Recursive function vs while loop in javascript

Table of contents
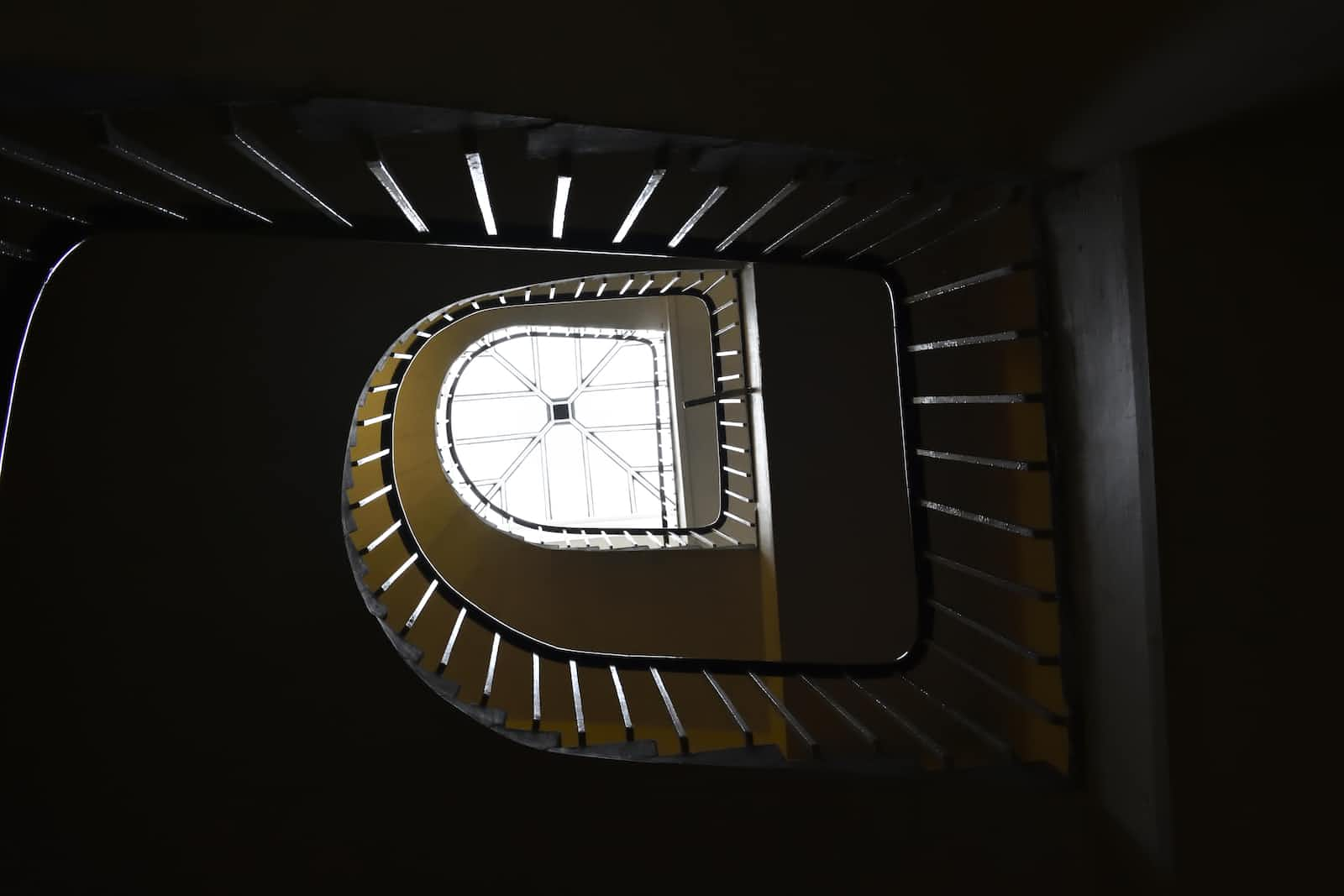
Recursive functions and while loops are common methods for iterating through JavaScript processes. Still, it's important to understand their differences to choose the best approach for a particular situation.
Recursive functions call themselves repeatedly until a certain condition is met. They are often used when a problem can be divided into smaller subproblems that can be solved independently. Here's an example of a recursive function that calculates the factorial of a number:
function factorial(n) {
if (n === 0) {
return 1;
} else {
return n * factorial(n - 1);
}
}
In this example, the function calls itself with a value of n - 1 until n equals 0, at which point it returns 1. Recursive functions can be easier to read and understand but can also be more computationally expensive due to the additional function calls.
While loops, on the other hand, are control flow statements that allow a block of code to be executed repeatedly until a certain condition is met. Here's an example of a while loop that calculates the factorial of a number:
function factorial(n) {
let result = 1;
while (n > 0) {
result *= n;
n--;
}
return result;
}
In this example, the loop continues to execute as long as n is greater than 0, and then it returns the result. While loops are typically more efficient than recursive functions, they can be more difficult to understand if the loop condition and increment are not clearly defined.
So, which method is better to use? It depends on the situation. Recursive functions are best suited for problems that involve dividing problems into smaller subproblems, such as calculating the Fibonacci sequence or traversing a tree structure. Here's an example of a recursive function that calculates the Fibonacci sequence:
function fibonacci(n) {
if (n <= 1) {
return n;
} else {
return fibonacci(n - 1) + fibonacci(n - 2);
}
}
While loops, on the other hand, are better for straightforward iterations, such as iterating through an array and performing a task on each element. Here's an example of a while loop that iterates through an array and prints each element to the console:
let arr = [1, 2, 3, 4, 5];
let i = 0;
while (i < arr.length) {
console.log(arr[i]);
i++;
}
In conclusion, both recursive functions and while loops are useful tools in JavaScript, and the choice between them depends on the specific problem you are trying to solve. By understanding the strengths and weaknesses of each method, you can choose the one that best fits your needs.
Subscribe to my newsletter
Read articles from Engr. Animashaun Fisayo Michael directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Engr. Animashaun Fisayo Michael
Engr. Animashaun Fisayo Michael
Frontend Developer | Javascript programmer | Registered Mechanical Engineer (MNSE, COREN) | Facilities Management Technologies Developer