Basic JAVA interview Questions you MUST know
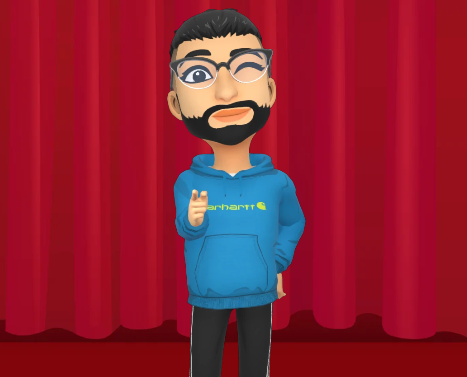
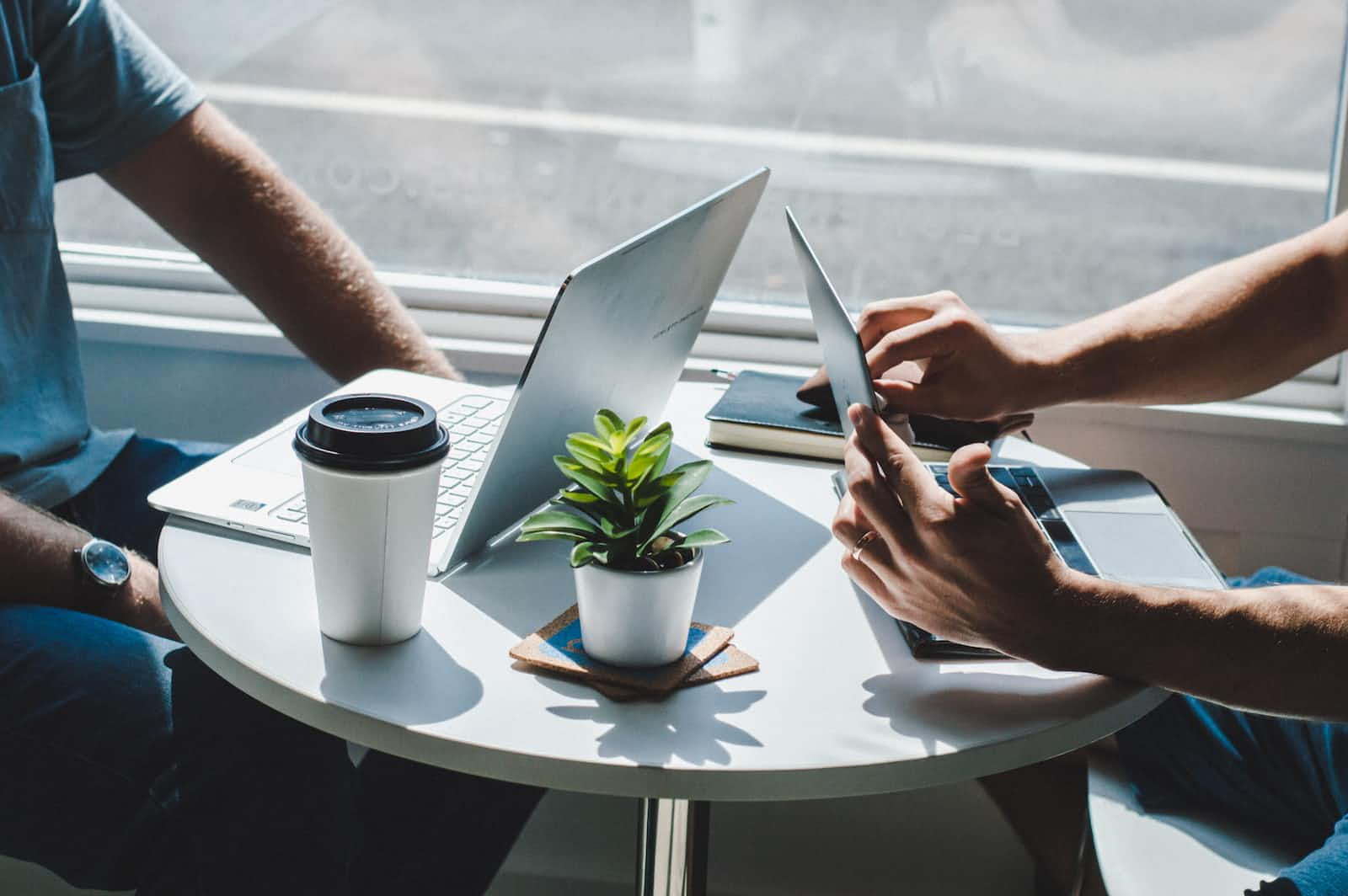
Here are some basic Java interview questions along with their answers:
What is Java?
Java is a high-level, object-oriented programming language developed by Sun Microsystems in the mid-1990s. It is designed to be simple, easy to learn, and easy to write, compile, and debug.
What are the main features of Java?
Some of the main features of Java include:
Object-oriented programming
Platform independence
Simple, easy-to-learn syntax
High performance
Robustness and security
What is the difference between JDK, JRE, and JVM?
JDK (Java Development Kit) is a software development kit that provides tools for developing and running Java applications. It includes the JRE (Java Runtime Environment), as well as compilers and other tools for developing Java code.
JRE (Java Runtime Environment) is a package that contains the necessary tools for running Java programs, including the Java Virtual Machine (JVM) and various libraries.
JVM (Java Virtual Machine) is a virtual machine that executes Java bytecode and provides a runtime environment for Java programs.
What are the main OOP concepts in Java?
The main OOP (Object-Oriented Programming) concepts in Java are:
Encapsulation: The process of bundling data and methods that operate on that data within a single unit (such as a class).
Inheritance: The ability of a class to inherit characteristics and behavior from a parent class.
Polymorphism: The ability of a class to have multiple forms, either through inheritance or through the implementation of multiple interfaces.
What is an object in Java?
An object in Java is a self-contained unit of data and behavior. It is an instance of a class, and it contains data (stored in fields) and methods that operate on that data.
What is a class in Java?
A class in Java is a template or blueprint that defines the characteristics and behavior of an object. It specifies the data that the object will contain (in fields) and the methods that will operate on that data.
What is inheritance in Java and how does it work?
Inheritance in Java is the process by which a class (called the subclass or child class) can inherit characteristics and behavior from a parent class (called the superclass or base class). This allows the subclass to reuse the code and behavior of the superclass, and also to add or modify behavior as needed. Inheritance is implemented using the "extends" keyword.
What is polymorphism in Java and how does it work?
Polymorphism in Java is the ability of a class to have multiple forms, either through inheritance (where a subclass can override or extend the behavior of a superclass) or through the implementation of multiple interfaces. Polymorphism allows a single object to take on multiple forms and to behave differently depending on the context in which it is used.
What is an interface in Java and how does it work?
An interface in Java is a set of abstract methods that define a contract for the behavior of a class. A class that implements an interface must implement all of the methods defined in the interface. Interfaces are useful for specifying the behavior that a class must implement, without specifying the implementation details.
What is a package in Java?
A package in Java is a namespace that organizes a set of related classes and interfaces. Packages provide a way to group related classes and interfaces and prevent naming conflicts between classes with the same name.
To use a class or interface from a package, you must first import it using the "import" statement. You can also use the "*" wildcard to import all of the classes and interfaces in a package.
Packages can also be used to enforce access control, by using the "public" and "private" access modifiers. Classes and interfaces that are marked as "public" can be accessed from anywhere, while those that are marked as "private" can only be accessed within the package.
Subscribe to my newsletter
Read articles from Udit Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
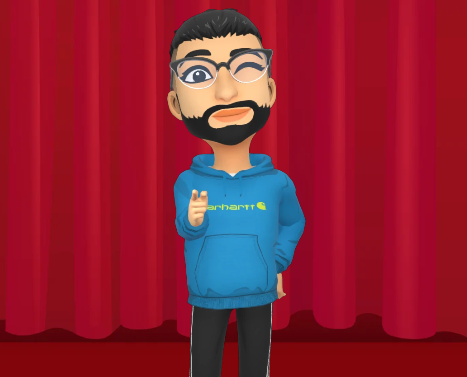