Explainer For The Rock-Paper-Scissors Game With Python (2)

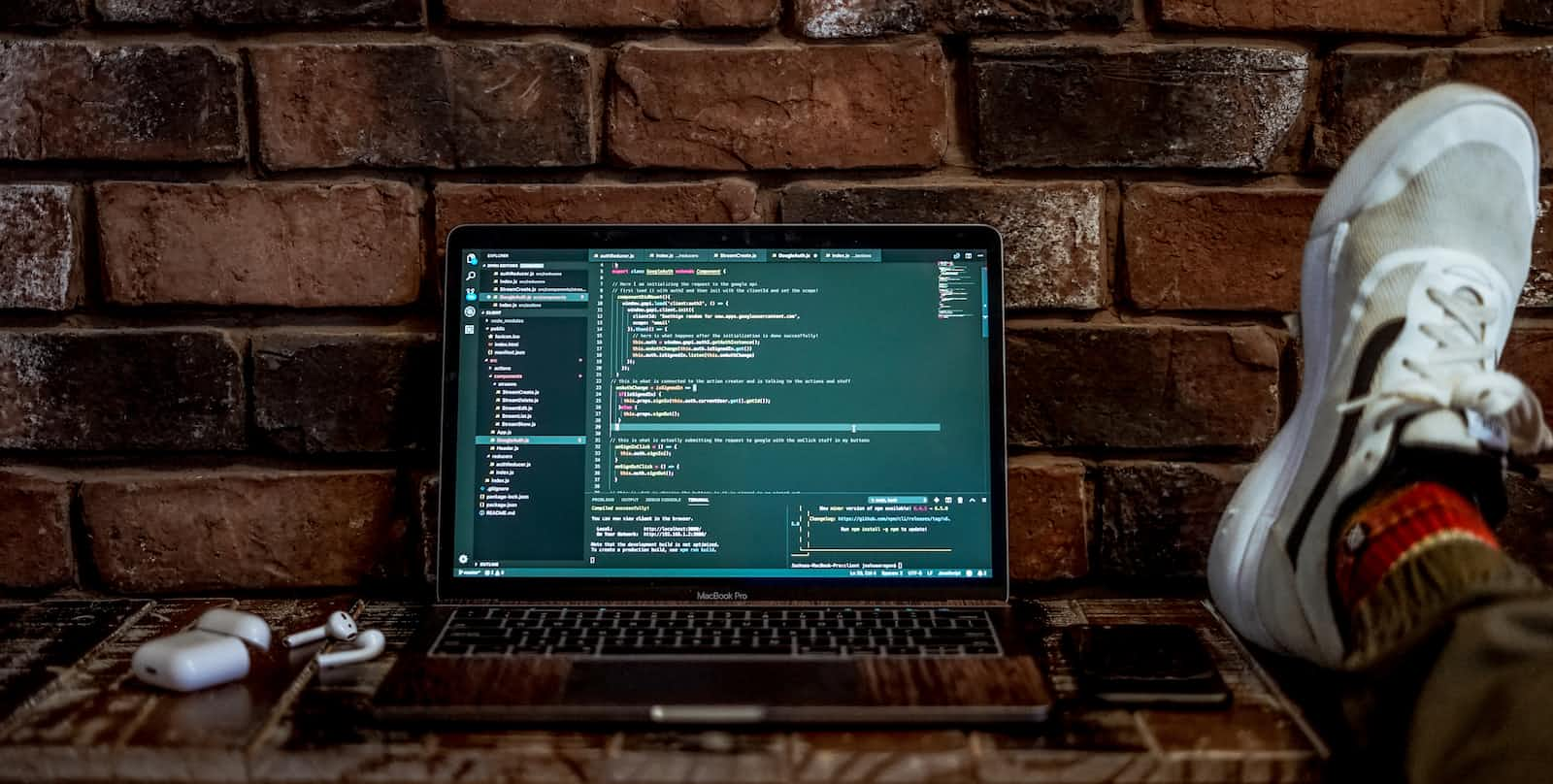
import random
def the_game():
player_choice = input("Enter a choice (rock, paper, scissors): ")
options = ["rock", "paper", "scissors"]
computer_choice = random.choice(options)
response = {"player": player_choice, "computer": computer_choice}
return response
def check_win(player, computer):
print(f"You chose {player}, computer chose {computer}")
if player == computer:
return "A tie!"
elif player == "rock":
if computer == "scissors":
return "Rock smashes scissors! You win!"
else:
return "Paper covers rock. You lose."
elif player == "paper":
if computer == "paper":
return "Paper covers rock. You win!"
else:
return "Scissors cuts paper. You lose."
elif player == "scissors":
if computer == "paper":
return "Scissors cuts paper. You win!"
else:
return "Rock smashes scissors! You lose."
response = the_game()
score = check_win(response["player"], response["computer"])
print(score)
Welcome back! In the last post, we were able to discuss the first line of code with the code block following it...which is the first function def the_game().
Let's look the next code block which is the second function def check_win(player, computer):
def check_win(player, computer):
print(f"You chose {player}, computer chose {computer}")
if player == computer:
return "A tie!"
elif player == "rock":
if computer == "scissors":
return "Rock smashes scissors! You win!"
else:
return "Paper covers rock. You lose."
elif player == "paper":
if computer == "paper":
return "Paper covers rock. You win!"
else:
return "Scissors cuts paper. You lose."
elif player == "scissors":
if computer == "paper":
return "Scissors cuts paper. You win!"
else:
return "Rock smashes scissors! You lose."
This function is used to check the winner of the game and we inputed player and computer as the parameters to check their individual inputs and get a winner.
Remember, in the last post, we used these two parameters as variables and assigned them to values:
response = {"player": player_choice, "computer": computer_choice}
So, when you see the variable player just know it represents player_choice variable + value and when you see the variable computer, know it represents computer_code variable + value. Let's move on...
The next line has the print keyword and a statement we would want displayed when the player and computer have both selected an item from the options variable.
print(f"You chose {player}, computer chose {computer}")
The f included in the parenthesis is the easiest way to concatenate strings and variables without using the "+" sign, just begin and end the statement with inverted commas, then wrap the variables in curly braces. Easy, right?
Following what we have understood earlier, you can guess what the statement simply means...
You chose {any of the option you typed into the input displayed}, computer chose {any random choice from the items contained in the options variable}.
Now, how do we know who wins and who doesn't? That's where the if conditional statement comes in...more like:
"if you don't stop eating lots of junk food, you'll be obessed;
else if (in this case, elif) you visit the gym often".
if player == computer:
return "A tie!"
elif player == "rock":
if computer == "scissors":
return "Rock smashes scissors! You win!"
else:
return "Paper covers rock. You lose."
So, if the player's choice is the same with what the computer randomly chose, then it's a tie!
The next set contains nested if and else inside the else if (in Python, you just say elif...). This simply means, else if the player chose rock and if the computer randomly chose scissors, or (else) paper in that same scenerio, so and so should be returned.
Got it?
It is the shorter form of this code:
elif player == "rock" and computer == "scissors":
return "Rock smashes scissors! You win!"
elif player == "rock" and computer == "paper":
return "Paper covers rock. You lose."
Eh...you wouldn't want that long process, would you? Well, unless you want to hoard lots of codes to wow your non-techie friends.
----------------------------------------------------------------------
You can figure out the rest of the elifs left, right? Let's discuss just the last part on the next post.
Subscribe to my newsletter
Read articles from Victory Ukachukwu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
