5 Essential JavaScript Concepts for Beginners
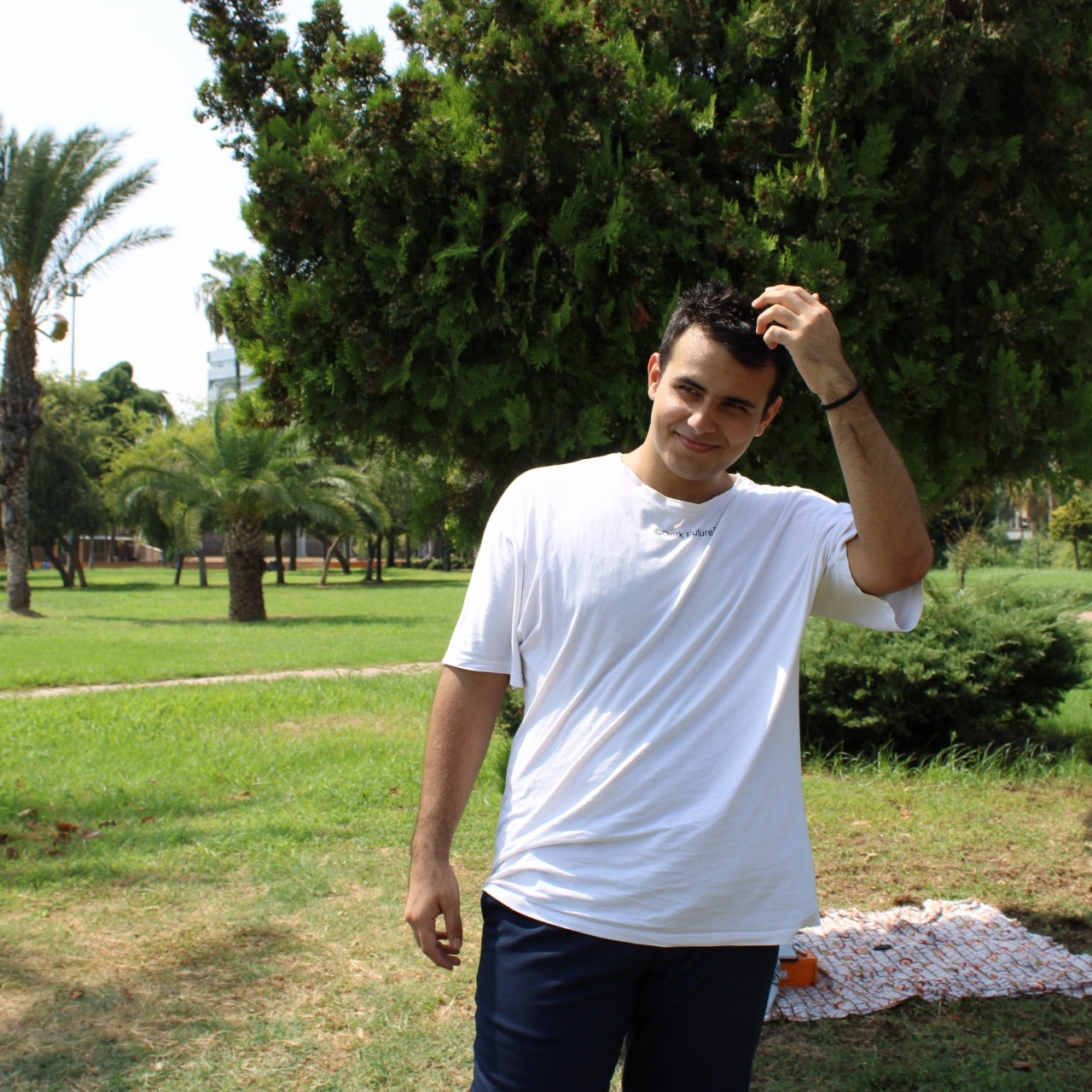
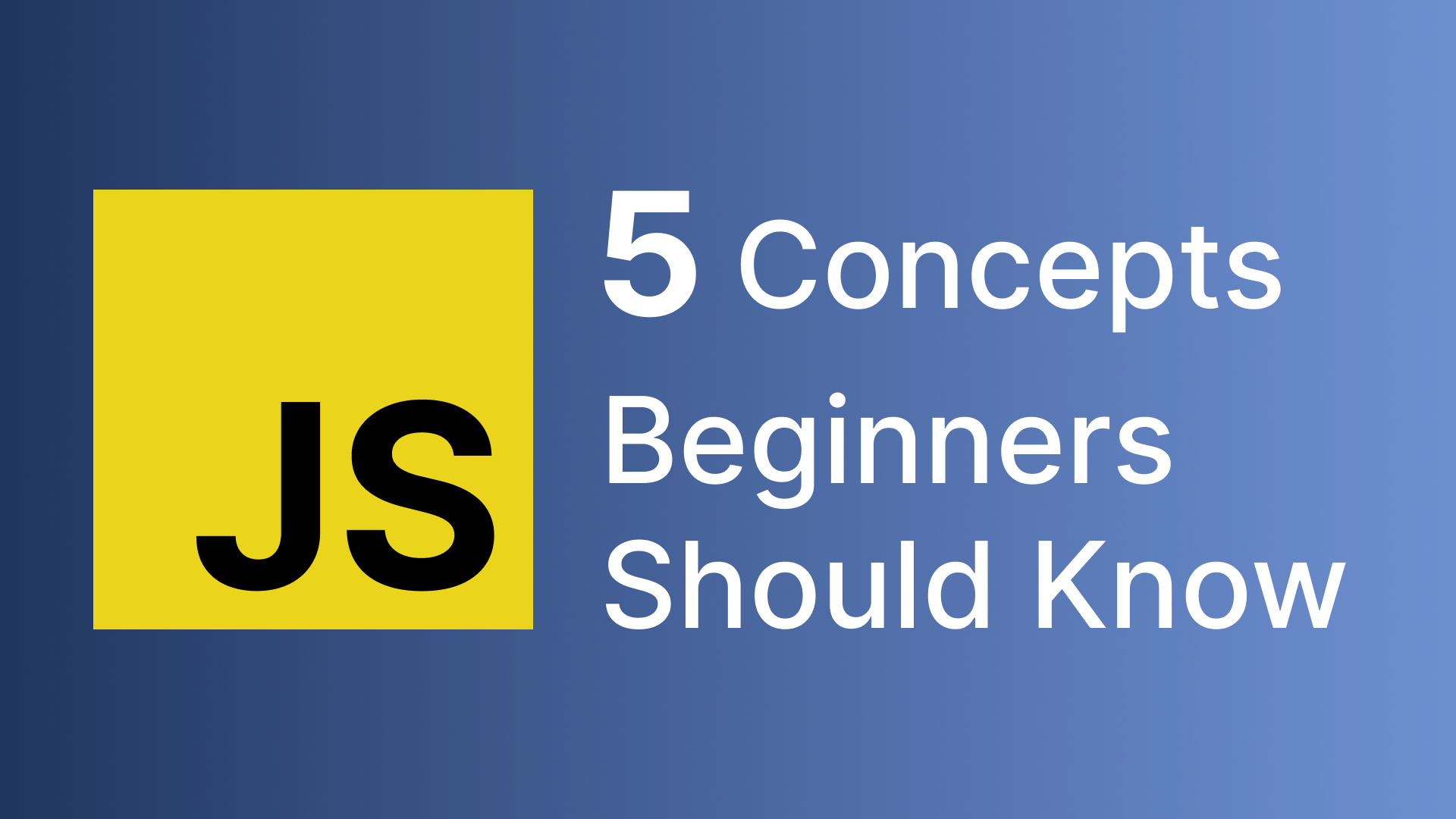
Here Are 5 Essential JavaScript Concepts:
1. Variables
Variables are used to store and manipulate data in JavaScript. They can be declared using var
, let
, and const
keywords and can be assigned a value using the assignment operator =
.
For Example:
var widthOfBox = 25;
let isBox = true;
const boxName = "Cool Box";
When should you use the `var` keyword to declare a variable
You should use var
keyword if you want your variable to accessible to the entire function within where it's declared. It can be a little complicated to understand at first so let's give an example:
function myFunction(){
var myVariable = 10;
// You can access to myVariable entirely in myFunction
console.log(myVariable); // logs 10
while(myVariable > 0)
{
console.log(myVariable); // logs 10
myVariable--;
}
}
function anotherFunction(){
//This function doesn't know myVariable
console.log(myVariable) // error
}
As you can see, we declared a variable with var myVariable = 10
. We've declared it as a function. So you can use myVariable
if you are in the function but you can't use it in another function because it's outside of our myFunction
. As a result var keyword is function-level scoped.
When should you use the `let` keyword to declare a variable
let
keyword is block-scoped. That means it is accessible by the block of its declared. Let's say you created an if statement and declared a variable with
let myVariable = 10;
. You can use this variable within the if statement brackets only. Let's show it with the real javascript code:
function multiplyByTwo(arg){
if(arg > 10)
{
let result = arg * 2;
console.log(result) // arg * 2
}
console.log(result) // error
}
We have created a function that gets a parameter and multiplies it by 2 and stores it in the `result` variable. We can see log the result in the if statement but we can't outside of the if.
When should you use the `const` keyword to declare a variable?
const
is also block-scoped. It's similar to the let
keyword but the only difference is variables that are declared with the const keyword can not be reassigned or changed. When it's declared, it holds the value entire program.
const capacity = 50;
console.log(capacity) // logs 50
capacity = 25; // error 'const' can not be changed or redeclared
2. Functions
Functions are blocks of code that can be defined and then called by name. They can accept parameters and return a value. Functions are a key concept in JavaScript and are used to organize and reuse code.
//Example function
function myFunction(param1, param2){
return x; // Returns the x value
}
3. Objects
Objects are collections of key-value pairs that represent real-world objects or abstract concepts. They are a fundamental building block of JavaScript and are used to model data and behavior. Let's look at how is it looks like:
const student = {
id = 2022,
name = "Tahaberk",
studentClass = 2
};
const student std1;
console.log(std1.name); // logs "Tahaberk"
So here we declared an object called a student. It has some keys and values. Every instance of a student object can have different values.
4. Arrays
Arrays are ordered collections of values. They are used to store lists of data and can contain elements of any data type.
const studentIds = [2001, 2002, 2003, 2004, 2005]; // Array created
console.log(studentIds[0]); // logs first value of studentIds array => 2001
5. Control Structures
Control structures are used to control the flow of execution in a program. JavaScript has several control structures, including if
statements, for
loops, and while
loops, which allow you to write code that can make decisions and repeat actions.
That concepts are must know for every programming language. You can start your JavaScript journey by learning these concepts. I just tried to introduce the concepts for beginners you should learn more and practice these concepts to master them.
Subscribe to my newsletter
Read articles from Tahaberk SOYSAL directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
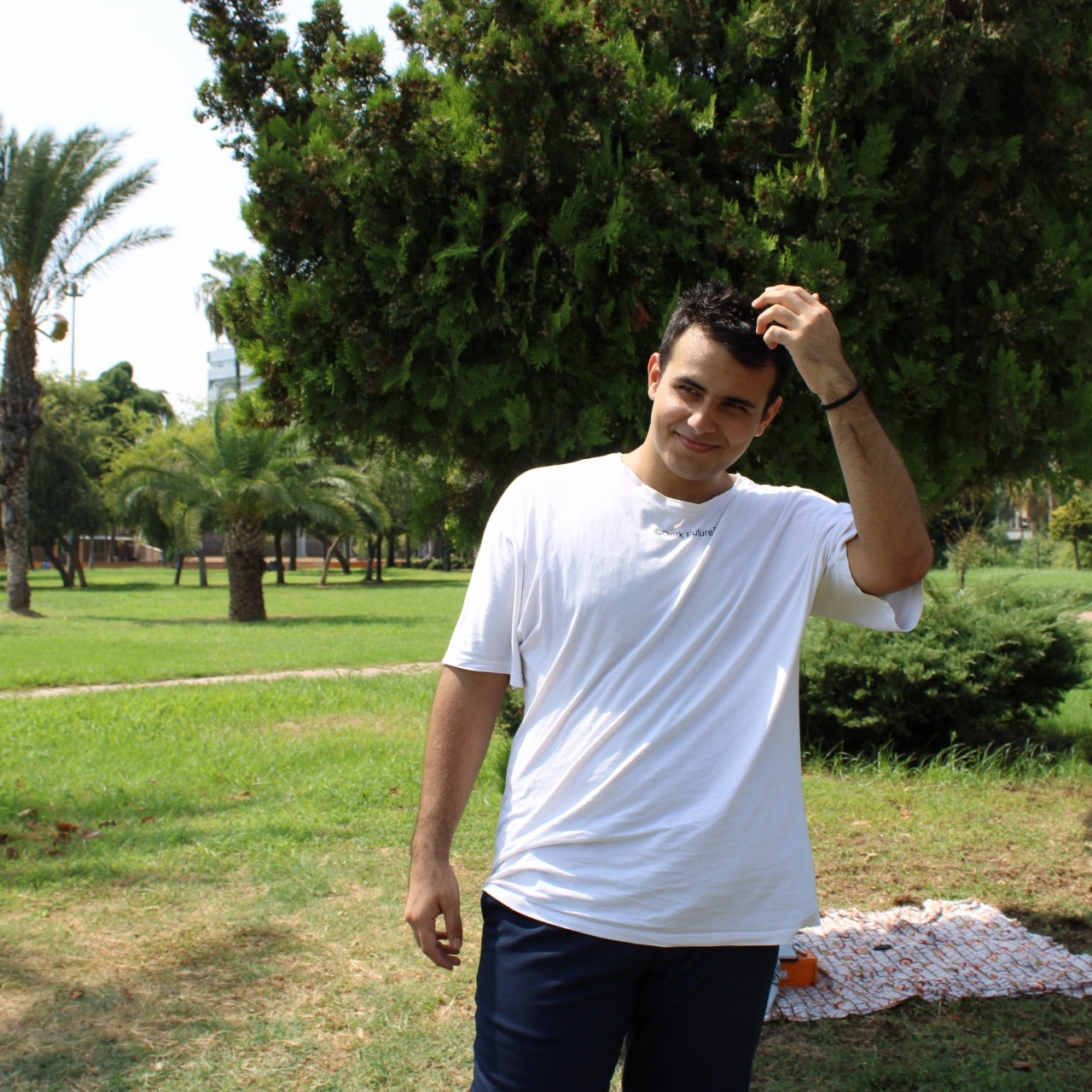
Tahaberk SOYSAL
Tahaberk SOYSAL
I am a self-taught developer for 5 years so far. I'm 21 years old and I'm from Turkey. I am going to write articles about web programming (Html, Css, JavaScript etc.) and simply try to explain some concepts to you. I will also share my coding projects and would want to get feedback and interact with you. Let's learn to code !!