Copying text to the clipboard in JavaScript
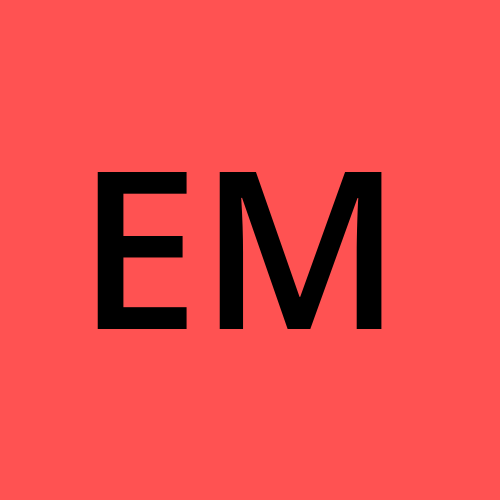
Copying text to the clipboard is a common task in web development. It can be useful for allowing users to quickly copy a URL or some other piece of text from a web page.
In this blog post, we will look at how to copy text to the clipboard in JavaScript in a way that works in all modern browsers and operating systems.
Copying text to the clipboard in modern browsers
In modern browsers, you can use the navigator.clipboard
API to copy text to the clipboard. This API is supported by most modern browsers, including Chrome, Firefox, Edge, and Safari.
Here is a function that uses the navigator.clipboard
API to copy text to the clipboard:
function copyToClipboard(text) {
navigator.clipboard.writeText(text).then(
function() {
/* clipboard successfully set */
},
function(err) {
console.error('Could not copy text: ', err);
}
);
}
To use this function, simply call it and pass in the text that you want to copy as an argument:
copyToClipboard('Hello, world!');
The writeText
method returns a promise, which resolves if the clipboard was successfully set and rejects if there was an error. You can use the then
method to handle the success and error cases, as shown in the example above.
Copying text to the clipboard in older browsers
Unfortunately, the navigator.clipboard
API is not supported by all browsers. If you need to support older browsers, you can use a fallback solution that uses a temporary input
element.
Here is a function that uses this fallback solution:
function copyToClipboard(text) {
const tempInput = document.createElement('input');
tempInput.style.position = 'fixed';
tempInput.style.opacity = 0;
tempInput.value = text;
document.body.appendChild(tempInput);
tempInput.select();
document.execCommand('copy');
document.body.removeChild(tempInput);
}
This function creates a temporary input
element, sets its value to the text that you want to copy, selects the text, and then uses the execCommand
method with the 'copy'
command to copy the text to the clipboard. The input
element is then removed from the DOM.
To use this function, simply call it and pass in the text that you want to copy as an argument:
copyToClipboard('Hello, world!');
Combining the two approaches
To support both modern browsers and older browsers, you can combine the two approaches into a single function. Here is an example of how to do this:
function copyToClipboard(text) {
if (navigator.clipboard) {
// supported by modern browsers
navigator.clipboard.writeText(text).then(
function() {
/* clipboard successfully set */
},
function(err) {
console.error('Could not copy text: ', err);
}
);
} else {
// not supported by modern browsers, use fallback
const tempInput = document.createElement('input');
tempInput.style.position = 'fixed';
tempInput.style.opacity = 0;
tempInput.value = text;
document.body.appendChild(tempInput);
tempInput.select();
document.execCommand('copy');
document.body.removeChild(tempInput);
}
}
This function first checks if the navigator.clipboard
API is available. If it is, it uses the writeText
method to write the text to the clipboard. If the navigator.clipboard
API is not available, it falls back to the method that uses a temporary input
element.
To use this function, simply call it and pass in the text that you want to copy as an argument:
copyToClipboard('Hello, world!');
Copying text to the clipboard on iOS
One important thing to note is that the navigator.clipboard
API is not supported on iOS devices. However, you can use the Selection
API to copy text to the clipboard on iOS.
Here is an updated version of the function that uses the Selection
API to support copying to the clipboard on iOS devices:
function copyToClipboard(text) {
if (navigator.clipboard) {
// supported by modern browsers
navigator.clipboard.writeText(text).then(
function() {
/* clipboard successfully set */
},
function(err) {
console.error('Could not copy text: ', err);
}
);
} else {
// not supported by modern browsers, use fallback
const tempInput = document.createElement('input');
tempInput.style.position = 'fixed';
tempInput.style.opacity = 0;
tempInput.value = text;
document.body.appendChild(tempInput);
tempInput.select();
tempInput.setSelectionRange(0, text.length);
document.execCommand('copy');
document.body.removeChild(tempInput);
}
}
This function works in the same way as the previous version, except that it adds a call to setSelectionRange
on the input
element to select the entire text. This is necessary because the execCommand
method with the 'copy'
command does not work on iOS unless the text is selected.
I hope this blog post has been helpful in showing you how to copy text to the clipboard in JavaScript in a way that works in all modern browsers and operating systems. Let me know if you have any questions or need further clarification on any of the concepts discussed.
That's it.
Subscribe to my newsletter
Read articles from Eirik Madland directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
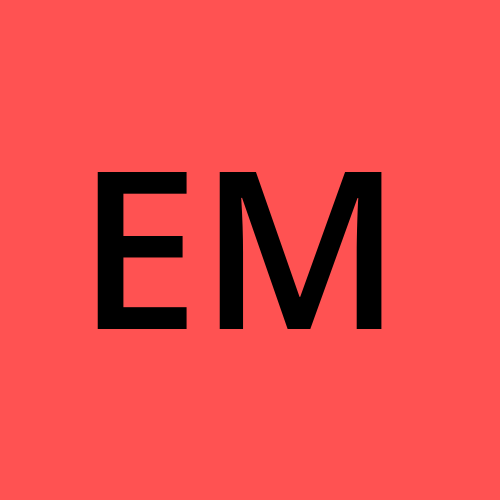