Explainer For The Rock-Paper-Scissors Game With Python (1)

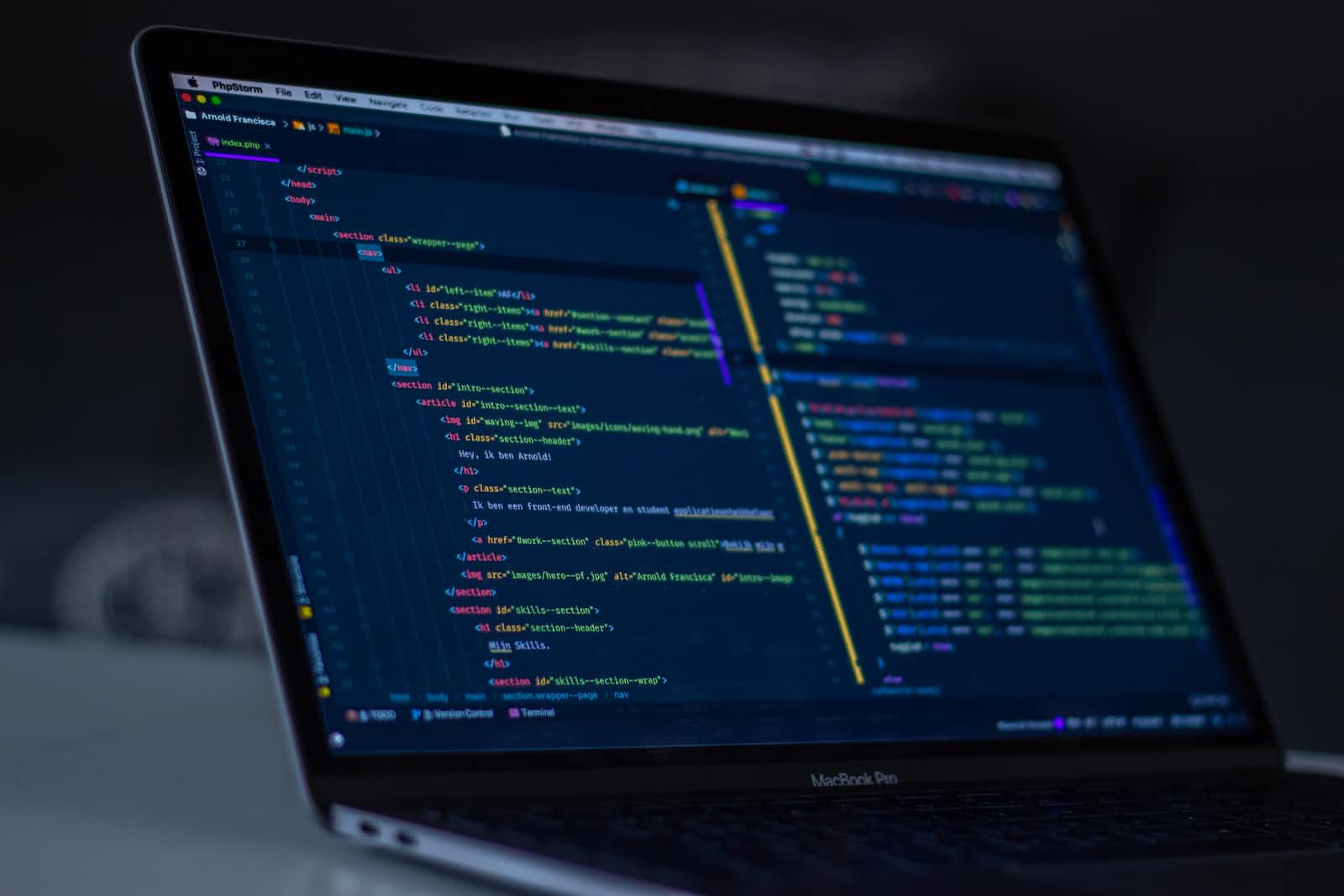
import random
def the_game():
player_choice = input("Enter a choice (rock, paper, scissors): ")
options = ["rock", "paper", "scissors"]
computer_choice = random.choice(options)
response = {"player": player_choice, "computer": computer_choice}
return response
First, I will like to point out that I am using the Replit code editor to run my codes because I actually think it’s cool.
Now, following the image we will go through the code blocks, one at a time:
import random
Why import random? Well, since we would want the computer to randomly generate a choice or option, we need to import the random as outlined here :
https://docs.python.org/3/library/random.html
This is more like linking your CSS, Bootstrap, or JS to your index page.
def the_game():
player_choice = input("Enter a choice (rock, paper, scissors): ")
options = ["rock", "paper", "scissors"]
computer_choice = random.choice(options)
response = {"player": player_choice, "computer": computer_choice}
return response
We declare a function in Python with the def keyword and the colon (:) serves as braces to contain the actions to be executed when the function is called.
Here, we created a function named the_game, and in it are a few variables:
player_choice which is assigned to an action that will be displayed for the player to input an option.
options is a variable assigned to an array of options to select from, in this case, rock, paper, or scissors.
computer_choice is a variable assigned to one of the random operations where the computer will randomly choose from the items in the random variable.
response is a variable we created that contains objects and their values, in this case, we said the word player should have player_choice variable as its value while the word computer should have the variable computer_choice as its value.
Now, we can return response when the function is called.
— — — — — — — — — — — — — — — — — — — — — — — — — — — — -
Follow up on the next post for the conclusion.
Subscribe to my newsletter
Read articles from Victory Ukachukwu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
