Golang Basics I: Variables, Datatypes & Arithmetic Operations
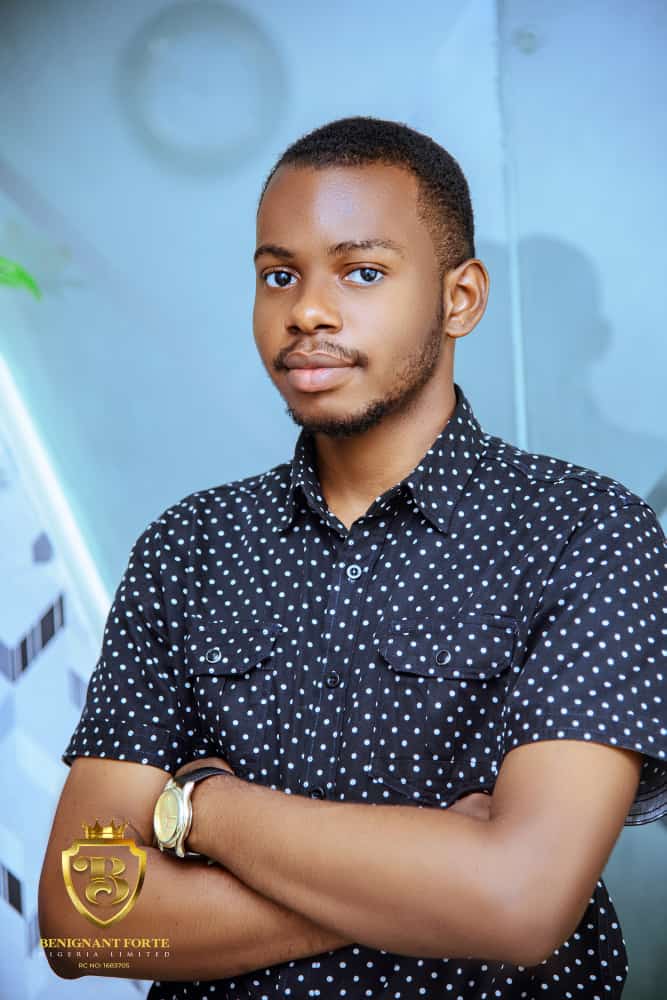
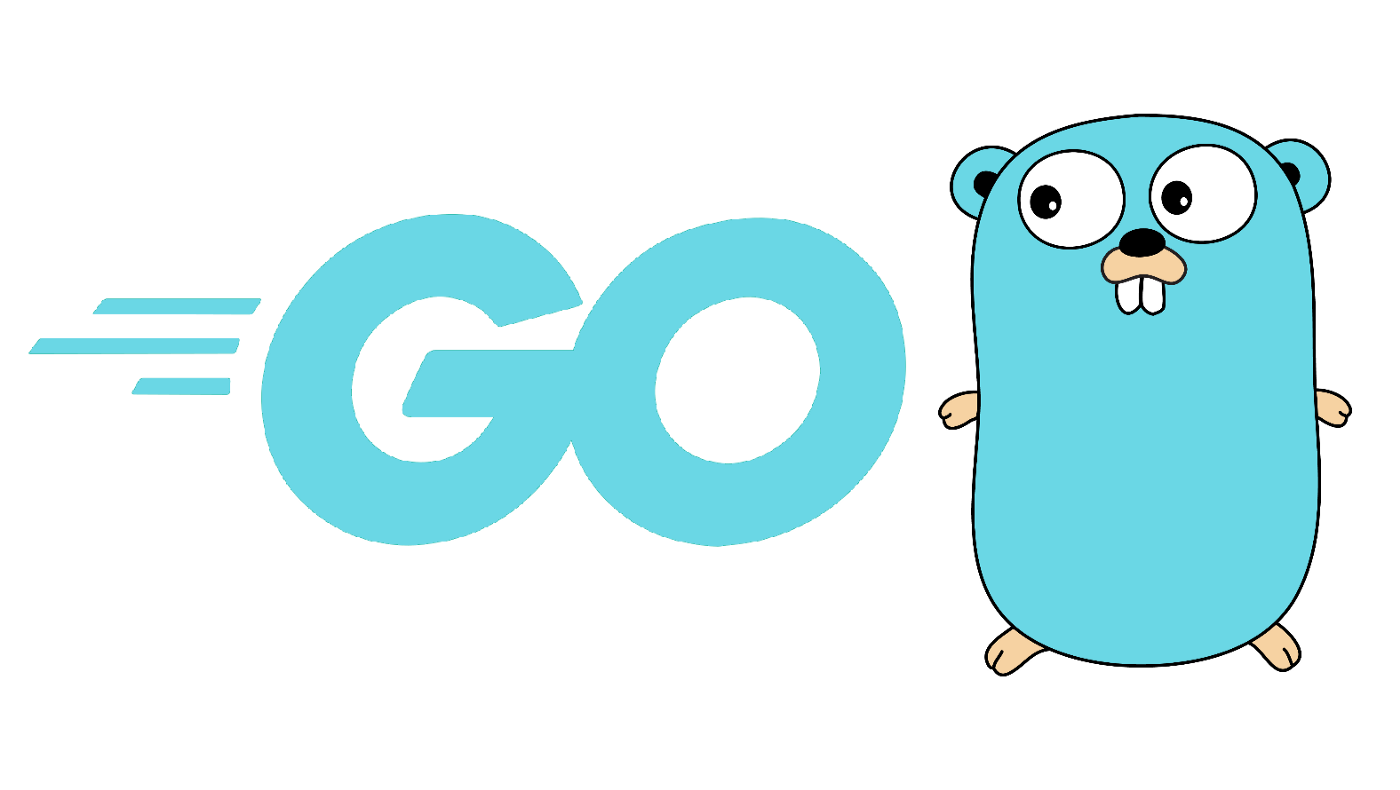
Before you start, you need to approach GO as a newbie and if you're a newbie great!! Also, it is best to practice (do not copy and paste), write the codes yourself no matter how boring or easy it may look, and do the act of testing the code yourself.
You should also have GO installed and know how to run a GO program.
For the first section, we'd be learning about;
Datatypes
Variables
Printing & Strings Formatting
Arithmetic Operations
Below is the structure of GO;
package main
import "fmt"
func main() {
//code comes in here
}
DATA TYPES
In GOLANG the basic datatypes are integers(int), strings, boolean(bool), floats. but as we go on we'll learn about the complex types.
For the integers, They store whole numbers without decimal points. We have the unsigned integers(uint) and signed integers(int).
a). The integers (int) store both positive and negative whole numbers and can be categorized as;
int8 = ranges from -128 to 127
int16 = ranges from -32768 to 32767
int32 = ranges from -2147483648 to 2147483647
int64 = ranges -9223372036854775808 to 9223372036854775807
and leaving it as int, depending on your system it has a default int32 (for 32bits system) or int64(for 64bits system).
The Unsigned Integers store only positive numbers and can be categorized as;
uint8 = ranges from 0 to 255
uint16 = ranges from 0 to 65535
uint32 = ranges from 0 to 4294967295
uint64 = ranges from 0 to 18446744073709551615
and leaving it as uint, depending on your system it has a default uint32(for 32bits system) or uint64(for 64bits system).
For Floats, it stores only numbers with decimal points. it has float32 and float64
For Boolean (bool), it stores either "true" or "false" as its value.
For strings (string), it stores characters, letters or words.
VARIABLES
In GOLANG we declare a variable as;
var variableName datatype = expression. eg
package main import "fmt" func main() { var Name string = "Mike" var Age int = 23 var isLoggedIn bool = false var scores float64 = 34.56 }
You could also declare variables without assigning any values to them as
package main
import "fmt"
func main() {
var name string
var age int
var isLoggedIn bool
var scores float64
//And you can assign values to them as
Name = "Mike"
Age = 25
isLoggedIn = true
scores = 56.03
}
You could also declare multiple variables as;
package main
import "fmt"
func main() {
//var variableName1, variableName2, variableName3 datatype
var age, class, block, level int
var name, book, school string
}
There is a short form of declaring variables is GO which we'll be using a lot which is;
package main
import "fmt"
func main() {
//variableName := expression
name := "Mike"
age := 30
isAlive := true
}
PRINTING &STRINGS FORMATTING
we join variables together using comma (,) when printing. And in printing or displaying codes we use fmt.Println. If you check the code snippets you'll see import "fmt". "fmt" represents a package and we're going to do a lot of packages importing when using GOLANG. so let's try it!!
package main import "fmt" func main() { var name string = "Mike" age := 78 fmt.Println("Welcome!!", name) fmt.Println("Your Age is:", age) }
For Strings Formatting;
package main
import "fmt"
func main() {
var name string = "Mike"
age := 78
fmt.Printf("Welcome %v, your new age is %v\n", name, age)
//notice the use of %v used in place of name and age in the strings
// we also have %T = datatype; you can try it out for the above and see the results.
// %v = variable
// Printf is used when we need to use %v, %T in our print statements.
}
ARITHMETIC OPERATIONS
This refers to math operations we can use while coding to solve certain problems. eg
package main import "fmt" func main() { sum := 3 + 1 minus := 45 -8 divide := 50 / 2 multiply := 34 * 54 modulo := 10 % 3 // this means the remainder after dividing two numbers equals := 12 == 3 //this is read as 12 is equal to 3, true or false? greater := 9 > 12 // this is read as 9 is greater than 12, true or false? lesser := 4 < 9 // this is read as 4 is less than 9, true or false? greaterEqual := 9 >= 12 // this is read as 9 is greater or equal to 12, true or false? lesserEqual := 4 <= 9 // this is read as 4 is less or equal to 9, true or false? notEqual := 9 != 12 // this is read as 9 is not equal to 12, true or false? fmt.Println(sum,minus,divide,multiply,modulo,equals,greater,lesser,greaterEqual,lesserEqual,notEqual) }
Solve the above yourself, then type them out to see the results.
Subscribe to my newsletter
Read articles from MIchael Akor directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
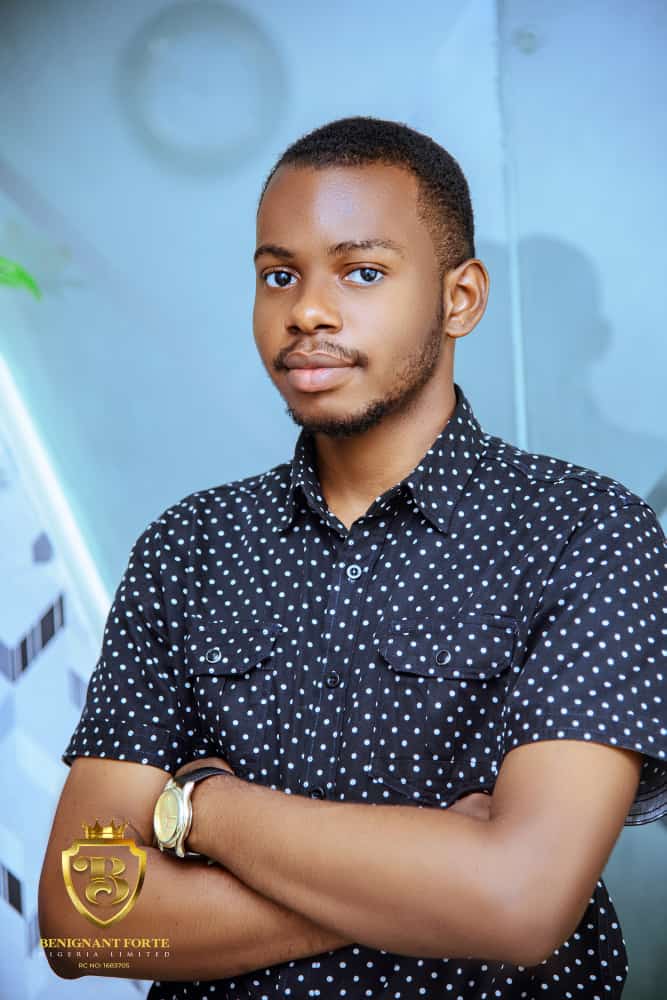