An Introduction to JavaScript: The Programming Language of the Web
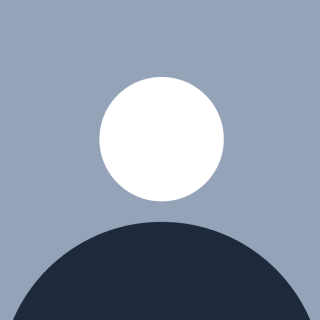
Introduction to JavaScript
JavaScript is a programming language that is commonly used in web development to create interactive and dynamic web pages. It is a client-side language, meaning that it is executed by the web browser rather than the server, allowing for real-time updates and interactions without the need to refresh the page.
History of JavaScript
JavaScript was originally developed in the mid-1990s by Netscape Communications Corporation as a means to add interactivity to their web browser, Netscape Navigator. It was first released in 1995 under the name LiveScript, but it was later renamed to JavaScript in an effort to capitalize on the popularity of Java, a programming language developed by Sun Microsystems.
JavaScript Versions
Since its inception, JavaScript has gone through several versions, each introducing new features and capabilities. The most recent version is ECMAScript 2021, which was released in June 2021. Some of the notable versions of JavaScript include:
ECMAScript 1 (1997): This was the first standardized version of JavaScript, released by the European Computer Manufacturers Association (ECMA).
ECMAScript 3 (1999): This version added regular expressions, improved error handling, and introduced try-catch statements.
ECMAScript 5 (2009): This version introduced new features such as the "strict mode" that enforces stricter syntax and error checking and the JSON object for working with data in JavaScript.
ECMAScript 6 (2015): Also known as ECMAScript 2015, this version introduced a number of new features including classes, arrow functions, and the spread operator.
ECMAScript 2016 (2016): This version introduced the
Array.prototype.includes()
method and theexponentiation operator
.ECMAScript 2017 (2017): This version introduced the
async
andawait
keywords for working with asynchronous code.
How to Run JavaScript
There are several ways to run JavaScript code:
In a web browser: To run JavaScript in a web browser, you can include it in an HTML file and then open the file in a browser. You can also use the browser's developer console to run JavaScript code directly.
Using a command-line interface: You can use a command-line interface such as Node.js to run JavaScript code on the server side.
Using an online code editor: There are many online code editors that allow you to write and run JavaScript code directly in the browser.
Types of JavaScript Applications:
JavaScript can be used to build a variety of applications, including:
Web applications: JavaScript can be used to create interactive and dynamic web applications, such as online shopping carts and social media platforms.
Mobile applications: JavaScript can be used to build cross-platform mobile applications using frameworks such as React Native.
Games: JavaScript can be used to create browser-based games and even mobile games using frameworks like Phaser.
Desktop applications: JavaScript can be used to build desktop applications using frameworks like Electron.
Popular JavaScript Frameworks:
There are many JavaScript frameworks that are popular among developers for building web applications. Some of the most popular frameworks include:
React : A popular library for building user interfaces that is maintained by Facebook.
Angular: A full-featured framework for building web applications.
Vue.js: A progressive framework for building user interfaces.
Node.js: A runtime environment for building server-side applications in JavaScript.
Each of these frameworks has its own unique features and benefits, and choosing the right one can depend on the specific needs of the project.
Debugging JavaScript:
As with any programming language, debugging is an important part of the development process. There are a few common tools and techniques that can be used to debug JavaScript code:
The browser's developer console: Most modern web browsers have a developer console that allows you to view error messages and log output from your code.
Debugging tools: There are many debugging tools available that can help you identify and fix issues in your code. Some popular tools include the Chrome DevTools and the Firefox Developer Edition.
Breakpoints: You can use breakpoints to pause the execution of your code at a specific point and examine the values of variables and the call stack.
Console output: Using
console.log()
and other methods of theconsole
object, you can output messages and data to the developer console for debugging purposes.Security Considerations:
Because JavaScript is a client-side language, it is important to consider security when developing applications. Some best practices for securing JavaScript code include:
Validating user input: It is important to validate user input to prevent injection attacks, such as SQL injection and cross-site scripting (XSS).
Encrypting sensitive data: Any sensitive data, such as passwords or credit card information, should be encrypted to prevent interception.
Protecting against cross-site request forgery (CSRF): CSRF attacks involve tricking a user into making an unintended request to a server. One way to protect against this is by using unique tokens for each user session.
Tips for Learning JavaScript:
If you are new to JavaScript, here are a few tips to help you get started:
Practice, practice, practice: The best way to learn any programming language is to practice writing code. There are many online platforms and resources available for learning and practicing JavaScript.
Start with the basics: It is important to start with the fundamentals of the language, such as variables, data types, loops, and control structures.
Don't be afraid to ask for help: There is a large and active community of JavaScript developers who are willing to help others learn and improve. Don't be afraid to ask for help or clarification if you are stuck on something.
Keep learning: JavaScript is a constantly evolving
Overall, learning JavaScript can open up a wide range of career opportunities and allow you to create interactive and dynamic web applications.
Subscribe to my newsletter
Read articles from Sohan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by