Coding clean in react js
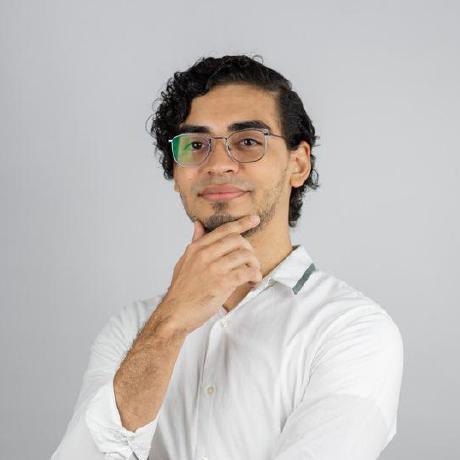
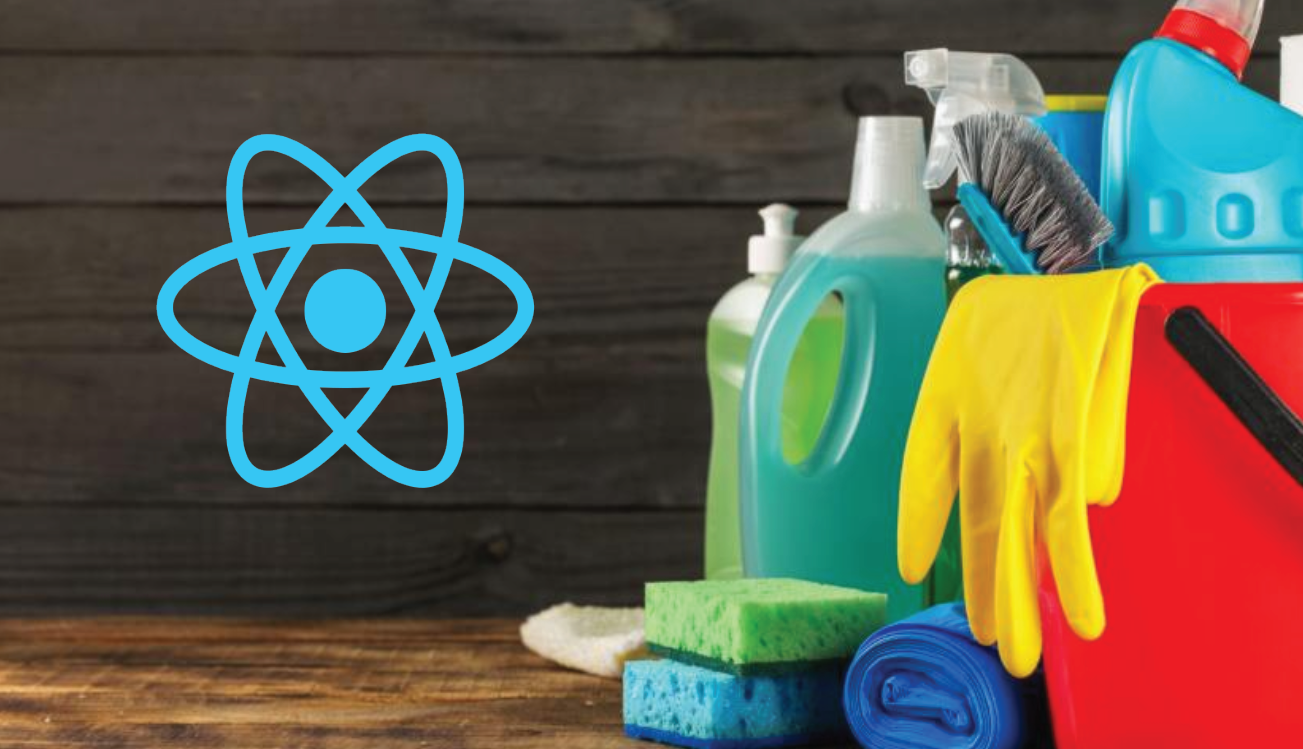
Introduction
Clean code is important in any programming language, but it is especially important in React due to the nature of the framework and the importance of readability in a component-based architecture. In this blog post, we'll discuss some best practices for writing clean code in React.
First and foremost, it's essential to understand what clean code is and why it matters. Clean code is code that is easy to read, understand, and maintain. It follows a consistent style, uses descriptive and meaningful names, and is well-structured. This is critical because code is often read more often than it is written, and having clean code makes it easier for other developers (including yourself) to understand and work with.
Use functional components!
One of the key principles of clean code in React is to use functional components wherever possible. Functional components are simpler and easier to read than class-based components, and they also have the added benefit of being easier to test. If you do need to use a class-based component, make sure to keep the logic as simple and concise as possible.
Examples
Here are some examples of the difference between functional components and class-based components:
Rendering a simple text
Class-based component:
class Greeting extends React.Component {
render() {
return <h1>Hello, {this.props.name}!</h1>;
}
}
Functional component:
import React from 'react';
// This is a functional component that takes in a single prop called "name"
function Greeting(props) {
return <h1>Hello, {props.name}!</h1>;
}
Counter
Class-based:
import React from 'react';
// This is a class-based component that extends the "React.Component" class
class Greeting extends React.Component {
render() {
return <h1>Hello, {this.props.name}!</h1>;
}
}
// This is another class-based component that manages state using a class property
class Counter extends React.Component {
state = {
count: 0,
};
handleClick = () => {
this.setState({
count: this.state.count + 1,
});
}
render() {
return (
<div>
<p>You clicked {this.state.count} times.</p>
<button onClick={this.handleClick}>Click me!</button>
</div>
);
}
}
Functional:
// This is another functional component that uses the "useState" hook to manage state
function Counter() {
const [count, setCount] = React.useState(0);
return (
<div>
<p>You clicked {count} times.</p>
<button onClick={() => setCount(count + 1)}>Click me!</button>
</div>
);
}
Meaningful names
Another important principle is, as the book Clean Code by Robert C. Martin says, to use descriptive and meaningful names for your components, props, and state variables. This will make it easier for other developers (and yourself) to understand what the code is doing, and it will also make it easier to refactor and maintain your code in the future. For example, instead of using a variable named "data" or "items", you could use a more descriptive name like "productList" or "customerRecords".
Example
Render
renderInputField
: This could be a function that renders an input field on the page.
renderErrorMessage
: This could be a function that renders an error message to the user.
Data fetching
fetchUserData
: This could be a function that fetches user data from an API.
fetchBlogPosts
: This could be a function that fetches blog posts from an API.
Event handling
handleFormSubmit
: This could be a function that handles a form submission.
handleDelete
: This could be an event handler function that is called when a user deletes something.
Other
sortDataByDate
: This could be a function that sorts data by date.
calculateTotalPrice
: This could be a function that calculates the total price of items in a shopping cart.
Small components
It's also significant to keep your components small and modular. This will make it easier to understand and maintain your code, and it will also make it easier to reuse your components in different parts of your application. If you find that a particular component is becoming too large or complex, consider breaking it down into smaller, more focused components.
Example
Let's look at the following example:
const UserProfile = ({ user }) => {
return (
<div>
<img src={user.avatarUrl} alt={user.name} />
<div>
<h1>{user.name}</h1>
<p>{user.email}</p>
</div>
<div>
<p>{user.followers} followers</p>
<p>{user.following} following</p>
</div>
</div>
);
};
This component is small enough to understand it quickly, but you still have to dive in to get the idea. You could even modify something by mistake. This could be avoided by separating these into smaller components:
import React from 'react';
const UserProfile = ({ user }) => {
return (
<div>
<UserAvatar user={user} />
<UserInfo user={user} />
<UserStats user={user} />
</div>
);
};
const UserAvatar = ({ user }) => {
return <img src={user.avatarUrl} alt={user.name} />;
};
const UserInfo = ({ user }) => {
return (
<div>
<h1>{user.name}</h1>
<p>{user.email}</p>
</div>
);
};
const UserStats = ({ user }) => {
return (
<div>
<p>{user.followers} followers</p>
<p>{user.following} following</p>
</div>
);
};
export default UserProfile;
The UserProfile component is a parent component that displays a user's avatar, basic info, and follower stats. This parent component is composed of three child components: UserAvatar, UserInfo, and UserStats. These child components are responsible for rendering the avatar image, user name and email, and follower stats, respectively.
The UserProfile component passes the user object as a prop to each of the child components. This allows each child component to access the data it needs to render its part of the user profile.
Separating the UserProfile component into smaller, more focused components like this can make the code easier to read and maintain. It can also make it easier to reuse individual parts of the user profile in other parts of the application.
Keep it consistent!
Finally, make sure to follow a consistent coding style throughout your codebase. This will make it easier for other developers to read and understand your code, and it will also make it easier for you to work with your own code in the future. There are a number of tools and libraries available to help you enforce a consistent coding style, such as ESLint and Prettier.
Wrap-up
In conclusion, writing clean code in React is essential for building scalable and maintainable applications. By following best practices and using tools like ESLint and Prettier, developers can ensure that their code is easy to read, understand, and modify. Additionally, using functional components and descriptive, meaningful names can make code more concise and reusable. Overall, taking the time to create clean code in React can save time and effort in the long run and lead to better-performing applications.
If you have questions, feel free to leave a comment!
Subscribe to my newsletter
Read articles from Daniel Feliz directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
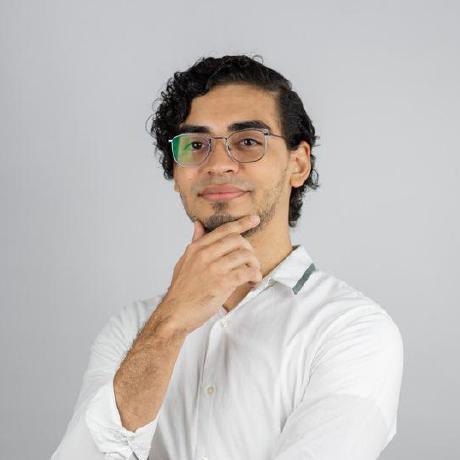