Lists in Python
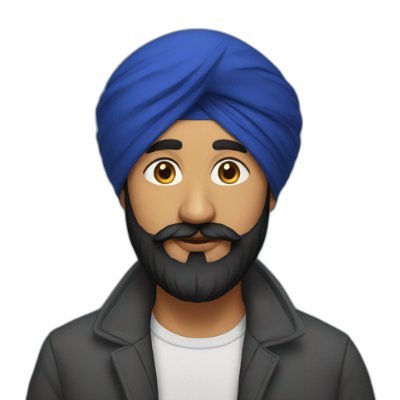
Table of contents
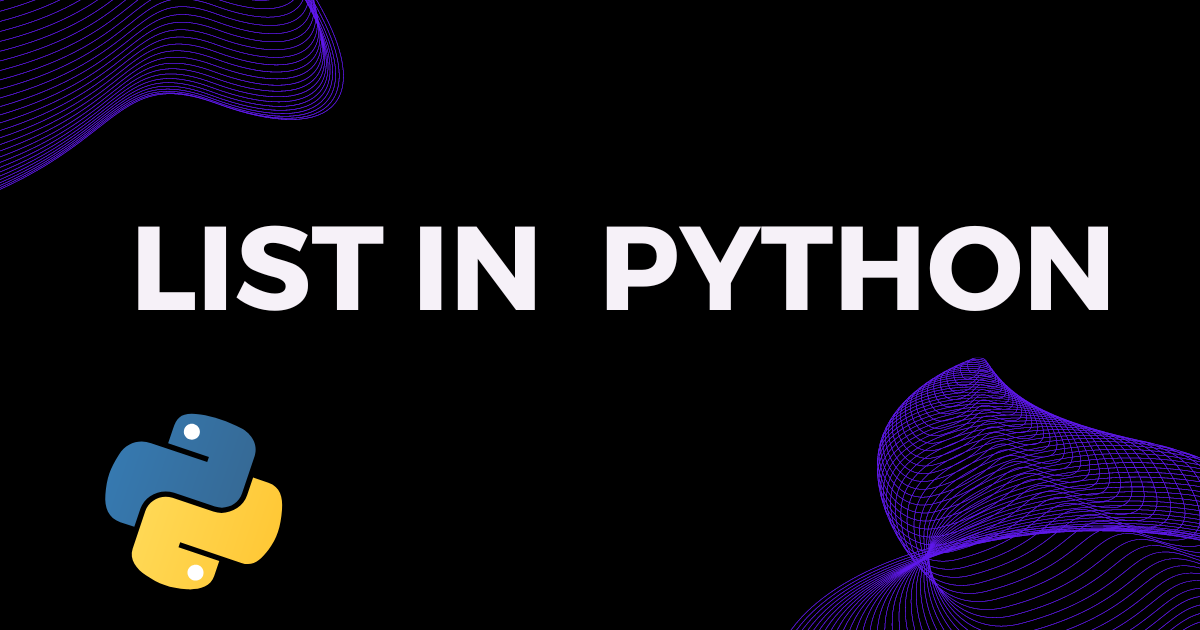
Are you seeking a resource that can teach you all there is to know about lists in Python? If so, you've come to the correct spot. You'll be equipped to work with list data structures once you've finished reading this blog post. Let's get started without further delay.
What is a list in python and how is it different from arrays?
List is an ordered data structure in Python that is dynamic and can be used to store values of different data types. List writing's fundamental syntax is [ ].
list = [1, 'Yuvraj' , 45.6 , [1,2,3]]
How the list is different from an array?
Let's now discuss the distinctions between a list and an array. Lists and arrays differ in a few ways, but they also have two things in common: they both use the notion of indexing, which allows us to quickly traverse through and access the items that are stored inside, and both are mutable, which means that we can change the values stored in them.
List | Array |
The list is dynamic, which simply means that we can easily manipulate the overall size of the list during runtime. | An array is static, which means that before storing values in it, we first need to specify how many values we want to store in it. |
The list can store values of different data types | The array can be used to store the value of only the same datatype |
Execution speed is slower than an array | Execution speed is faster than the list |
Similarities between list and array
Both lists and arrays use zero-based indexing
Both arrays and lists are ordered data structures, which means that the elements of an array are stored in a specific order
Types of Lists in python
In Python, there are 4 types of lists: an empty list, a 1D list, a 2D list, and a 3D list.
# Types of list
list_empty = []
list_1d = [1,2,3]
list_2d = [[1,2,3] , [4,5,6] , 7]
list_3d = [[[1,2,3],[4,5,6]],[[7,8,9],[10,11,12]]]
Question: Does list_2d have homogeneity or heterogeneity?
Answer: List_2d is heterogeneous because in that not all of the values it stores are of the same data type.
Keep in mind that the way we access elements from a 2D and 3D list will differ from that of a 1D list. To access 1 from list_1d, list_2d, and list_3d, for example, we would need to write print(list_1d[0]), print(list_2d[0][0], and print(list_3d [0] [0].
How the list is stored in memory?
A list is also known as a referential array, where a referential array simply means a type of array in which, instead of storing the values directly, we are storing the addresses of those values. To better understand this let us assume that we have made a list having 3 elements: list = [ 1, 2, 3 ].
The Python interpreter will allocate three blocks internally, and within those three blocks, the address where the values are stored in memory will be stored.
You can also check this by yourself by using id( )
that simply gives us the memory address of the parameter passed into it.
Methods for adding items to a list
There are three different methods for adding items to the list, and we can choose any one of them based on our needs, such as how we want the item to be added to the list.
append( ) | extend( ) | insert( ) |
append( ) method is used to add a new item at the end of the list | extend( ) method is used to add multiple values at the end of the list | insert( ) method is used to add a new item at a specific position in the list |
list = [1,2,3]
# For adding single item at the end of the list
list.append(4)
# For adding multiple items at the end of the list
list.extend([5,6,7])
# For adding item at specific position in the list -> insert(index,value)
list.insert(7,8)
Different ways to remove an item from the list
We can delete items from the list in the same manner that we can add items to it, and there are 4 ways to do this: delete method, pop method, del keyword, and
Let's start with the "del" keyword first. In essence, the del keyword allows us to remove items from a list depending on their index. Let's imagine that we have a list of five items, and out of those five items, we want to remove item 4 from the list, so for doing this code is given below.
list = [1,2,3,4,5]
del list[4]
# We cal remove value from the list using slicing as well
del list[1:3]
Moving on to the removal method, it should be noted that this method is used when we want to remove a value from a list but are unsure of the index at which it is present. As a result, we send the value we wish to remove from the list as an argument to the remove function.
list = [1,2,3,4,5]
list.remove(4)
To remove an item from a list, we may also use the pop method. However, we need to be aware that pop behaves differently depending on whether we supply the index of the item to be removed as an argument or not. In essence, the pop method removes the value that is present at the index that is supplied, but if we don't provide it, it removes the last item from the list by default.
list = [1,2,3,4,5]
list.pop(4)
# This will remove 5 from the list
list.pop()
Finally, a clear()
can be used to make a list empty by removing all the items from the list.
list = [1,2,3,4,5]
list.clear()
Operations on the list
On list data structure, a total of three different types of operations can be performed: traversal, member operations, and arithmetic operations.
hen conducting arithmetic operations on the list, there are just two possible operators that we can employ + and *
list1 = [1,2,3]
list2 = [4,5,6,[7,8]]
# Arithmetic operators (+ and *)
print(list1 + list2) -> 1,2,3,4,5,6
print(list1 * 2) -> 1,2,3,1,2,3
# Membership operation
print(5 in list1) -> Will give true
print(7 in list2) -> Will give false
print([7,8] in list2) -> Will give true
# Itemwise traversal
for item in list1:
print(item)
# Index-wise traversal
for i in range(0,len(list1)):
print(list1[i])
List functions
Aside from append(), extend(), insert(), remove(), clear(), and pop()
, we are unaware of a few more functions. Those functions are as follows:
list = [1,2,3]
# For finding lenght of th list
print(len(list))
# For finding maximum value in homogenous numerical list
print(max(list))
# For finding minimum value in homogenous numerical list
print(min(list))
# For finding index of a value
print(list.index(2))
# For doing inplace sorting in ascending order
list.sort()
# For doing inplace sorting in descending order
list.sort(reverse = True)
# For doing out-of-place sorting in ascending order
print(sorted(list))
# For doing out-of-place sorting in descending order
print(sorted(list,reverse = True))
List comprehension
List comprehension, by definition, is a concise way to create a list. The basic syntax for creating a list using list comprehension is [ expression for item in iterable if condition ]
To better understand the power of lists let's imagine we have a list with five items in it and want to construct another list with items multiplied by 2 .
Making a list without using the list comprehension concept
list = [1,2,3,4,5] new_list = [] for item in list: new_list.append(item*2)
Making a list using list comprehension
list = [1,2,3,4,5] new_list = [i*2 for item in list] # Another example : Imagine we want to make a list from 1 to 100 using items that are multiples of 2. list = [i for i in range(1,101) if i%2 == 0]
Now that you hopefully have a better understanding of list comprehension, let's look at nested list comprehension.
Ways to traverse in a list
There are essentially two methods for traversing a list: itemwise and indexwise.
list = [1,2,3,4,5]
# Item-wise traversal
for item in list:
print(item)
# Index-wise traversal
for i in range(0,len(list)):
print(list[i])
That's all for now, and I hope this blog provided some useful information for you. Additionally, don't forget to check out my ๐ TWITTER handle if you want to receive daily content relating to data science, mathematics for machine learning, python, and SQL.
Subscribe to my newsletter
Read articles from Yuvraj Singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
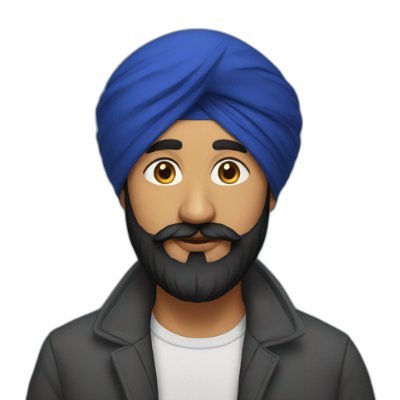
Yuvraj Singh
Yuvraj Singh
With hands-on experience from my internships at Samsung R&D and Wictronix, where I worked on innovative algorithms and AI solutions, as well as my role as a Microsoft Learn Student Ambassador teaching over 250 students globally, I bring a wealth of practical knowledge to my Hashnode blog. As a three-time award-winning blogger with over 2400 unique readers, my content spans data science, machine learning, and AI, offering detailed tutorials, practical insights, and the latest research. My goal is to share valuable knowledge, drive innovation, and enhance the understanding of complex technical concepts within the data science community.