Heart Disease Prediction Model Using Logistic Regression
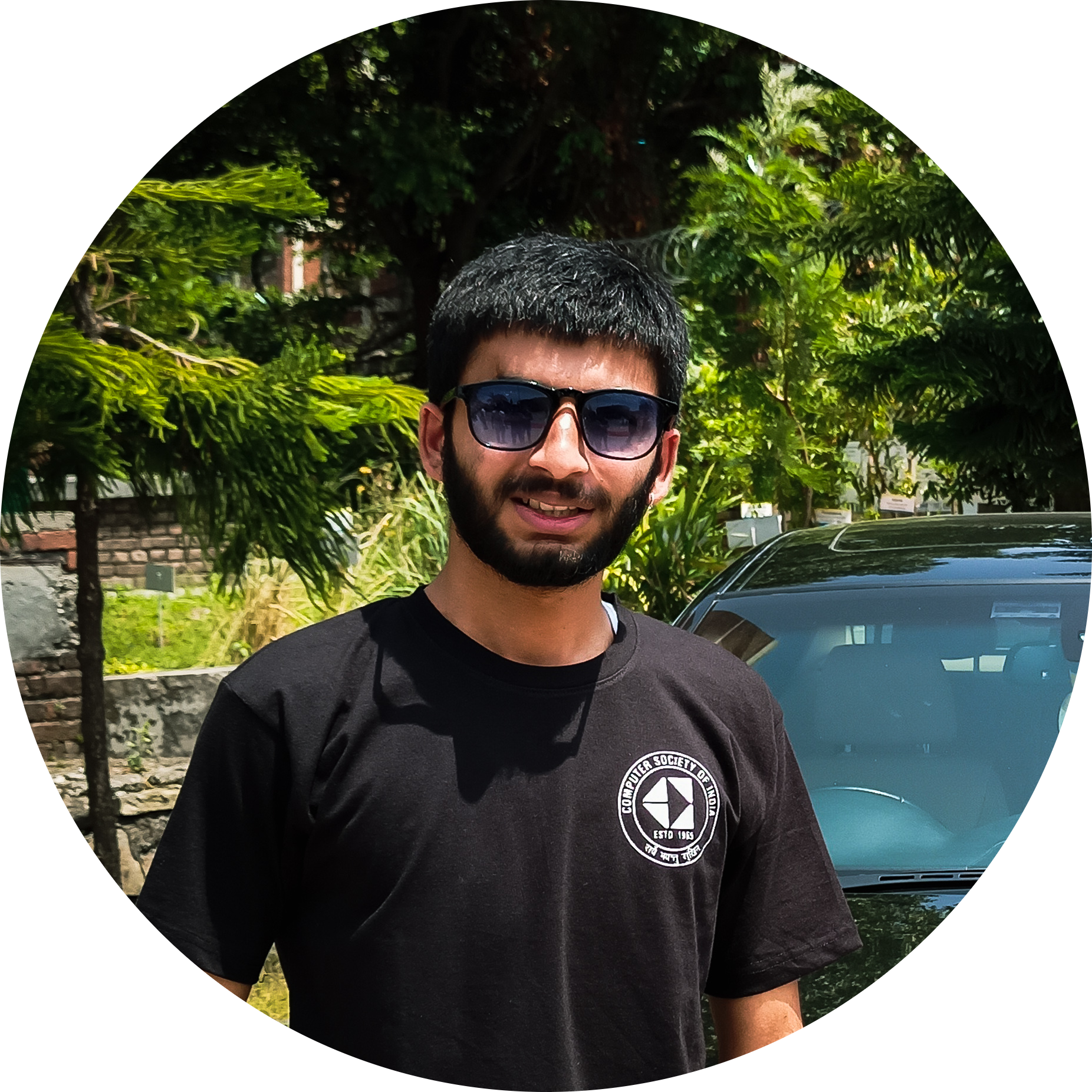

A Heart Disease Prediction System built on machine learning using logistic regression
Principle
This prediction system is based on ECG data on heart diseases of patients
What is ECG ??
An electrocardiogram (ECG) is a quick test that can be used to examine the electrical activity and rhythm of your heart. The electrical signals that your heart beats out each time it beats are picked up by sensors that are affixed to your skin. A machine records these signals, and a doctor examines them to see whether they are odd.
Workflow of model
Data collection
Split Features and Target set
Train-Test split
Model Training
Model Evaluation
Predicting Results
Data collection
Dependencies
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LogisticRegression
from sklearn.metrics import accuracy_score
Split Features and Target set
X = heart_data.drop(columns = 'target', axis = 1)
X.head()
# now X contains table without target column which will help for training the dataset
Train Test Split
X_train, X_test, Y_train, Y_test = train_test_split(X, Y, test_size = 0.15, stratify = Y, random_state = 3 )
- here we have a test data size is 20 percent of the total data which is evenly distributed with a degree of randomness = 3
Model Training
model = LogisticRegression()
model.fit(X_train.values, Y_train)
Model Evaluation
# accuracy of traning data
# accuracy function measures accuracy between two values,or columns
X_train_prediction = model.predict(X_train.values)
training_data_accuracy = accuracy_score(X_train_prediction, Y_train)
print("The accuracy of training data : ", training_data_accuracy)
# accuracy of test data
X_test_prediction = model.predict(X_test.values)
test_data_accuracy = accuracy_score(X_test_prediction, Y_test)
print("The accuracy of test data : ", test_data_accuracy)
Predicting Results
Steps :
take input data
Process the data, change it into an array
reshape data as a single element in the array
predict output using the predict function
output the value
# input feature values
input_data = (42,1,0,136,315,0,1,125,1,1.8,1,0,1)
# change the input data into numpy array
input_data_as_numpy_array = np.array(input_data)
# reshape the array to predict data for only one instance
reshaped_array = input_data_as_numpy_array.reshape(1,-1)
Printing Results
# predicting the result and printing it
prediction = model.predict(reshaped_array)
print(prediction)
if(prediction[0] == 0):
print("Patient has a healthy heart ๐๐๐๐")
else:
print("Patient has a unhealthy heart ๐๐๐๐")
Notations of predicted output:
[0] : means the patient has a healthy heart ๐๐๐๐
[1] : means the patient has an unhealthy heart ๐๐๐๐
Steps for using this system:
Download the repository and find `Heart_Disease_Prediction_using_Machine_Learning.ipynb` file.
Open the file in jupyter Notebook or vscode if the user has jupyter extension.
Run every cell above predicting steps.
give input of your choice for prediction, and make sure the number of inputs must map the features present in the model.
Run the last two cells and if the data is correct and valid to model it will predict the output with an accuracy
Repository link
Contributor: Ankit Nainwal
Please โญโญโญ.
Subscribe to my newsletter
Read articles from Ankit Nainwal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
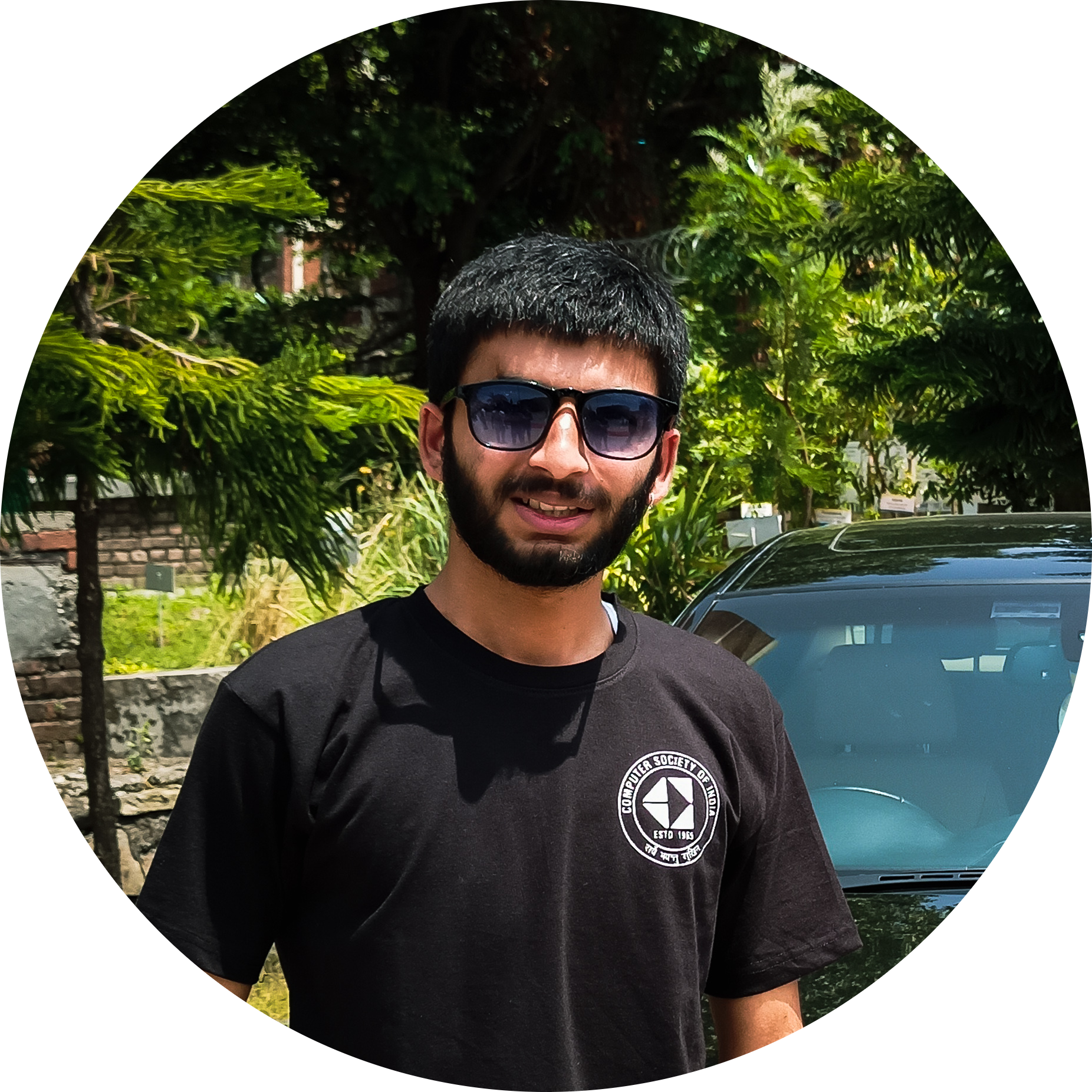
Ankit Nainwal
Ankit Nainwal
CSE Undergrade, with specialization in Aritficial Intellilgence and Machine Learning. From Dehradun, UK, INDIA