Day 1+2 : Building an Algorithm Visualizer in C++
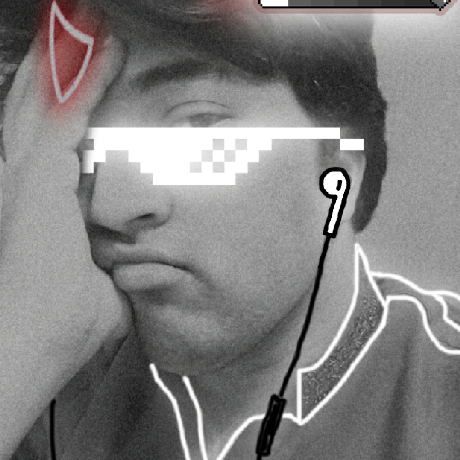
Table of contents
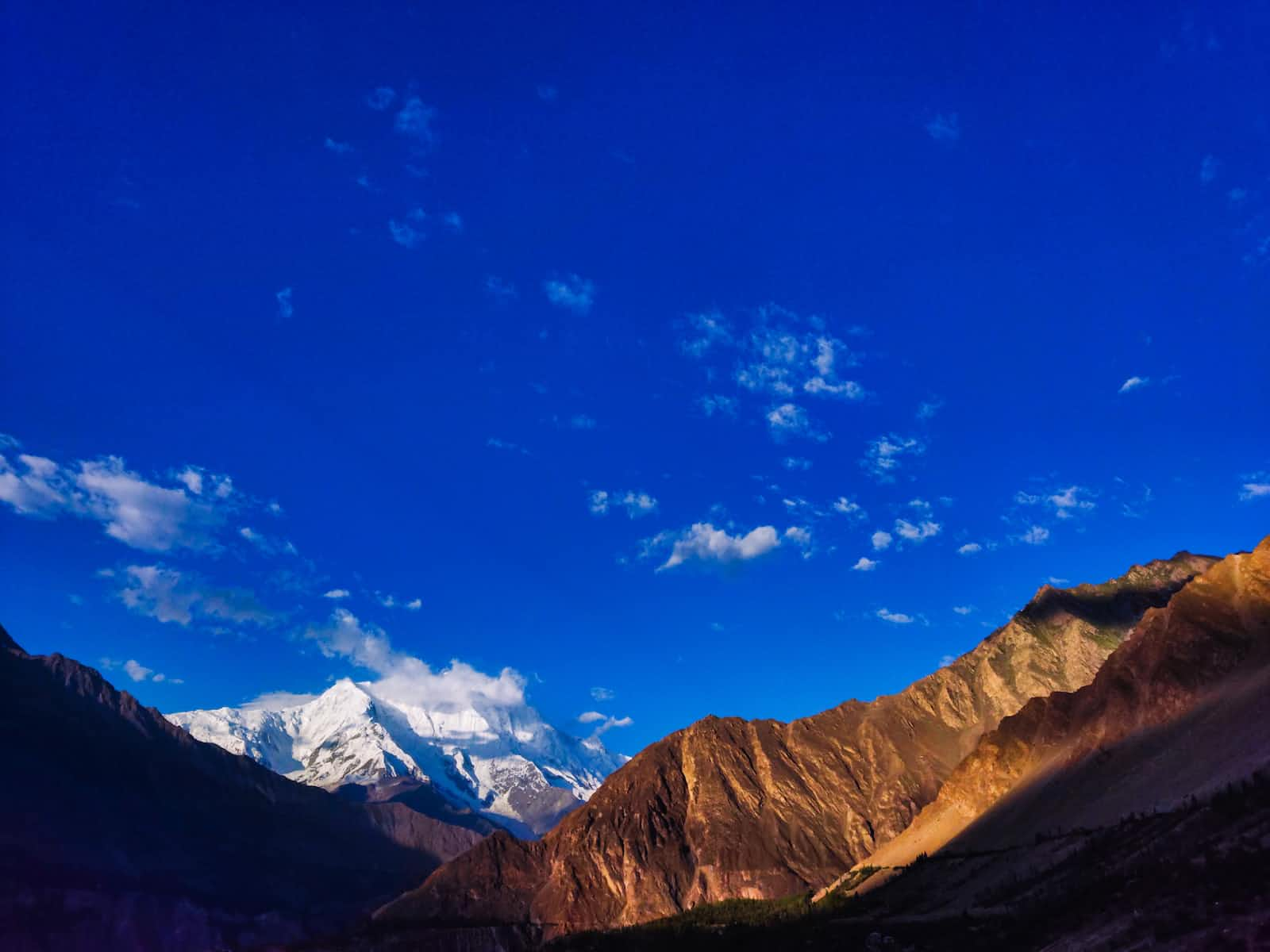
This Blogpost is a compile of 2 days , after the initial setup of the library and generating a screen.
This one :
By the way , the Thumbnails are the from my photography archive .
you can view more Here :
As i am writing this post , coding the solution for this section . I can give a review of what i have done . and add code snippets as i progress .
π Link for the code π :
Click here for : Code of Day 1 + 2
The project , now have :
A file structure
A basic GUI
Mouse interactions
FPS (variable) fixed at 60
Close Button
Button to render the Algorithm Window
Button to render the Data Structure Window
π File Strucutre :
I have decided to divide the code , into headers files .
it looks like this for now :
β‘ It has 3 main header files and a source.cpp
audio.h :
This header files contains the code that , loads the audio files from the assets folder and assigns it to the variable objects . That are initialzed via the globals.h
Globals.h:
Here , i have declared the variables that are being used . For example the ScreenWidth and ScreenHeight values . Reason for keep these here is to make it easy to edit these values instead of searching it again and again.
menu.h:
This header files , draw the close button , Algorithm and the Data Structure button.
source.cpp
This files , initializes the variables , assign them memory and calls the functions defined in the header files .
Drawing The Shapes:
Drawing basic shape :
There are built-in functions for basic shapes , texts in 2D and 3D . Below is code to draw the shapes that are needed for this specific section of the project (Circles , Rectangles and Lines fo Text)
Circle :
// void DrawCircle(value of X, value of Y, radius , Color of the circle);
DrawCircle(x, y, 30, RED);
Rectangle :
// void DrawRectangle(int posX, int posY, int width, int height, Color);
DrawRectangle(100, 100, 200, 100, BLUE);
Text :
//void DrawText("String here ", int posX, int posY, int fontSize, Color);
DrawText("Hello World", 100, 100, 20, BLACK);
How i Positioned these shapes ?
To position , you can manually give the X and Y values depending on the Screen Resolution that you have opted for. That approach work every time , but if you change the screen resolution values you have to alter these positioning variables too.
What i have done it to do some basic maths , to get the co-ordinates.
Math For the Circle Position :
DrawCircle(global.ScreenWidth - 50,global.ScreenHeight/4 - 150 , 30, RED);
As we want the circle to be displayed that top right corner we have to find the coordinates for it .
The Cartesian Plane starts at the top left corner , with Y axis in the inverted direction from what are used to in the geometry and calculus .
Math For the Rectangle Position :
DrawRectangle1(global.ScreenWidth / 2 - 200, global.ScreenHeight / 2 - 50, 200, 100, BLUE);
DrawRectangle2(global.ScreenWidth / 2 + 100, global.ScreenHeight / 2 - 50, 200, 100, BLUE);
Here the ScreenWidth was 1920 and ScreenHeight was 1080 .
To draw the rectangles in the center , we need the X and Y values that are at the center of the Screen . The screen as we know is a rectangle . So to find the center of the rectangle we Divide the Values of Height and Width by 2 respectively .
( ScreenWidth/2 and ScreenHeight/2 )
this will give us the coordinates ( X , Y ) for the center of the rectangle.
Now we can add or subtract from these ( X and Y ) to get the desired positions . I wont get into too much detail of this step . You can copy my code and i will work just as good .
βDrawing text and Interacting with the shapes :
Drawing Text inside Shapes :
This steps stems from the above two and is super simple .
To draw text , you have to just give the exact same (x,y) values as the shape . In the DrawText( ) function.
For Cirlce :
// drawing the circle
DrawCircle(global.ScreenWidth - 50,global.ScreenHeight/4 - 150 , 30, RED);
//drawing the text inside the circle
DrawText("Close[X]", global.ScreenWidth - 50, global.ScreenHeight / 4 - 150, 20, BLACK);
For Rectangles :
//drawing the first rectangle
DrawRectangle(global.ScreenWidth / 2 - 200, global.ScreenHeight / 2 - 50, 200, 100, BLUE);
// drawing text inside the rectangle
DrawText("Algorithm", global.ScreenWidth / 2 - 110, global.ScreenHeight / 2 - 10, 20, BLACK);
// drawing the second rectangle
DrawRectangle(global.ScreenWidth / 2 + 100, global.ScreenHeight / 2 - 50, 200, 100, BLUE);
// drawing text inside the rectangle
DrawText("Data Structure", global.ScreenWidth / 2 + 120, global.ScreenHeight / 2 - 10, 20, BLACK);
Interacting with the Shapes :
To do this , two built in functions Raylib are used . CheckCollisionPointCircle( ) and CheckCollisionPointRec( )
These Functions , gets the mouse position using the GetMousePosition( ) and then compare that to the ( X , Y ) values of the shape and Returns a Boolean output .
That then triggers the code blocks inside the if-conditional .
For Circle :
//if the mouse is inside the circle, change the color of the circle to green
if (CheckCollisionPointCircle(GetMousePosition(), { global.ScreenWidth - 50,global.ScreenHeight / 4 - 150 }, 30)) {
DrawCircle(global.ScreenWidth - 50, global.ScreenHeight / 4 - 150, 30, GREEN);
//drawing text after it turns green
DrawText("Close[X]", global.ScreenWidth - 80, global.ScreenHeight / 4 - 160, 20, BLACK);
}
OUTPUT :
For Rectangles :
//if the mouse is inside the rectangle, change the color of the rectangle to green
if (CheckCollisionPointRec(GetMousePosition(), { global.ScreenWidth / 2 - 200, global.ScreenHeight / 2 - 50, 200, 100 }))
{
DrawRectangle(global.ScreenWidth / 2 - 200, global.ScreenHeight / 2 - 50, 200, 100, GREEN);
//drawing text after it turns green
DrawText("Algorithm", global.ScreenWidth / 2 - 110, global.ScreenHeight / 2 - 10, 20, BLACK);
}
OUTPUT :
Making the Shapes Clickable :
For this we use a nested if-conditional , that gets the collision point from the above conditional and then using the built-in function IsMouseButtonPress( ) , gives a Boolean output that excutes the code block inside the if-condition.
The IsMouseButtonPress( ) , takes a key-ID .
//if the mouse is clicked, close the window
if (IsMouseButtonPressed(MOUSE_LEFT_BUTTON))
{
CloseWindow();
}
When left mouse button is pressed the code will close the window .
We can give any set of instructions , to be executed . In the next post i will add the Algorithms( ) and DataStructure( ) , classes and then these Mouse Clicks will close the main window and open the new respective windows .
ill catch you up in the next blogpost in this series ...
< link to be added here >
Leave your questions in the comments below πππ
Subscribe to my newsletter
Read articles from Moez directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
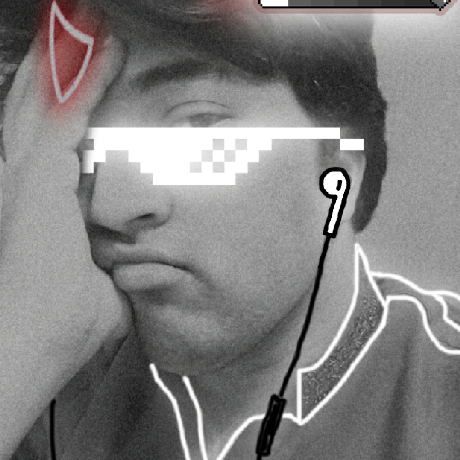
Moez
Moez
Hi , Moeez is the one whose summary you are reading . A STEM major , who is a stoic polymath by practice and Developer/Designer by training and self-learning . For past few years i have been dabbling my way through several skills that have bloomed as side hustles and hobbies (photography and entrepreneurship ) . My main area of expertise are in Development , Design and management. In Development i have Google's Automation certification i work with native c/c++ , gui and all things opensource. Also i have 3years of experience in full-stack frontend including wordpress development . Further i have been leading my tech/e-commerce startup for 2years as CTO. In Design i have Google's Ui/Ux certification . with 3 years of experience my main area of expertise are in minimalist design , illustration and vector animations. Alongside Photoshop manipulation art. I am affluent in all mainstream adobe software products [ Ps,Ai,Pr,Dw,Ae,Id ]. I read , write and compile the abstractions into flexible finality via my yet limited but growing skill-sets.