CSS Media Queries

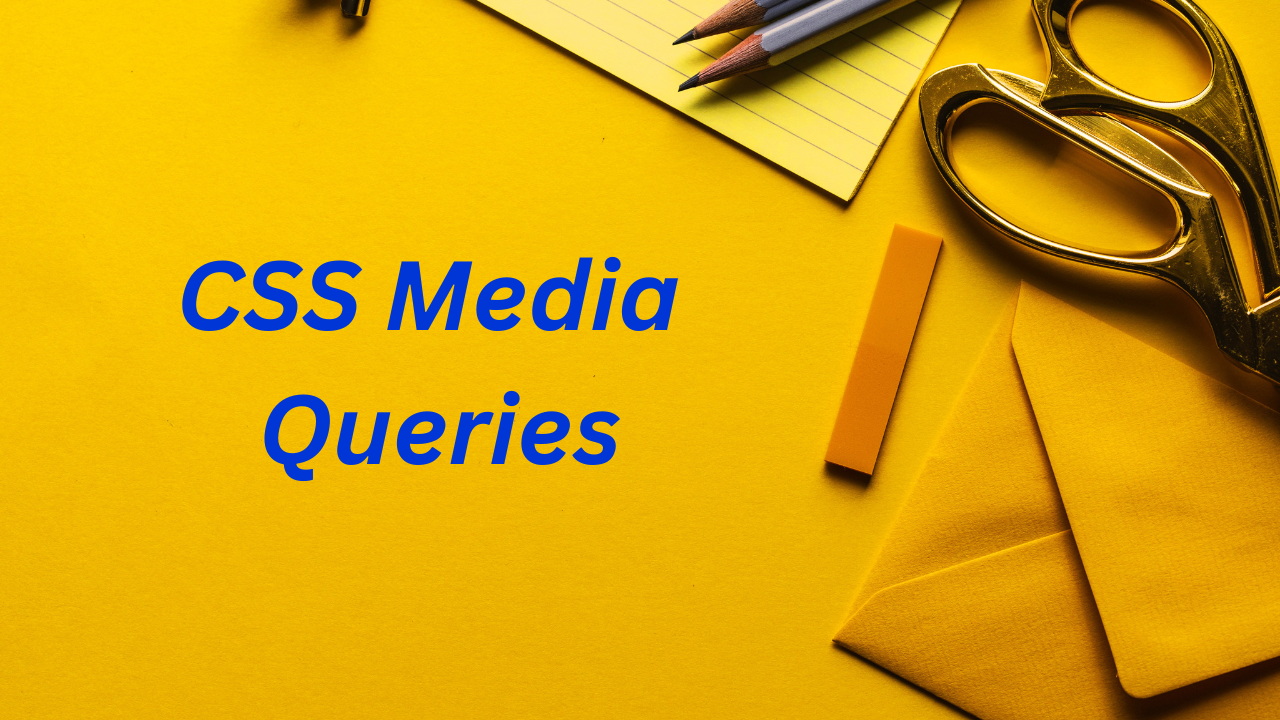
CSS Media queries are a powerful tool for web developers to create responsive websites. A media query is a CSS expression that allows a developer to control the display of content based on certain conditions, such as the type of device, the size of the viewport, and the orientation of the device.
A basic media query consists of an @media rule followed by a media type and or media feature expressions. The media type defines what type of device the media query is targeting (e.g. screen or print) while the media feature expressions are used to define additional properties like the width and height of the viewport, the orientation of the device, and other related conditions.
Syntax Media Query
A media query is made up of one or more media feature expressions, an optional media type, and logical operators that may be used to combine the expressions in different ways. In media questions, case is irrelevant.
@media [media-type] ([media-feature]) {
/* Styles */
}
For example, if we wanted to target all Apple devices, we could use the following media query:
@media only screen and (min-device-width: 667px) and (max-device-width: 812px) {
/* Styles here will be applied to all Apple devices */
}
Media Types
Media categories, such as all, print, and screen, specify the broad category of the device to which the media query is applicable. With the exception of when employing the not or only logical operators, the type is optional (it is presumed to be all).
Screen
The screen can target specified media queries on screen devices. devices like computers, tablets and mobile phones.
Syntax:
@media screen {
.container{
/* styles */
}
}
Print can target specifies media queries for the printers.
Syntax:
@media print {
.container{
/* styles */
}
}
All
All can target specified media queries on all devices, it is the default type.
Syntax:
@media all {
.container{
/* styles */
}
}
Media Features
We may begin specifying the features we're aiming to match after we've established the kind of material we're attempting to replicate. Expressions that test for media feature presence or value are fully optional. Parentheses are required around each expression used in a media feature.
Viewport / Page Features
width: Defines the widths of the viewport. This can be a specific number (e.g. 400px) or a range (using min-width and max-width). value -
<length>
height: Defines the height of the viewport. This can be a specific number (e.g. 400px) or a range (using min-height and max-height). value -
<length>
aspect-ratio: Defines the width-to-height aspect ratio of the viewport, value -
<ratio>
orientation: The way the screen is oriented, such as tall (portrait) or wide (landscape) based on how the device is rotated. value -
portraitlandscape
overflow-block: Checks how the device treats content that overflows the viewport in the block direction, which can be scroll (allows scrolling), optional-paged (allows scrolling and manual page breaks), paged (broken up into pages), and none (not displayed). value -
scroll
optional-paged
paged
overflow-inline: Checks if the content that overflows the viewport along the inline axis be scrolled, which is either none (no scrolling) or scroll (allows scrolling), value -
scroll
none
Display Quality
resolution: Defines the target pixel density of the device, values -
<resolution> infinite
scan: Defines the scanning process of the device, which is the way the device paints an image onto the screen (where interlace draws odd and even lines alternately, and progressively draws them all in sequence), values -
interlace
progressive
grid: Determines if the device uses a grid (1) or bitmap (0) screen, values-
0 =
Bitmap
1 = Grid
update: Checks how frequently the device can modify the appearance of content (if it can at all), with values including none, slow and fast. values -
slow fast none
environment-blending: A method for determining the external environment of a device, such as dim or excessively bright places. values -
opaque
additive
subtractive
display-mode: Tests the display mode of a device, including fullscreen(no browsers chrome), standalone (a standalone application), minimal-UI (a standalone application, but with some navigation), and browser (a more traditional browser window), values-
fullscreen
standalone
minimal-UI
browser
Color
color: Defines the color support of a device, expressed numerically as bits. So, a value of 12 would be the equivalent of a device that supports 12-bit color, and a value of zero indicates no color support. value-
<integer>
color-index: Defines the number of values the device supports. This can be a specific number (e.g. 10000) or a range (e.g. min-color-index: 10000, max-color-index: 15000), just like width.
monochrome: The number of bits per pixel that a device’s monochrome supports, where zero is no monochrome support.
color-gamut: Defines the range of colors supported by the browser and device, which could be
srgb
,p3
, orrec2020
.dynamic-range: The combination of how much brightness, color depth, and contrast ratio is supported by the video plane of the browser and user device. values -
standard high
inverted-colors: Checks if the browser or operating system is set to invert colors (which can be useful for optimizing accessibility for sight impairments involving color), values -
inverted
none
.
Interaction
pointer: Sort of like any pointer but checks if the primary input mechanism is a pointer and, if so, how accurate it is (where coarse is less accurate, fine is more accurate, and none is no pointer). values -
coarse
fine
none.hover: Sort of like any-hover but checks if the primary input mechanism (e.g. mouse of touch) allows the user to hover over elements. value -
hover
any-pointer: Checks if the device uses a pointer, such as a mouse or styles, as well as how accurate it is (where coarse is less accurate and fine is more accurate). values -
coarse fine
any-hover: Checks if the device is capable of hovering elements, like with a mouse or stylus. In some rare cases, touch devices are capable of hovering.
Scripting
- scripting: Checks, whether the device allows scripting (i.e. JavaScript) where enabled, allows scripting, initial-only enabled.
Device Breakpoints
- Extra small devices (phones, 600px and down)
@media only screen and (max-width: 600px) {...}
- Small devices (portrait tablets and large phones, 600px and up)
@media only screen and (min-width: 600px) {...}
- Medium devices (landscape tablets, 768px and up)
@media only screen and (min-width: 768px) {...}
- Large devices (laptops/desktops, 992px and up)
@media only screen and (min-width: 992px) {...}
- Extra large devices (large laptops and desktops, 1200px and up)
@media only screen and (min-width: 1200px) {...}
Example:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Media Query</title>
<style>
body {
background-color: lightblue;
}
@media only screen and (max-width: 600px) {
body{
background-color: lightcoral;
}
}
@media only screen and (min-width: 600px) {
body{
background-color: lightcyan;
}
}
@media only screen and (min-width: 768px) {
body{
background-color: lightgoldenrodyellow;
}
}
@media screen and (min-width:992px) {
body{
background-color: lightgreen;
}
}
@media screen and (min-width:1200px) {
body{
background-color: lightsalmon;
}
}
</style>
</head>
<body>
</body>
</html>
Subscribe to my newsletter
Read articles from Gaurav Patil directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Gaurav Patil
Gaurav Patil
I am a Full stack developer.