Java Arrays
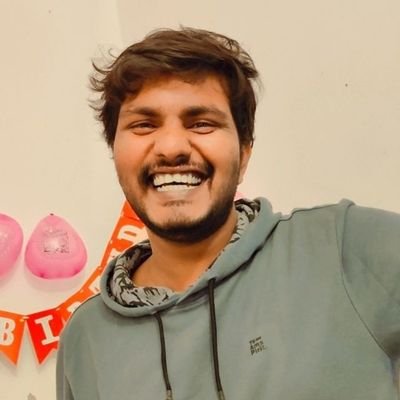
Table of contents
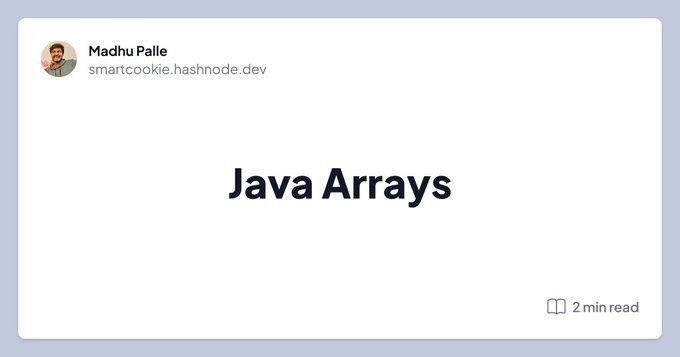
Arrays: Arrays can be used to store multiple values
in a single variable
.
Instead of using separate variables for each value, we will declare an array to store multiple values in a single variable.
To use the java arrays
we need to define the array
first. The array can be declared with square brackets [ ]
.
// syntax for array decleration
String[] names; //names is the variable
//it is a string type array which contains the names
We can directly assign
the values to the array.
//here we are assigning the names to the names variable
String[] names = {"abc","def","ghi","jkl","mno","pqr","stu","vwx","yz"};
we can also create an array of integers
like this
int [] numbers = {1,2,3,4,5,6,7,8,9};
Element Accessing in the Array:
to access some specific elements, we need to call the array
and pass the index
number to the array.
In java array index starts from 0
. 0 is the first element
, 1 is the second element
and so on.
public class Main{
public static void main(String[] args){
String[] names = {"abc","def","ghi","jkl","mno","pqr"};
System.out.println(names[0]); // o th index position element => abc
System.out.println(names[2]); // 2 nd index position element => ghi
System.out.println(names[5]); // 5 th indext position element => pqr
}
}
Output:
Java arrays are Mutable
that means we can change
or re-assign
the new value to it even after declaring the value.
public class Main{
public static void main(String[] args){
String[] names = {"abc","def","ghi","jkl","mno","pqr"};
System.out.println("Before : "+ names[5]); // before to the 5th index
names[5] = "smart cookie"; // assign new value to 5th index
System.out.println("After :" + names[5]); //after assigning
}
}
Array Length :
we can also find the total length
of the array by using the length property
.
The length
property can be helpful to tell how many elements are there in the entire array.
The Array
length count
can be started from 1.
public class Main{
public static void main(String[] args){
String[] names = {"abc","def","ghi","jkl"}; //string type array
names[2] = "smart cookie"; // assigning the new value
System.out.println(names.length); //count the length
}
}
Subscribe to my newsletter
Read articles from Madhu Palle directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
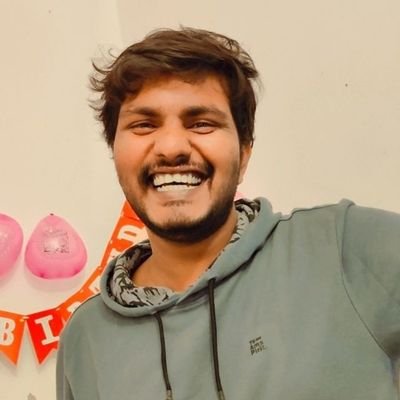
Madhu Palle
Madhu Palle
IIIT - N Software Geek. Startup Enthusiast. Build for the Future.