[Solution]237. Delete Node in a Linked List

1 min read
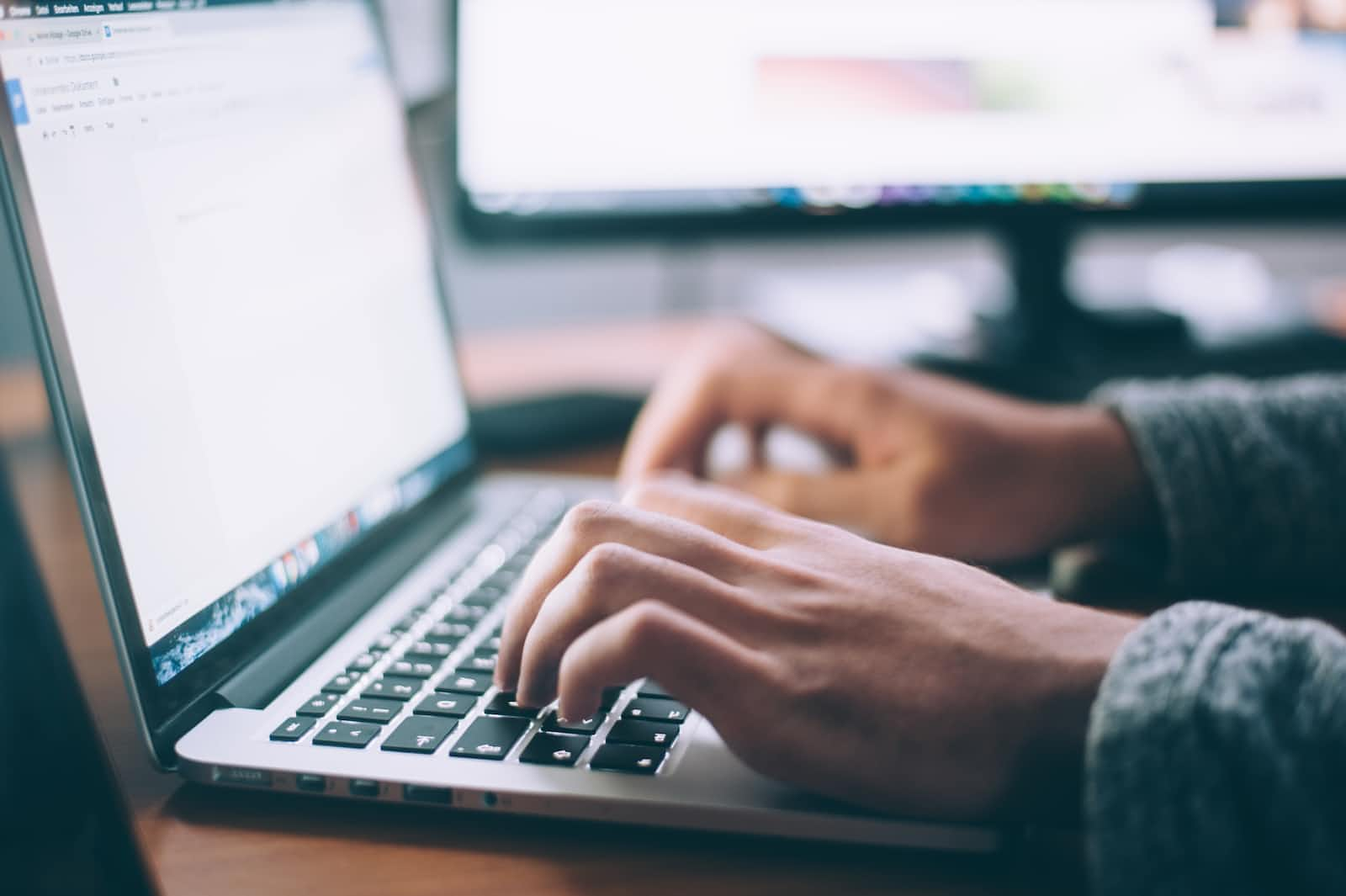
Problem
Solutions (time, space)
O(1), O(1)
/**
* Definition for singly-linked list.
* public class ListNode {
* int val;
* ListNode next;
* ListNode(int x) { val = x; }
* }
*/
class Solution {
public void deleteNode(ListNode node) {
ListNode nextNode = node.next;
node.val = nextNode.val;
node.next = nextNode.next;
nextNode.next = null;
}
}
Explanation
copy value from the next node
connect the current node to next.next node
disconnect next node to next.next node
bad code
class Solution {
public void deleteNode(ListNode node) {
node.val = node.next.val;
node.next = node.next.next;
}
}
This is bad code. It may cause a memory leak.
The next node has to be disconnected.
0
Subscribe to my newsletter
Read articles from Han directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
