Character, Pointer And String In C Part 1
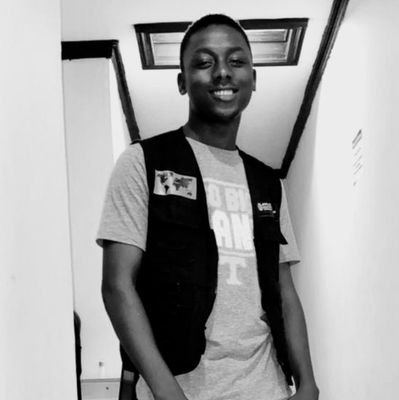
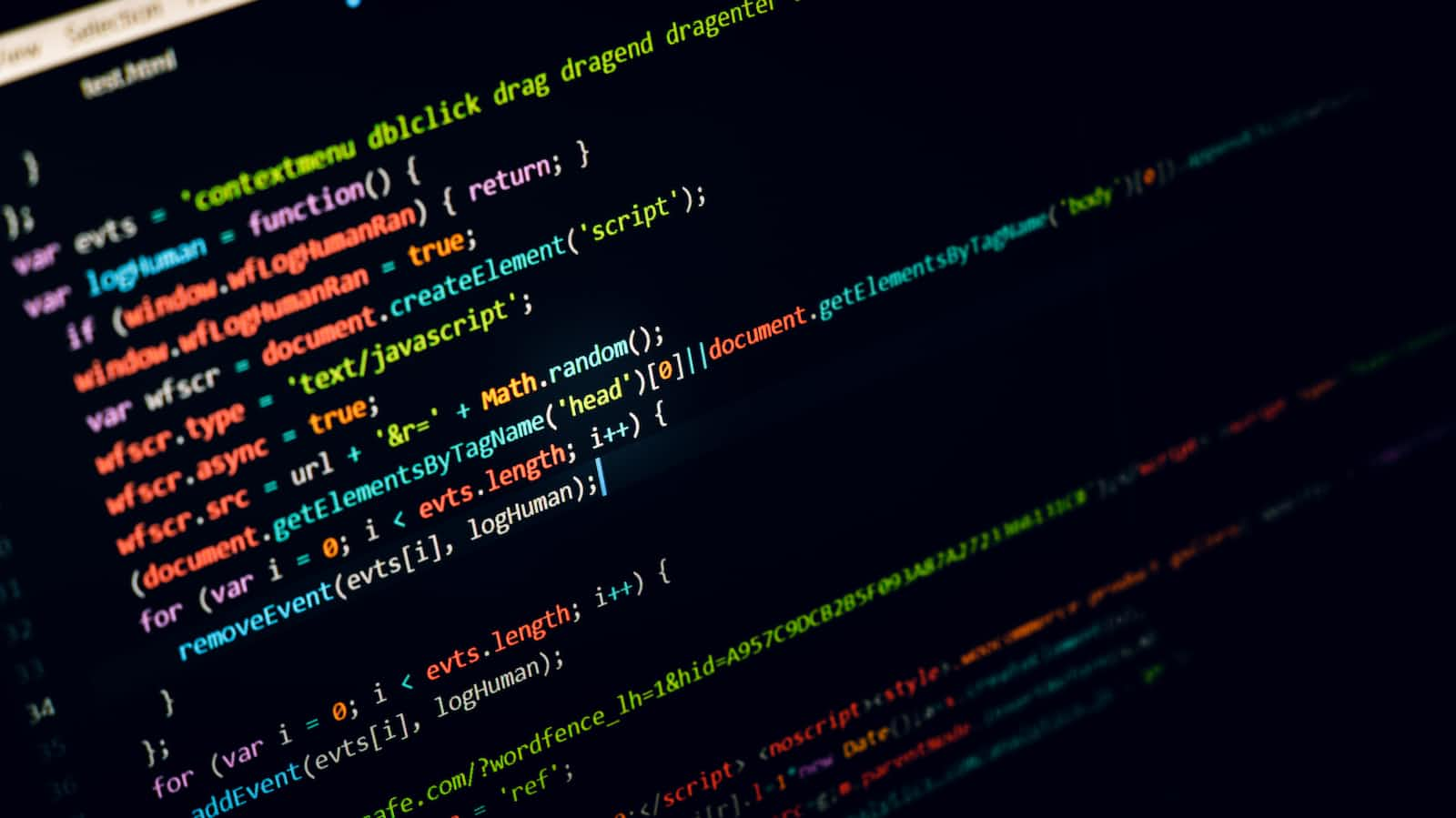
In this series of tutorials we are going to explore the inner working of character, pointer and string in c.
For today's tutorial we will be discussing characters.
Objective:
Understand:
What is a character in C?
How character is represented internally?
How character is printed in C?
Prerequisite
Basic C Knowledge
Willingness To Learn
What is a character in C?
Character in C is a promoted type of integer that can represent any single letter of the alphabet (A-Z a-z), number (0-9), or symbol ($#&%*... ).
But wait what this statement means "character is a promoted type of integer"?
This statement means characters are actually integer treated specially.
How character is represented internally in C?
Internally characters are represented by integer mapped to responding ASCII character.
But wait what is a ASCII Character?
Firstly ASCII means American Standard Code for Information Interchange. It's a character encoding standard for electronic communication between computers.
ASCII assigns standard numeric values(0 - 127) to letters, numerals, punctuation marks, and other characters used in computers, these letters, numerals and symbols are referred to as ASCII Characters. Also the numeric value assigned to each ASCII Character is called ASCII Value.
For Example:
Capital Letters:
65 => 'A' to 90 => 'Z'
Small Letters:
97 => 'a' to 122 => 'z'
Numbers:
48 => '0' to 57 => '9'
ASCII TABLE
More Details On ASCII Character
How character is printed in C?
Character is printed using the standard putchar function of the C language. All function that print value in C used the putchar function or write system call internally.
putchar function prototype:
int putchar(int ch);
Let do some examples
It's important to think of character as ASCII Value (integer in the range of 0 - 127) in C.
Let print a char using its ascii character:
#include <stdio.h>
int main(void)
{
char ch = 'A';
putchar(ch);
return (0);
}
Output
A
Let print a char using its ascii value
#include <stdio.h>
int main(void)
{
char ch = 65;
putchar(ch);
return (0);
}
Output
A
Let print from A-Z using only integer
#include <stdio.h>
int main(void)
{
int ch = 65;
while (ch <= 90)
{
putchar(ch);
ch++;
}
putchar('\n');
return (0);
}
Output
ABCDEFGHIJKLMNOPQRSTUVWXZY
Let write a function that check if a letter is in upper case
#include <stdio.h>
void is_upper(char ch)
{
if (ch >= 65 && ch <= 90)
printf("%c is a capital letter.\n", ch);
else
printf("%c is not a capital letter.\n", ch);
}
int main(void)
{
char ch1 = 'C';
char ch2 = 'c';
is_upper(ch1);
is_upper(ch2);
return (0);
}
Output
C is a capital letter.
c is not a capital letter.
Practice Work
Using The Knowledge Get You From This Tutorial:
Write a program that print from lower case a - z.
Write a program that check if a character is in lower case.
Write a program that convert lower case character to upper case.
Write a program that convert upper case character to lower case.
Note: You can submit your solution in the comment section if you like
Please Don't Forget To Like This Post If You Learn Something New From It.
Please feel free to reach out to me if you want to discuss anything with me or if you identify an error in this tutorial. You can also suggest some places I need to improve on.
Thanks ๐ ๐ For Your Time! See You In Part 2!
Subscribe to my newsletter
Read articles from Maxwell Dorliea directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
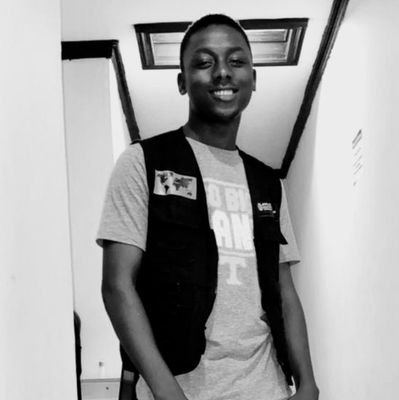
Maxwell Dorliea
Maxwell Dorliea
I am a Full Stack Developer and Writer who loves working on projects that solve real problems, collaborating with like minded developers and follows emerging technologies.