ServiceNow: Check for duplicates in an array
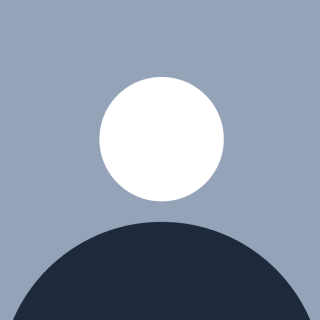
2 min read
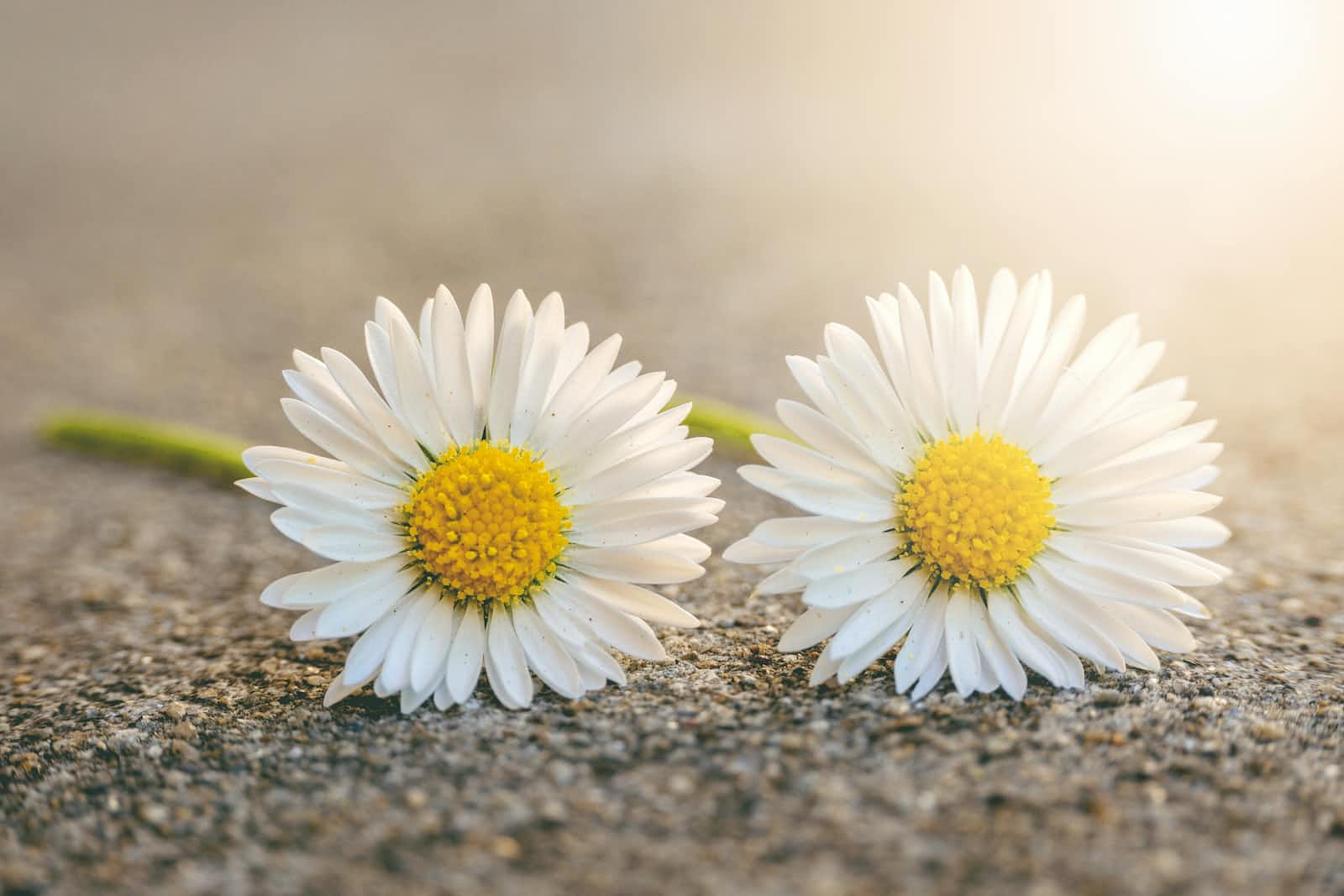
Use cases
Check if an array contains duplicate values
Options / Tools to achieve this
every()
some()
filter()
more will be added soon
How To - Details:
1. Use every() with indexOf()
/*
** @param (string) element - current element that is being processed in the array.
** @param (number) index - index of the current element being processed in the array.
** @param {array} arrValues - array of values
** return {Boolean} true if values are unique, false if it contains duplicates
*/
// Use Case 1: currently logged in user
var arrValues = ['test1', 'test2', 'test1'];
function isUnique(arrValues) {
return isUniqueValues = arrValues.every(function (element, index, arrValues) {
// Check if there is any duplicate value
return arrValues.indexOf(element) === index;
});
}
isUnique(arrValues); // returns false in this case
2. Use some() with indexOf()
/*
** @description: Check if the array contains duplicates (if it finds more than one index for each value / element)
** @param (string) element - current element that is being processed in the array.
** @param {array} arrValues - array of values
** @returns {boolean} - true if it contains duplicates
*/
var arrValues = ['test1', 'test3', 'test1'];
function containsDuplicates(arrValues) {
var result = arrValues.some(function (element) {
return arrValues.indexOf(element) !== arrValues.lastIndexOf(element);
});
return result;
}
containsDuplicates(arrValues);
3. Use filter() with indexOf()
var arrValues = ['test1', 'test3', 'test1'];
function fetchDuplicates(arrValues ) {
return arrValues.filter(function(item, index) {
return arrValues.indexOf(item) !== index});
}
var hasDuplicates = fetchDuplicates(arrValues ).length > 0 ? true : false;
Test this in a Background Script or in Xplore:
Of course you can also return the value that has a duplicate / is not unique:
Useful links
1
Subscribe to my newsletter
Read articles from GROW2DEV directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by