set it to false, we can use it to change the appearance of our React component.
import React from "react";
var strike = false;
function App() {
return <p> style = {{textDecoration: "line-through"}} Hello</p>;
}
export default App;
For example, within this element, I can add a style property and set it to equal a new Javascript object with the key text decoration. Then, as the value, it will be line-through. You can see the output in your code environment this is how you would create a line through. Of course, we don't want a line through our text to be present all the time. Instead, when this variable is set to true, we want this specific style to be rendered. Let's extract this Javascript object to make our code a little more understandable.
import React from "react";
var strike = false;
const strikeThrough = {textDecoration: "line-through"}
function App() {
return <p> style = {strike? strikeThrough:null} Hello</p>;
}
export default App;
So let's make a const called strikeThrough and set it to equal this Javascript object, which is the styling. To use that style whenever the variable strike occurs.
It is done by ternary operators. Inside those curly braces, all we have to do is check the value of strike. So we'll start with a question mark, and if it's true, we'll use strikethrough styling. However, if it is not true, we will use null. So now, when our strike property, which is the state we're monitoring, is false,
I get the normal text, but when that changes to true, the strike-through styling is applied and my user interface updates to reflect the state change. You can, of course, do it in another way.
import React from "react";
var strike = false;
const strikeThrough = {textDecoration: "line-through"}
function App() {
return <p> style = {strike && strikeThrough} Hello</p>;
}
export default App;
It does exactly the same thing.
The important thing to remember here is that we have a user interface that is determined by the value of a state variable. Declarative programming is another term for this type of programming. When we write code, we declare how our user interface should look under different conditions based on the state.
The other style of programming would be called imperative programming.
This is exactly what we've been doing with Javascript. When we say document.getElementById and then we tap into its properties and set it equal to something, this is us telling this element to do something different early on. And you can achieve the same outcomes.
let us see this in code, Get the element by id from the document in the DOM. Get the entire root div and set its style, specifically the text-decoration property, to line-through.
import React from "react";
import ReactDom from "react-dom";
import App from "./App";
ReactDOM.render(<App />. document.getElementById("root"));
document.getElementById("root").style.textDecoration = "line-through";
And the same thing happens when this line of code is executed.
This is absolutely necessary. Each time we want this change to occur, we grab an item and set its property to a new value. Instead of having to get the element and update its properties, you can simply change strike to true or strike to false if we return to our previous declarative style of code where we were tracking that variable strike. However, despite the fact that this code should theoretically work, it does not. And the reason for this is that these elements are being rendered and cannot be changed. They must be re-rendered on the screen for changes in their properties, such as their style property, to be visible. To make these complete Hooks are used. Hooks is a very effective one. They are essentially functions that allow us to hook into our app's state and read or modify it.
Thank you for reading!
Check out my profiles on these platforms.
Subscribe to my newsletter
Read articles from Karan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Karan
Karan
Hey, I'm Karan! A passionate Fullstack developer from India