Notification & Observer Pattern

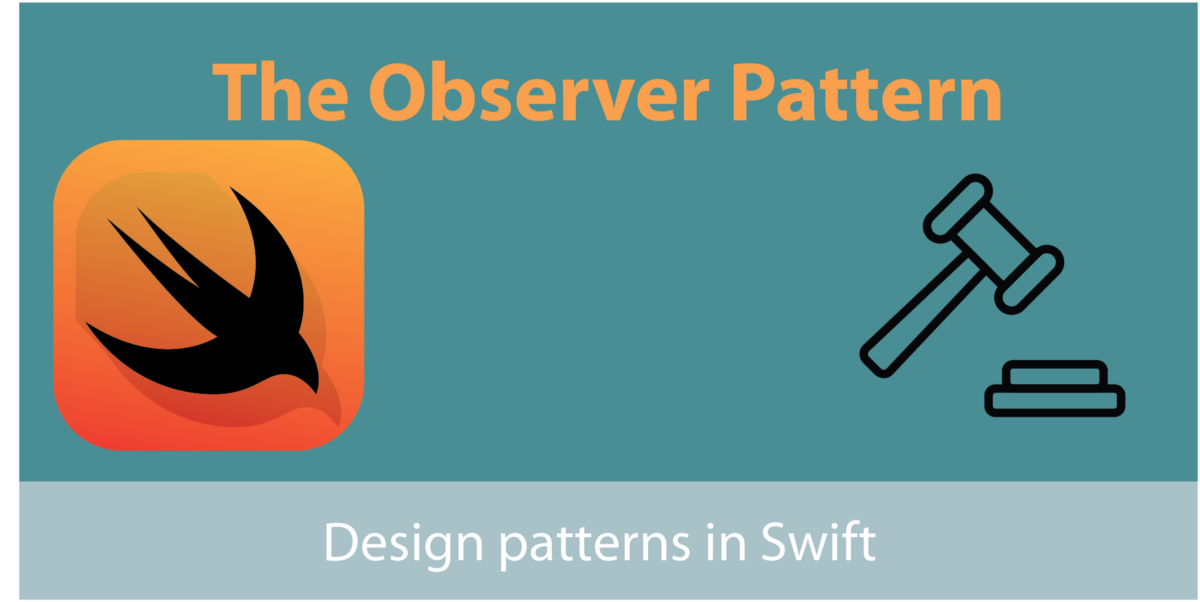
The notification/observer communication pattern is useful when you need parts of your code to respond to events triggered in separate areas of the app (e.g. in separate methods or even separate classes). A concrete example is using the notification pattern to dismiss the keyboard when a certain part of the screen is tapped. In Swift, this pattern is most commonly implemented using Apple’s NotificationCenter API.
There are 2 parts to notifications:
Register: specifying the observer that will listen for these notifications, via the addObserver method.
Broadcast: sending out these notifications and triggering method calls, via the post method.
I like to use the following analogy to explain and remember the pattern: The observers are the paparazzi, who get a “CelebritySightingNotification” whenever there’s a celebrity sighting. Once they get this notification, they respond by posting to the tabloids, which maps to the selector
parameter in the examples below.
Registering:
// This is just the notification setup; this code alone doesn’t trigger anything. Wherever it does get triggered (see next code example), the selector, in this case postToTabloids, will execute.
NotificationCenter.default.addObserver(observer: paparazzi,
selector: #selector(PaparazziTasks.postToTabloids()),
name: Notification.name(rawValue: "CelebritySightingNotification"),
object: nil)
Broadcasting (trigger):
func checkForCelebrities() {
if celebrity > 0 {
// This default.post call triggers postToTabloids to get called.
NotificationCenter.default.post(name: Notification.name(rawValue: "CelebritySightingNotification"),
object: nil,
userInfo: ["CelebritySightingNotification": false])
}
}
Subscribe to my newsletter
Read articles from Christine Chang directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Christine Chang
Christine Chang
Passionate about: Defensive Programming • Effective Engineering Documentation • Emotion-Centered Design and Microcopy