Flutter MVVM App Architecture using Riverpod - Flutter Example
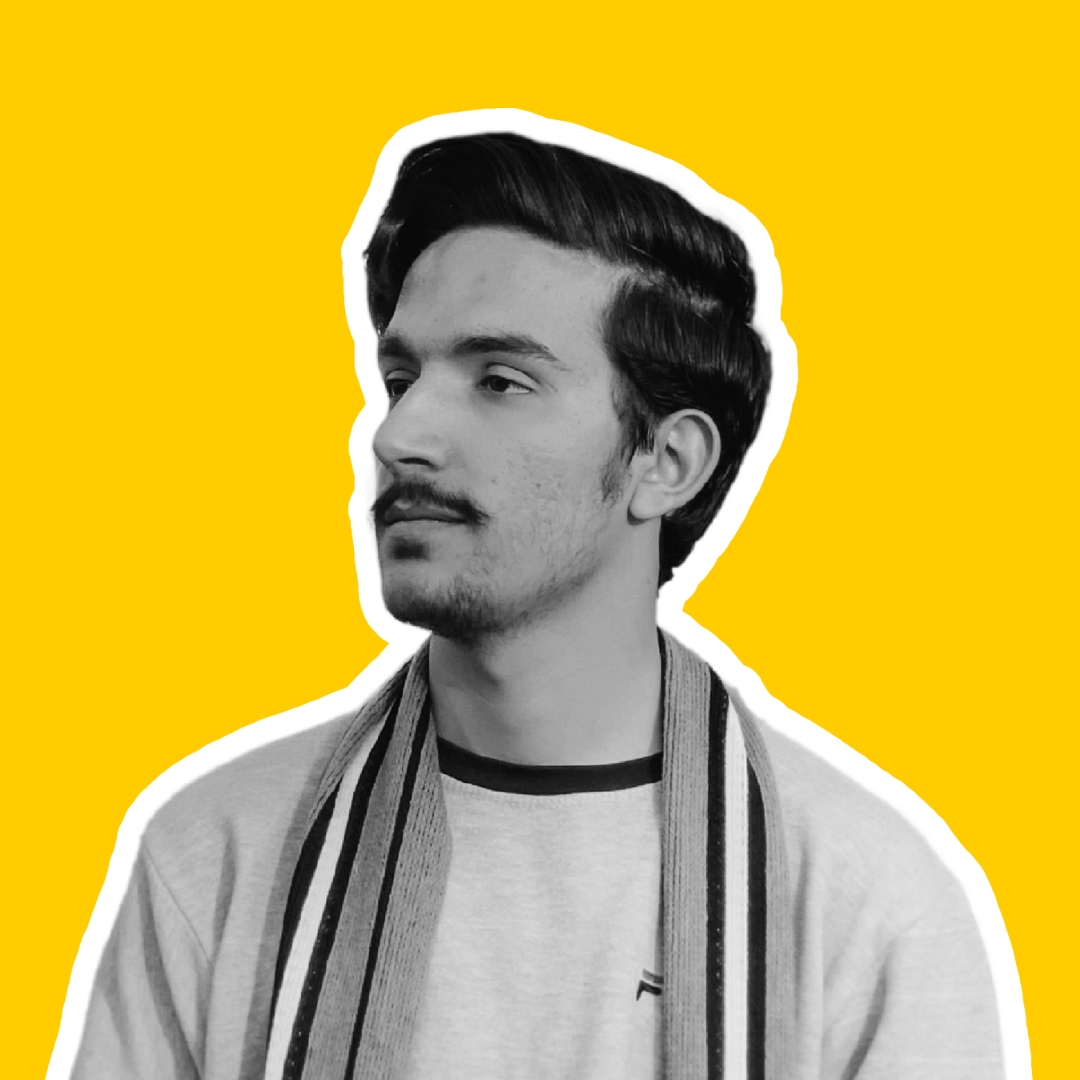
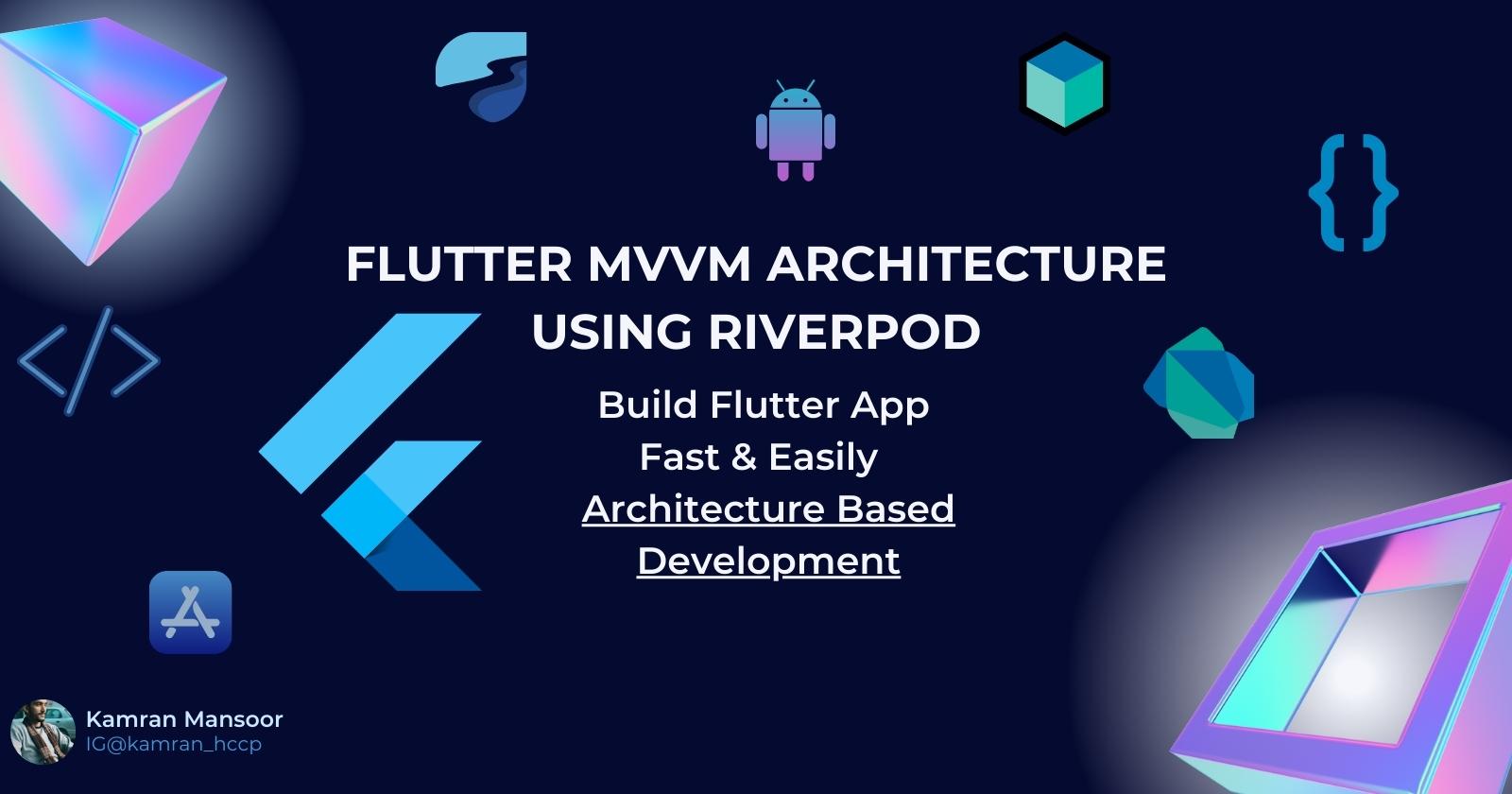
Architecture is the systematized arrangement and construction of a software system. It outlines the components of the system, the way they interact and cooperates with one another, and their relationships.
Selecting the proper architecture for an app is crucial as it can influence the codebase's structure, handiness, and performance. A well-constructed architecture can make the app simpler to develop and manage, while a poorly designed architecture can make the app challenging to understand and modify.
There are numerous architectures that can be utilized for app development, each having its own advantages and disadvantages.
Several Architecture used for Flutter Application Development
Model-View-Controller (MVC):
MVC is a traditional architecture that divides the app into three fundamental components: the Model, which stands for the data and business logic; the View, which represents the user interface; and the Controller, which mediates between the Model and the View.
Model-View-Presenter (MVP):
In MVP, the Presenter acts as a mediator between the View and the Model, handling the business logic and updating the View. This architecture can be useful for testing the Presenter in isolation from the View.
Model-View-ViewModel (MVVM):
MVVM separates the presentation of data from the business logic, with the ViewModel acting as a link between the View and the Model. This architecture can be useful for reusing the ViewModel across multiple Views.
BLoC (Business Logic Components):
BLoC is a reactive architecture that uses streams and sinks to communicate between the View and the business logic. It can be useful for building apps that require real-time data updates.
Ultimately, the choice of architecture will depend on the needs of the app and the preferences of the development team. It's important to choose an architecture that is well-suited to the app and that can be easily maintained and modified as the app evolves over time.
1. Introduction to MVVM
Model-View-ViewModel (MVVM) is a software architectural pattern that separates the presentation of data from the business logic. In MVVM, the ViewModel acts as a link between the View and the Model, enabling the View to bind to the ViewModel and display the data it represents.
MVVM is a popular pattern for building cross-platform applications because it allows developers to reuse the ViewModel across different platforms. For example, the same ViewModel can be used in a Flutter app for mobile devices and a web app using Angular or React.
2. Introduction to Riverpod
Riverpod is a state management library for Flutter that uses the Provider pattern. It allows developers to manage the state of their Flutter app simply and consistently.
The Provider pattern is widely used in Flutter for exposing values to the rest of the app. A Provider is a widget that holds a value and tells it to the rest of the app through a build context. Any widget can access the value in the app by using a Consumer widget, which takes a builder function that receives the value from the Provider as an argument.
Riverpod builds on the Provider pattern by adding features such as dependency injection, lazy evaluation, and scoped providers. These features make it easier to manage the state of a Flutter app and test the app's business logic.
3. Using MVVM with Riverpod in Flutter
To use MVVM in a Flutter app with Riverpod, you would define a ViewModel class that represents the app's state and contains the business logic. You would then create a Provider that holds an instance of the ViewModel and exposes it to the rest of the app. The View would bind to the ViewModel through the Provider and display the data it represents.
In this tutorial, we'll see how to use MVVM in a Flutter app with Riverpod.
Setting up the project
First, let's create a new Flutter project using the following command:
Copy codeflutter create mvvm_app
Next, we'll add the hooks_riverpod
and flutter_hooks
dependencies to our pubspec.yaml
file:
Copy codedependencies:
flutter:
sdk: flutter
# Add the following dependencies
hooks_riverpod: ^0.7.0
flutter_hooks: ^0.7.0
Then, run flutter pub get
to install the dependencies.
Defining the ViewModel
Let's start by defining the ViewModel for our app. In this example, we'll create a simple counter app that increments a count when a button is pressed.
Create a new file called counter_view_model.dart
and add the following code:
Copy codeimport 'package:flutter/widgets.dart';
class CounterViewModel {
int _count = 0;
int get count => _count;
void increment() {
_count++;
}
}
The CounterViewModel
class contains the state for our app and the logic for incrementing the count. It exposes a count
property that represents the current count, and a increment
method that increments the count.
Creating the Provider
Next, we'll create a Provider for the CounterViewModel
. A Provider is a widget that holds a value and exposes it to the rest of the app.
In the root of our app, create a new file called providers.dart
and add the following code:
Copy codeimport 'package:hooks_riverpod/hooks_riverpod.dart';
import 'package:mvvm_app/counter_view_model.dart';
final counterProvider = Provider((ref) => CounterViewModel());
Here, we've defined a counterProvider
that holds an instance of the CounterViewModel
and exposes it to the rest of the app.
Building the View
Now, let's build the View for our app. In the main.dart
file, replace the default MyApp
widget with the following code:
Copy codeimport 'package:flutter/material.dart';
import 'package:hooks_riverpod/hooks_
Benefits of using MVVM in Flutter with Riverpod
Using an architecture like MVVM in a Flutter app can help to improve the structure and maintainability of the codebase. It can also make it easier to test and develop features.
Some of the benefits of using MVVM in Flutter with Riverpod include:
Separation of concerns:
MVVM helps to separate the presentation of data from the business logic, which can make the code easier to understand and maintain.
Improved structure:
An architecture can help to organize the code into logical, reusable components that are easier to understand and maintain.
Reusability:
The ViewModel can be reused across multiple Views, which means you can reuse the same business logic in different parts of the app.
Testability:
The ViewModel can be tested in isolation from the View, which makes it easier to test the business logic of the app.
Modularity:
MVVM allows developers to break the app into smaller, more modular components that are easier to understand and maintain.
Ease of use:
Riverpod makes it easy to manage the state of the app and bind it to the ViewModel, which can save developers time and make the code easier to write.
Overall, using MVVM with Riverpod can help to improve the structure, maintainability, and testability of a Flutter app, which can make it easier to develop and maintain over time.
Thank you for Reading this blog. Hope this blog helps you and if you have any problem, don't hesitate to contact me through the contact page.
Subscribe to my newsletter
Read articles from Kamran Mansoor directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
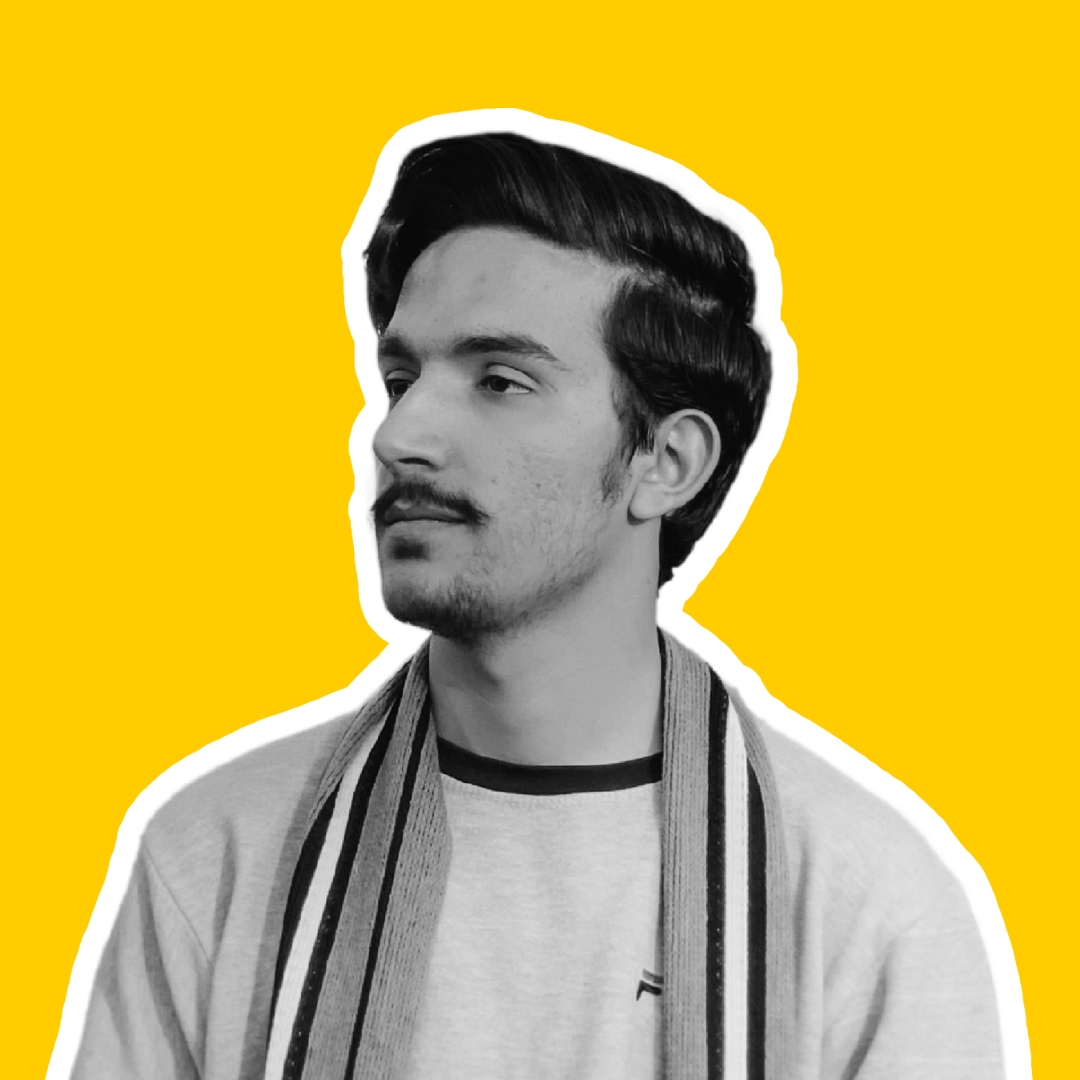
Kamran Mansoor
Kamran Mansoor
Student: Software Engineer. Junior Flutter Developer Data Scientist == Entrepreneur 🤞. COMSATS' 24. @kamran_hccp