Rock Paper Scissor with Javascript
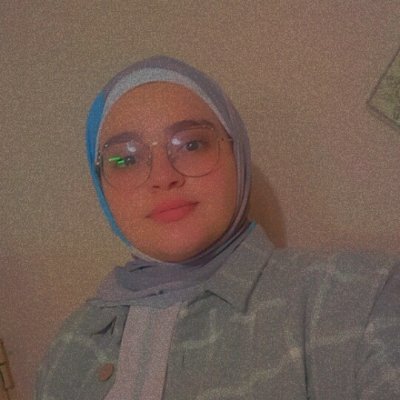
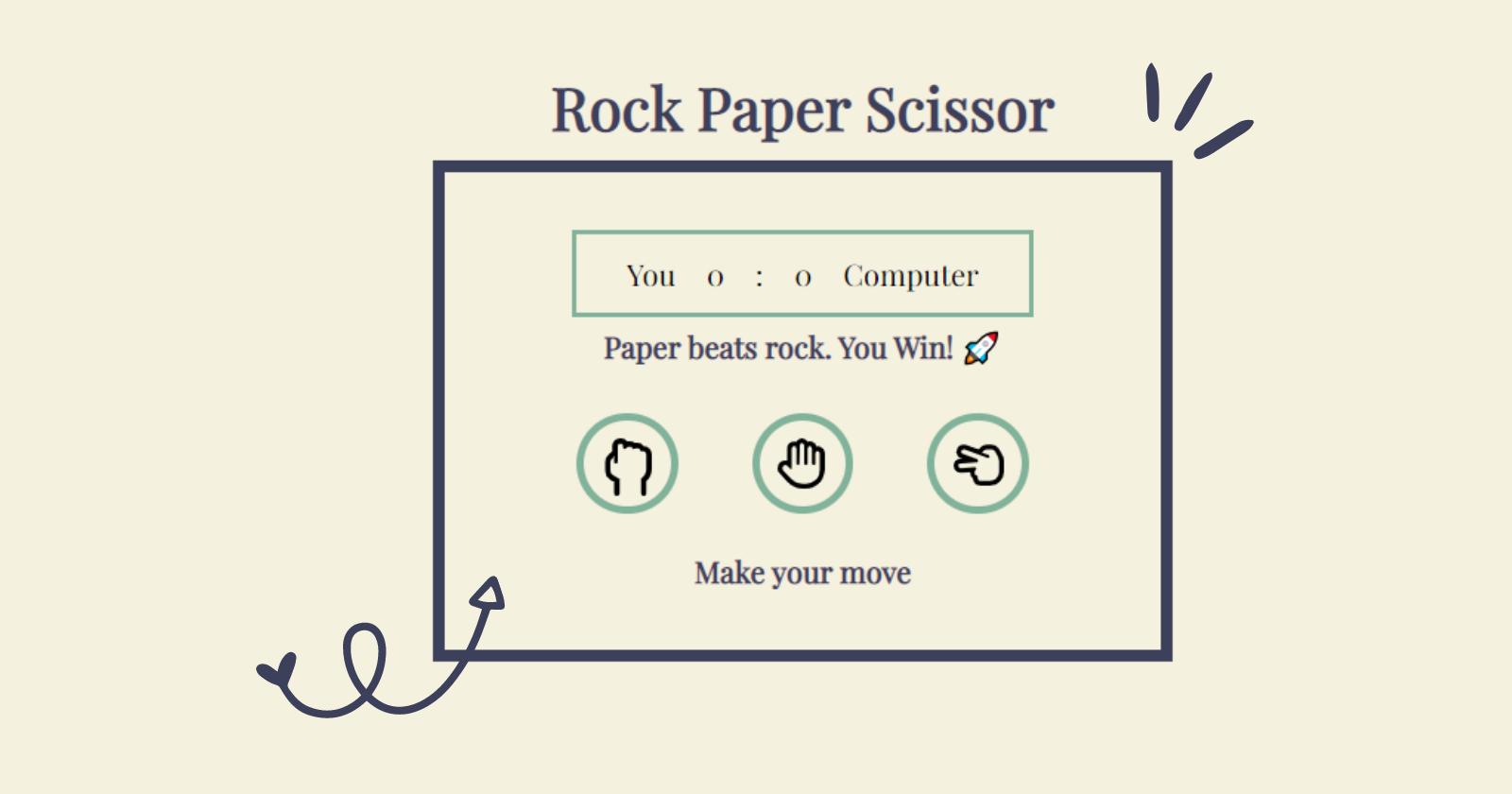
Rock, paper, and scissors game is a simple fun game, It has only two possible outcomes a draw, or a win for one player and a loss for the other player. We will create the game using JavaScript where a player will play against the computer. The player has to choose one option among the rock, paper, and scissors. A random choice will be generated from the computer’s side and the one who wins will get one point every time.
Key concepts covered:
addEventListener()
Math.floor()
Math.random()
Switch Statements
First Thing First
Create a folder
Create an HTML file (index.html)
Create a CSS file (styles.css)
Create a Javascript file (app.js)
Create an image file to include the choices
Set up:
Starting with the skeleton (HTML)
in the HTML file (for me index.html), include the CSS and javascript files.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="styles.css" />
<title>Rock Paper Scissor</title>
</head>
<body>
<script src="app.js"></script>
</body>
</html>
Let's make it pretty (CSS)
Choose the colour palette: I prefer using Coolors
Choose fonts: I prefer using Google Fonts
@import url('https://fonts.googleapis.com/css2?family=Playfair+Display&display=swap');
:root{
--Eggshell: #F4F1DE;
--Independence:#3D405B;
--Green-Sheen: #81B29A;
}
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
body {
width: 100%;
height: 100vh;
font-family: 'Playfair Display', serif;
background-color: var(--Eggshell);
}
Header
HTML
<header>
<h1>Rock Paper Scissor</h1>
</header>
CSS
header{
color: var(--Independence);
text-align: center;
height: 50px;
}
The Layout
Score Board
HTML
<section class="score-board">
<div id="user-label" class="labels">User</div>
<span id="user-score">0</span> : <span id="computer-score">0</span>
<div id="computer-label" class="labels">Computer</div>
</section>
CSS
main{
width: 400px;
border: 7px solid var(--Independence);
padding: 2%;
margin: auto;
}
.score-board{
border:3px solid var(--Green-Sheen);
width: 250px;
margin: auto;
padding: 10px;
display: flex;
justify-content: space-evenly;
}
Result
Rock crushes scissors but is covered by paper
Paper covers rock but is cut by scissors
Scissor is crushed by rock but cut paper.
Html
<section class="result">
<p>Paper beats rock. You Win! 🚀</p>
</section>
CSS
.result{
margin: 5px;
color: var(--Independence);
font-weight: bolder;
text-align: center;
}
Choices
Rock
Paper
Scissor
Html
<section class="choices">
<div class="choice" id="r">
<img src="./images/hand-back-fist-regular.svg" alt="Rock" />
</div>
<div class="choice" id="p">
<img src="./images/hand-regular.svg" alt="Paper" />
</div>
<div class="choice" id="s">
<img src="./images/hand-scissors-regular.svg" alt="Scissor" />
</div>
</section>
CSS
.choices{
display: flex;
justify-content: center;
align-items: center;
}
.choice{
width: 55px;
height: 55px;
border: 4px solid var(--Green-Sheen);
border-radius: 30px;
padding: 10px;
margin: 20px;
}
Message
Html
<p id="msg">Make your move</p>
CSS
#msg{
text-align: center;
color: var(--Independence);
font-weight: bolder;
}
Make it dynamic (Javascript)
Make sure scores start as 0
let userScore = 0;
let computerScore = 0;
Score Board and Result
const userScore_span = document.getElementById("user-score");
const computerScore_span = document.getElementById("computer-score");
const scoreBoard = document.querySelector(".score-board");
const theResult = document.querySelector(".result");
Options
const rock = document.getElementById("r");
const paper = document.getElementById("p");
const scissor = document.getElementById("s");
Computer Choice
function getComputerChoice() {
const choices = ["r", "p", "s"];
const randomNumber = Math.floor(Math.random() * 3);
return choices[randomNumber];
}
Convert to word
function convertToWord(letter) {
if (letter === "r") return "Rock";
if (letter === "p") return "Paper";
return "Scissor";
}
Main Function
function main() {
rock.addEventListener("click", function () {
game("r");
});
paper.addEventListener("click", function () {
game("p");
});
scissor.addEventListener("click", function () {
game("s");
});
}
main();
Game Function
function game(userChoice) {
const computerChoice = getComputerChoice();
switch (userChoice + computerChoice) {
case "rs":
case "pr":
case "sp":
win(userChoice, computerChoice);
break;
case "rp":
case "ps":
case "sr":
lose(userChoice, computerChoice);
break;
case "rr":
case "pp":
case "ss":
draw(userChoice, computerChoice);
break;
}
}
User Wins
function win(userChoice, computerChoice) {
userScore++;
userScore_span.innerHTML = userScore;
computerScore_span.innerHTML = computerScore;
theResult.innerHTML = `${convertToWord(userChoice)}(User) beats
${convertToWord(computerChoice)}(Computer). YOU WIN! 🚀`;
}
User Loses
function lose(userChoice, computerChoice) {
computerScore++;
userScore_span.innerHTML = userScore;
computerScore_span.innerHTML = computerScore;
theResult.innerHTML = `${convertToWord(computerChoice)}(Computer) beats
${convertToWord(userChoice)}(User). YOU LOSE!`;
console.log("you lose");
}
Draw
function draw(userChoice, computerChoice) {
userScore_span.innerHTML = userScore;
computerScore_span.innerHTML = computerScore;
theResult.innerHTML = `${convertToWord(userChoice)}(User) equals
${convertToWord(computerChoice)}(Computer). It's a Draw! 🚀`;
}
Conclusion
To see the completed project: Project
To see the final code: Code
Thank you for your time. I hope you found it useful. ❤️
If you enjoyed this article and want to be the first to know when I post a new one, you can follow me on Twitter @habibawael02 or here at Habiba Wael
Subscribe to my newsletter
Read articles from Habiba Wael directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
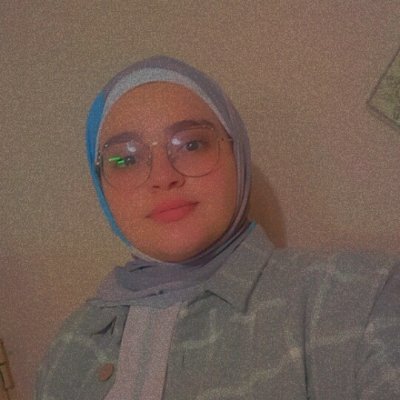
Habiba Wael
Habiba Wael
Hey, I am Habiba. 2⃣ 1⃣ year old full-time learner. 🎓College senior and UX developer intern. 💻 Self-taught web developer. 📚 I love reading, coffee, and discovering new things.