HTTP Requests
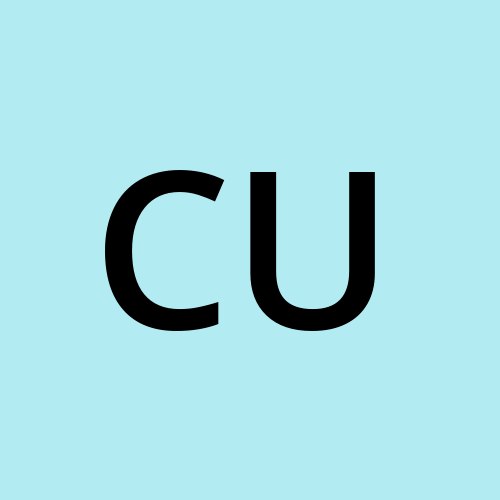
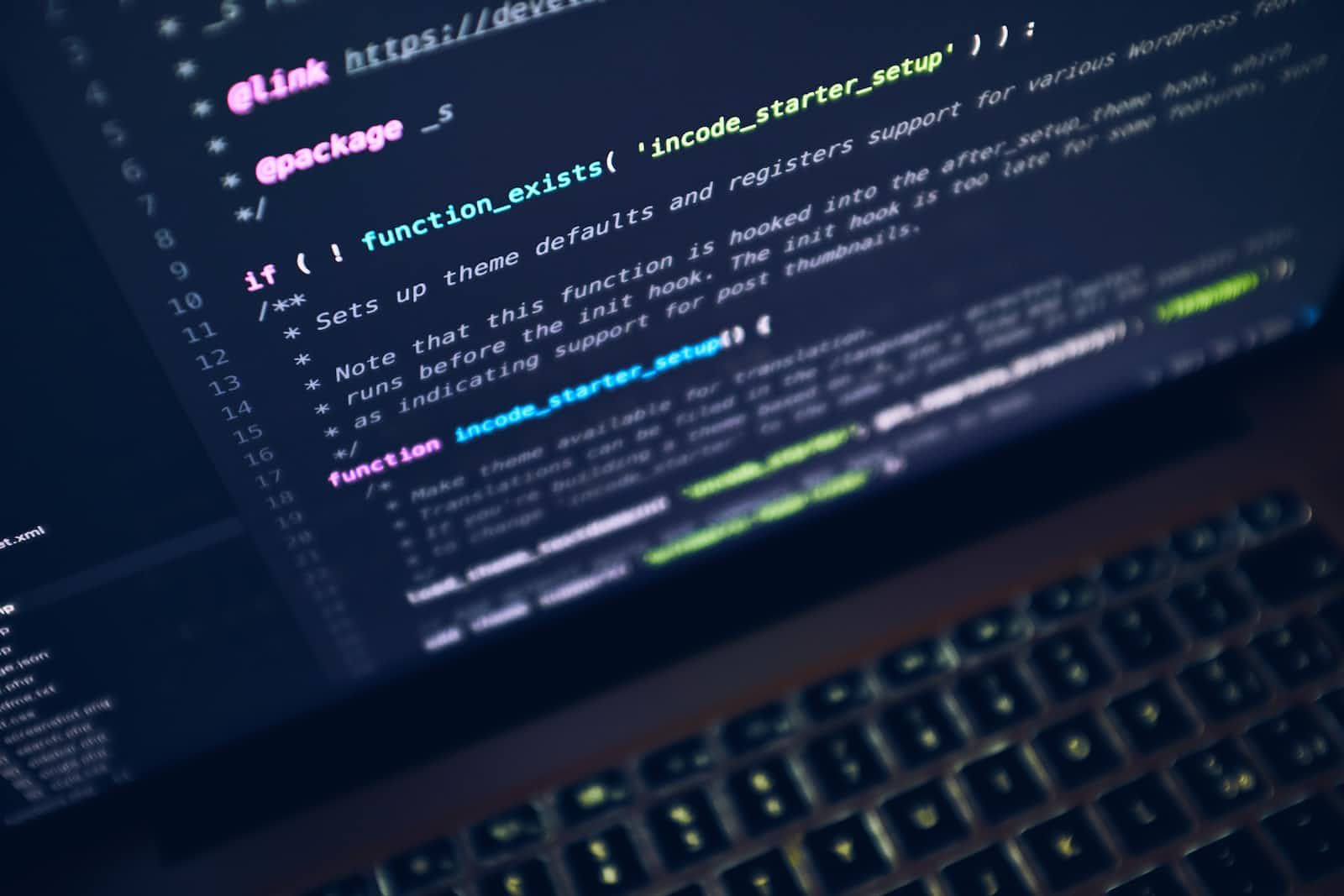
Introduction
The internet is home to a vast amount of resources spread across an equally vast number of servers waiting to be utilized. The client-server model is important to understand how these resources are accessed. The client-server model describes how servers share resources by requiring one program(the client) to make a request for resources from another program(the server) on the same computer or over a network which in turn processes this request and returns a valid response to the client.
When you type in the URL(Universal Resource Locator) of this article into your browser for instance, you are asking it to create a TCP(Transmission Control Protocol) communication channel to the server that houses the resources behind the URL. TCP is a communication standard that ensures the secure transmission of data over a network, it defines how to establish this secure connection as well as maintain it to ensure that the data transmitted is reliable and secure. After this secure connection is established, an HTTP GET request is issued to the server which in turn responds with this article. HTTP which stands for Hypertext Transfer Protocol is a request-response protocol used to structure the requests and responses between a client and a server.
HTTP Request Components
HTTP Request Methods
HTTP Request methods otherwise referred to as HTTP verbs indicate the various actions to be performed for each resource. Some common methods are given below;
GET: This method is used to request a specified resource, it should be used to only request data. An example could be to fetch the user with id 3 or username 'charlie' from the user table given above https://www.example.com/users/3.
HEAD: This method is identical to GET but without the response body.
POST: This method is used to send data to a server, to add a new child resource to the resource collection. An example could be an HTTP POST request to add a newly registered user to the users table above, you could use a POST request with a URL like https://www.example.com/users and a request body containing the data for the new user.
PUT: This method is used to update resources already available on the server with what has been provided in the request body. An example could be to update all fields of the user identified with id 3 on the users table above with new information https://www.example.com/users/3 and a request body containing the updated data for the user.
PATCH: This method is used to apply a partial modification to an existing resource. An example could be to modify the name/email address of the user identified with id 3 on the users table.
DELETE: This is used to delete a specified resource on the server. An example could be to delete the user with id 3 from the users table, you could use a DELETE request with a URL like https://www.example.com/users/3
OPTIONS: This method describes the communication options available for a given server/ resource.
TRACE: This method performs a message loop-back test along the path to the target resource. It is designed for diagnostic purposes.
CONNECT: This method is used to establish a tunnel through a proxy server.
HTTP Request Headers
HTTP requests can include headers, which are additional pieces of information that provide information about the request or the client making the request. These headers can be used to specify the type of data that is included in the request body, the preferred language or encoding of the response, the credentials of the user making the request, and other information.
Here are some examples of common HTTP request headers:
Accept The Accept header is used to specify the acceptable media types for the response. This header can be used to indicate the types of data that the client is able to handle, such as JSON, XML, or plain text. For example, the header Accept: application/json indicates that the client can handle JSON data in the response.
Content-Type The Content-Type header is used to specify the media type of the data included in the request body. This header is used to indicate the format of the data that is being sent to the server, such as JSON, XML, or form data. For example, the header Content-Type: application/json indicates that the request body contains JSON data.
Authorization The Authorization header is used to provide authentication credentials for the user making the request. This header is used to specify the username and password of the user, or a token that identifies the user and grants access to the requested resource. For example, the header Authorization: Basic dXNlcm5hbWU6cGFzc3dvcmQ= includes the base64-encoded username and password of the user.
Cache-Control The Cache-Control header is used to specify the caching behavior of the response. This header can be used to indicate whether the response can be cached, how long it can be cached, and whether it must be revalidated with the server before it is used. For example, the header Cache-Control: max-age=3600 indicates that the response can be cached for up to 1 hour.
These are just a few examples of common HTTP request headers. Many other headers can be used for various purposes, such as to specify the preferred language or encoding of the response, to indicate the type of compression used in the request, or to provide additional information about the client or the request.
HTTP Request Bodies
HTTP requests can include a request body, which is the data that you want to include in the request. This body is typically used with certain HTTP methods, such as POST and PUT, to submit data to the server.
The format of the data in the request body depends on the media type specified in the Content-Type header of the request. Some common formats for the request body include:
Form Data: Form data is a simple format that is commonly used to send data from web forms to the server. Form data is encoded as name-value pairs, with each pair representing a field in the form. For example, the form data name=John&email=john@example.com includes the name and email fields of a form, with the corresponding values "John" and "john@example.com".
JSON: JSON (JavaScript Object Notation) is a popular data interchange format that is used to represent data in a structured and easy-to-read format. JSON data is typically encoded as key-value pairs, where each key represents a property of the data and each value represents the corresponding value of the property. For example, the JSON data {"name": "John", "email": "john@example.com"} includes the name and email properties of an object, with the corresponding values "John" and "john@example.com".
XML: XML (Extensible Markup Language) is a markup language that is used to represent data in a hierarchical and structured format. XML data is encoded using tags and attributes, where each tag represents an element in the data and each attribute represents a property of the element. For example, the XML data Johnjohn@example.com includes the name and email elements of a user, with the corresponding values "John" and "john@example.com".
Making HTTP Requests
How to Make HTTP Requests in Python
To make HTTP requests in Python, you can use the requests module. This module provides a simple interface for sending HTTP requests and receiving responses.
Here is an example of using the requests module to make an HTTP GET request to a specified URL:
import requests
response = requests.get('https://www.example.com')
print(response.status_code)
print(response.headers)
print(response.content)
In this code, the requests.get()
method is used to make an HTTP GET request to the specified URL. The response object returned by this method contains the status code, headers, and response body of the HTTP response. These values can be accessed using the status_code
, headers
, and content
attributes of the response object, respectively.
The requests module also provides methods for making other HTTP request methods, such as requests.post()
for making POST requests, requests.put(
) for making PUT requests, and requests.delete()
for making DELETE requests.
Additionally, the requests module allows you to specify request headers and other parameters by passing them as keyword arguments to the request method. For example, you can set the headers parameter to a dictionary of headers to include in the request, or set the params parameter to a dictionary of query string parameters to include in the URL.
COPY
import requests
response = requests.get( 'https://www.example.com', headers={'Accept-Language': 'en-US'}, params={'key1': 'value1', 'key2': 'value2'}, )
print(response.status_code)
print(response.headers)
print(response.content)
In this code, the headers and params parameters are set when making the request, adding an Accept-Language header and query string parameters to the request.
How to Make HTTP Requests in Javascript
How to Make HTTP Requests in JavaScript To make HTTP requests in JavaScript, you can use the fetch()
function, which is a modern and powerful API for performing network requests. The fetch()
function returns a promise that resolves to a Response object, which contains the response data and metadata from the server.
Here is an example of how to use the fetch() function to make a GET request to retrieve a user from a server:
COPY
fetch('https://www.example.com/users/123')
.then(response => response.json())
.then(user => console.log(user))
.catch(error => console.error(error))
In this example, we are making a GET request to the URL https://www.example.com/users/123 to retrieve a user with the ID 123. The fetch() function returns a promise that resolves to a Response object, which we then use the json()
method to parse the response body as JSON data.
Once the response data has been parsed, we can access the properties of the user object, such as the name and email properties, and use them in our application. If there is an error in the request or the response, the catch() method is called to handle the error.
To make a POST request with a JSON body in JavaScript, you can use the fetch() function and the Headers and Request objects, like this:
COPY
const headers = new Headers({ 'Content-Type': 'application/json' })
const body = JSON.stringify({ name: 'John', email: 'john@example.com' })
const request = new Request('https://www.example.com/users', { method: 'POST', headers: headers, body: body })
fetch(request)
.then(response => response.json())
.then(user => console.log(user))
.catch(error => console.error(error))
In this example, we are making a POST request to the URL https://www.example.com/users to create a new user on the server. The Headers object is used to specify the Content-Type header of the request, indicating that the request body contains JSON data. The Request object is used to specify the method, headers, and body of the request.
We then pass the Request object to the fetch() function to make the request, and handle the response and any errors using the then() and catch() methods, as we did in the previous example.
These are just a few examples of how to make HTTP requests in JavaScript using the fetch() function. You can use this API to make any type of HTTP request and to specify any headers, methods, or body formats that you need.
Conclusion
Conclusion In this article, we have discussed HTTP requests and how to make them in different programming languages. We have looked at the different components of an HTTP request, such as the method, URL, headers, and body, and how these components are used to specify the desired action and data for the request.
We have also looked at some examples of how to make HTTP requests in Python, and JavaScript, using different request methods, headers, and body formats. By understanding the structure and purpose of HTTP requests, you can use them effectively to access and modify data on a server.
HTTP is a powerful and widely-used protocol that forms the foundation of the modern web. By learning how to make HTTP requests, you can build applications that can communicate with web servers and access the vast amounts of information and resources available on the internet.
Thank you for reading this article. I hope it has helped explain the concept of HTTP requests and how to make them in some programming languages. Please feel free to ask any questions or provide feedback in the comments section.
Subscribe to my newsletter
Read articles from CHARLES UDO directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
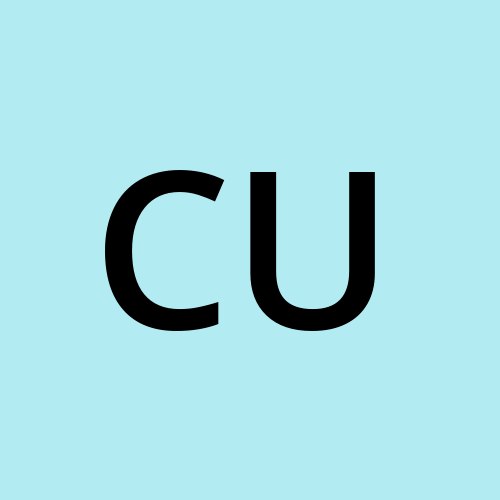
CHARLES UDO
CHARLES UDO
I am a software engineer from Nigeria looking to share and also absorb knowledge.