Virtual Dom In React

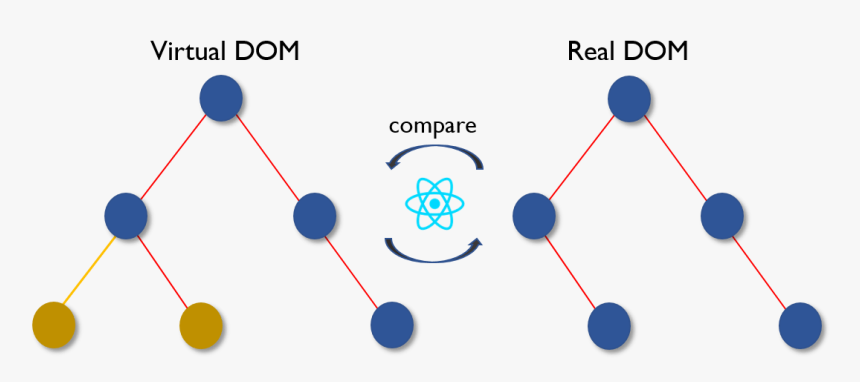
In React, the virtual DOM is used to improve the performance of a web application by minimizing the number of DOM updates that are needed when the user interacts with the application. This is achieved through a process called "diffing" and "reconciliation".
Diffing
Diffing" refers to the process of comparing the current virtual DOM tree with a previous virtual DOM tree, and creating a list of differences (or "patches") between the two trees. These patches describe the minimal set of changes needed to be made to the actual DOM in order to bring it in line with the current virtual DOM tree.
Reconciliation
"Reconciliation" refers to the process of applying the patches to the actual DOM, updating only the nodes that have changed. This can be done more efficiently than updating the entire DOM on each change, as it allows the virtual DOM to batch multiple changes into a single update.
Here's an example of how diffing and reconciliation work in a React application:
import { useState } from "react";
const Test = () => {
const [count, setCount] = useState(0);
const incCount =()=>{
setCount(count+1)
}
return (
<div>
<p>{count}</p>
<button onClick={incCount}>increment</button>
</div>
);
};
export default Test;
In this example, the Test
component has a state variable called count
that is displayed in a p
element. When the user clicks the Increment
button, the incCount()
function is called, which updates the component's state by incrementing the count
variable. This causes the component's render()
function to be called, which generates a new virtual DOM tree and applies any necessary patches to the actual DOM.
The virtual DOM then compares the new virtual DOM tree with the previous virtual DOM tree, and creates a list of patches that describe the differences between the two trees. In this case, the patch consists of changing the text of the p
element to display a count of 1.
Finally, the virtual DOM applies the patches to the actual DOM, updating the p
element to display a count of 1.
Pros of virtual DOM:
Improved performance: Virtual DOM can significantly improve the performance of a web application by minimizing the number of DOM updates that are needed when the user interacts with the application. This is because the virtual DOM can efficiently determine the minimal set of changes needed to be made to the actual DOM, and then apply those changes in a single batch, rather than updating the DOM on each individual change.
Declarative code: By using a virtual DOM, a developer can write declarative code that describes the desired state of the application, rather than imperative code that describes the steps needed to achieve that state. This can make the code easier to understand and maintain.
Reusable components: Virtual DOM frameworks like React allow developers to create reusable components that can be easily composed to build complex applications. This can save time and improve the maintainability of the application.
Cons of virtual DOM:
Additional complexity: Using a virtual DOM adds an additional layer of abstraction to the codebase, which can make it more difficult to understand and debug.
Extra memory usage: The virtual DOM requires additional memory to store the virtual DOM tree, which can increase the overall memory usage of the application.
Slower initial rendering: Because the virtual DOM requires an extra step of creating and comparing the virtual DOM tree before updating the actual DOM, it can be slower than directly updating the DOM in some cases, especially for small applications or applications with a simple user interface.
Conclusion
the benefits of using a virtual DOM often outweigh the drawbacks, especially for larger and more complex applications. However, it's important to carefully consider the specific requirements and constraints of an application before deciding whether to use a virtual DOM.
Subscribe to my newsletter
Read articles from jyoti directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

jyoti
jyoti
im a full stack web developer skilled in reactjs javascript and other mern technologies