Java For Loop
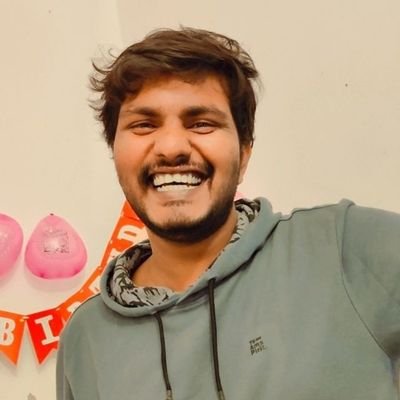
Table of contents
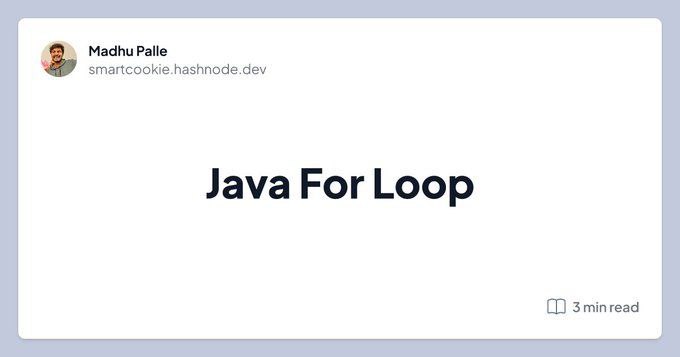
Loops: Loops
it is a special feature used to execute a particular task of the program repeatedly
when a certain condition is true
.
For repetitive tasks or work, we always go with the loops
concepts because loops will reduce the work.
Mainly we have 2 loops available in java which are
For
loopWhile
Loop
For Loop :
When you know exactly how many times a particular task is to be executed
then using the for loop
is suggestable.
//syntax for for loop
for (initialization ; condtion ; incrementation){
//code block to be executed
}
Explanation :
step1: To use any variable
, we need to initialize
first and then use it.
step2: After initialization, we need to specify the condition
.
step3: In the next step we need to increment / decrement
to the next process.
For Loop Example :
public class Main{
public static void main(String[] args){
for(int i=0 ; i<5; i++){
System.out.println("Number : " + i);
}
}
}
Explanation :
Step 1: Printing The first 5 elements using for loop
.
Step 2: First, we initialize an integer variable i
to 0
i.e,i = 0;
Step 3: Then we need to give the condition as print the first 5 numbers
so we are writing the condition like i < 5;
which will print the first 5 numbers starting from 0
.
Step 4: After completing one iteration we need to increment / decrement
the process. So that it will loop through all the items until the condition becomes false
.
Step 5: At each iteration i =0,1,2,3,4
it will come inside the for loop and it will print the output.
Step 6: Whenever the condition is false
i.e, i = 5
which means 5 < 5
, it will break out of the for loop and exit the program.
Output :
Java Nested For Loops:
In java, Nested for loops
is also possible which means we can write loops inside another loop
that is called "nested for loop
".
The inner loop
can be executed one time for every iteration of the outer loop
.
public class Main {
public static void main(String[] args) {
// Outer loop.
for (int i = 1; i <= 2; i++) {
System.out.println("Outer: " + i); // Executes 2 times
// Inner loop
for (int j = 1; j <= 3; j++) {
System.out.println(" Inner: " + j); // Executes 6 times (2 * 3)
}
}
}
}
Explanation :
Step 1: For the first loop
which is i
that is starting from 1 and checking the condition i.e,i <= 2
that is true so it will go inside the loop.
Step 2: And inside the for loop we have another for loop that is initiated to j = 1
and it checks till j< = 3
.
Step 3: In the first for loop the condition is true 1 < 2
, so it will go inside the loop
and print the j loop items
one time and increment
it and it will come out of the loop one time.
Step 4: Till now 1 time the loop gets executed
, and again it checks for the condition whether it meets the condition or not i.e, 2<=2
which is true
so it will go inside the j
loop once again and print the j loop
items.
Step 5: The loops get executed until the both i and j
loops become false
.
Step 6: If both the inner and outer loop
condition becomes false
it will come out of the for loop
and exit the program.
Output:
Subscribe to my newsletter
Read articles from Madhu Palle directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
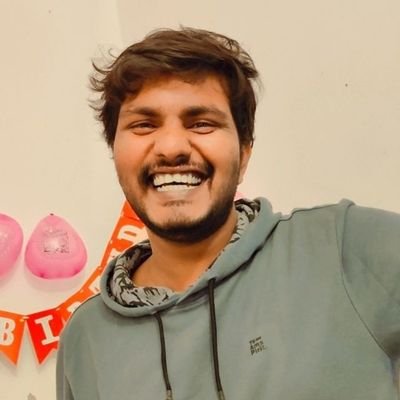
Madhu Palle
Madhu Palle
IIIT - N Software Geek. Startup Enthusiast. Build for the Future.