Understanding Interfaces in Solidity and Blockchain Smartcontracts

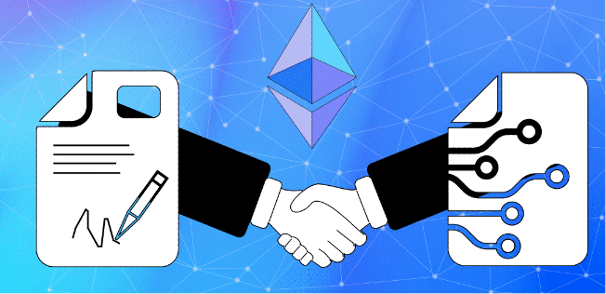
If you are new to building in the web3 space and have begun to write blockchain smart contracts in solidity, especially coming from the traditional web2 background, you would have come across the word "Interfaces".
Like many beginners in web3, the concept of interfaces might have been a little confusing for you. However, by the end of this article, you'd have a thorough understanding of what interfaces are, why they are needed and how they are used in writing EVM smart contracts in solidity
What are Interfaces
In Solidity, interfaces are contracts that define a set of functions that other contracts can implement. Think of it as a keyword in web3, used to expose API endpoints that can be "consumed" from a contract (the interface contract), by other external contracts.
Summarily, Interfaces are used to specify a contract's API, or the functions that it makes available to other contracts.
Importance of Interfaces
Interfaces are useful for a number of reasons. They allow contracts to be written in a modular and reusable way, by allowing contracts to implement the functions defined in an interface without needing to know the details of how those functions are implemented. This makes it easier to write and maintain complex smart contract systems.
Again, if you are coming from a web2 background, think of it as API endpoints(contract functions) that have been exposed by a service (the Interface contract); making it available to be consumed by another service (your smart contract in this case).
Additionally, interfaces can be used to ensure that a contract implements a specific set of functions. For example, a contract that expects to receive calls from another contract can use an interface to specify the exact set of functions that the other contract must implement in order to be compatible with it.
Example of how to implement and use an interface in a solidity smart contract
Let's go through see how you might implement an interface in developing EVM smart contracts:
pragma solidity 0.8.4;
// Define an interface that specifies a set of functions
// that a contract can implement.
interface IMyInterface {
function foo() external;
function bar(uint256 x) external;
}
// Define a contract that implements the IMyInterface interface.
// This contract must implement the foo() and bar(uint256) functions
// defined in the interface.
contract MyContract implements IMyInterface {
// This function implements the foo() function defined in the
// IMyInterface interface.
function foo() external {
// ...
}
// This function implements the bar(uint256) function defined
// in the IMyInterface interface.
function bar(uint256 x) external {
// ...
}
}
Explaining the Example given:
Let me explain the code above step by step to you. Follow closely:
In this example, the `MyContract`
contract implements the MyInterface
interface. This means that it must implement the foo()
and bar(uint256)
functions defined in the interface. This allows other contracts to call the foo()
and bar(uint256)
functions on an instance of MyContract
, knowing that those functions will be available and will have the behavior defined in the interface.
More on interfaces
Interfaces are necessary in Solidity because they allow contracts to be written in a modular and reusable way. By defining an interface, you can specify a set of functions that other contracts can implement, without needing to know the details of how those functions are implemented.
This makes it easier to write and maintain complex smart contract systems. For example, if you have a contract that expects to receive calls from other contracts, you can use an interface to specify the exact set of functions that the other contracts must implement in order to be compatible with it. This ensures that the contracts will be able to interact with each other properly.
Additionally, interfaces can be used to enforce certain standards or conventions in a smart contract system. For example, if you are building a decentralized application (DApp) that uses a particular token, you could define an interface that specifies the functions that a contract must implement in order to be considered a "valid" token contract. This would allow other contracts and DApps to easily interact with any token contract that implements that interface, without needing to know the details of how the token contract is implemented.
Overall, interfaces are an important tool for writing modular and reusable smart contract code, and for ensuring compatibility and interoperability between contracts in a smart contract system
That brings us to the end of this article on interfaces
Hope it helps.
Best Regards. See you in my next article.
Cheers and Happy Coding!
Subscribe to my newsletter
Read articles from clinton felix directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

clinton felix
clinton felix
I am a Blockchain Web3 and Software Engineer and Freelance web3/blockchain Tech content Writer. I have extensive experience building Decentralized web3 applications in DeFi, NFTs and DAOs, on EVM and Non-EVM protocols. I build scalable products and SaaS using GoLang, Rust and MERN stack. I enjoy writing about Tech, perhaps because I have a Strong Growth marketing background. I enjoy the sweet spot tangent of intersection between business and technology.