How to integrate MPESA API to node js application
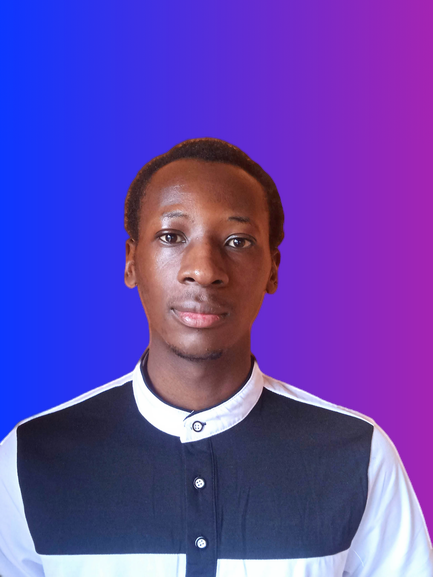
M-Pesa is a popular mobile payment service in Kenya that allows users to transfer money and make payments using their mobile phones. If you have a website and you want to integrate M-Pesa into it, you can use Node.js to create a server-side application that interacts with the M-Pesa API.
Here are the steps you can follow to integrate M-Pesa into your website using Node.js: First, you will need to sign up for a M-Pesa account and obtain the necessary API credentials, including your consumer key and consumer secret. Next, you will need to install the M-Pesa node module, which provides an easy-to-use interface for interacting with the M-Pesa API. You can do this by running the following command:
npm install mpesa
After the module has been installed, you can require it in your Node.js script using the following code:
let mpesa = require('mpesa');
Before you can make any API requests, you will need to initialize the M-Pesa module with your API credentials. You can do this using the following code:
var options = {
consumerKey: 'YOUR_CONSUMER_KEY',
consumerSecret: 'YOUR_CONSUMER_SECRET'
};
mpesa.init(options);
Once the M-Pesa module has been initialized, you can start making API requests. For example, if you want to initiate a payment request, you can use the initiatePayment() method, like this:
mpesa.initiatePayment({
amount: 100, // the amount to be paid, in Kenyan shillings
phoneNumber: '2547XXXXXXXX', // the phone number of the customer
reference: 'Payment for product XYZ' // a reference for the transaction
}, function (error, response) {
// handle the response or error here
});
You can also use the checkTransactionStatus() method to check the status of a transaction using its transaction ID:
mpesa.checkTransactionStatus('TRANSACTION_ID', function (error, response) {
// handle the response or error here
});
By following these steps, you can easily integrate M-Pesa into your website using Node.js and the M-Pesa node module. This will allow your customers to easily make payments using their mobile phones, and you can use the M-Pesa API to manage the transactions and check their status.
Subscribe to my newsletter
Read articles from Allan Juma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
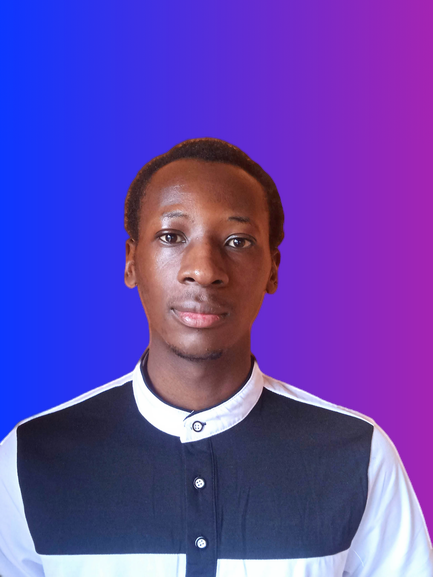
Allan Juma
Allan Juma
Full stack developer and web security enthusiast.