NodeJS and Multithreading using Worker Threads
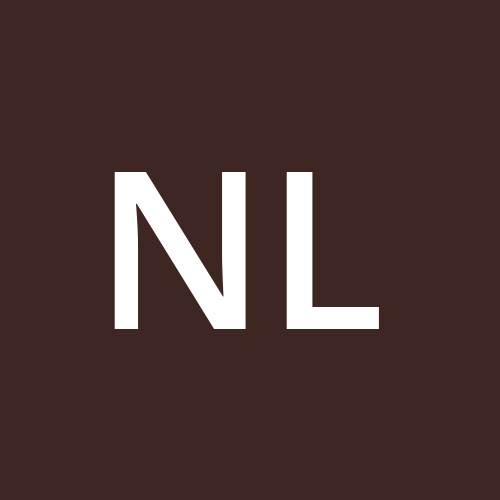
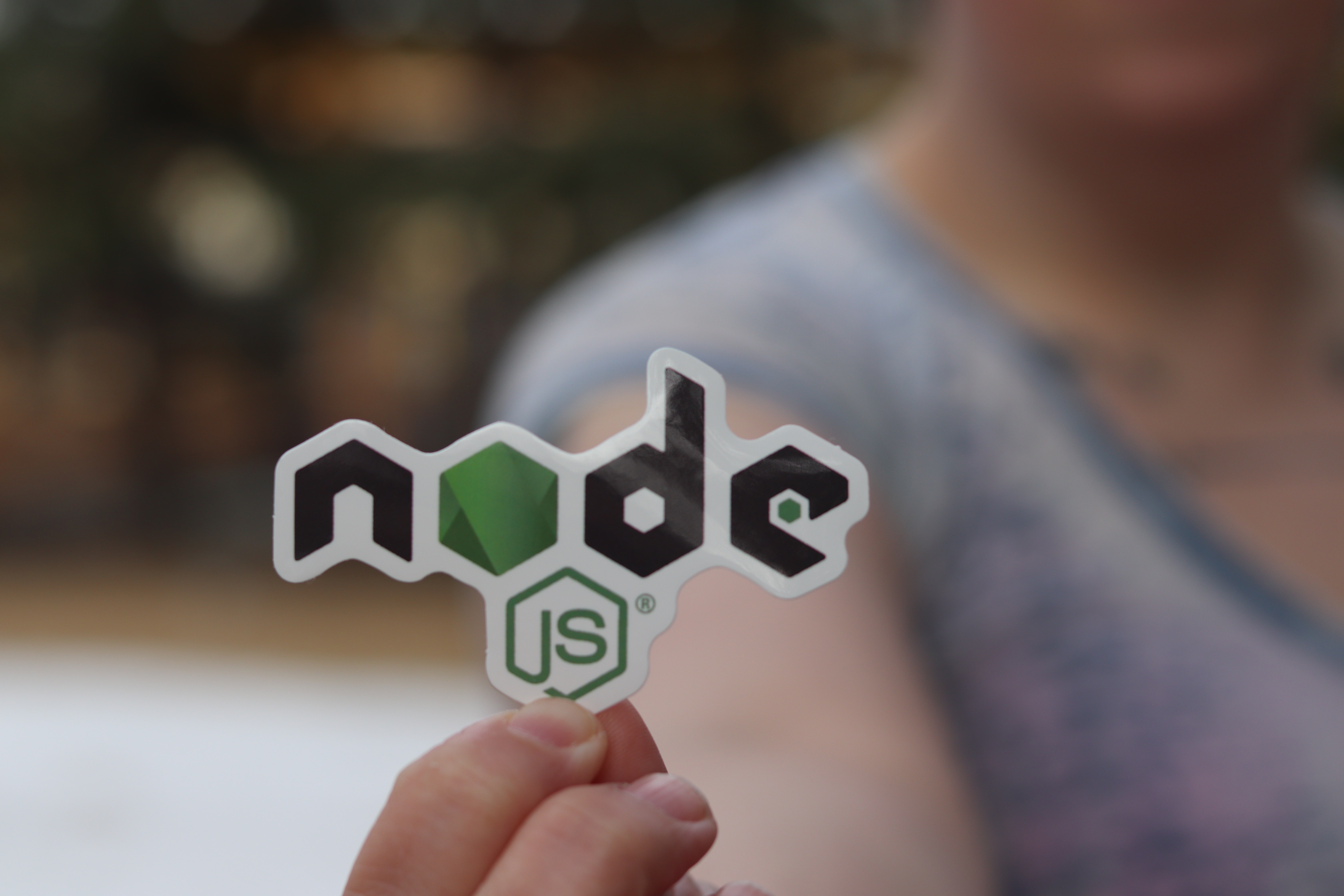
Multithreading in Node.js
Node.js is a JavaScript runtime built on Chrome's V8 JavaScript engine that allows developers to execute JavaScript code on the server side. One of the key features of Node.js is its non-blocking, event-driven architecture, which makes it lightweight and efficient for building scalable network applications. However, this architecture also has some limitations, particularly when it comes to CPU-intensive tasks such as image processing, data analysis, and machine learning.
To overcome these limitations, Node.js provides several mechanisms for implementing multithreading, including:
Child processes
Web Workers
Clustering
In this article, we will explore these three mechanisms and how they can be used to improve the performance of Node.js applications.
Child Processes
The simplest way to create a new thread in Node.js is to use the child_process
module, which allows you to spawn new processes using the spawn()
function. These child processes can be used to execute any system command or script, and they communicate with the parent process using standard input/output streams.
Here is an example of how to spawn a child process in Node.js:
const { spawn } = require('child_process');
const child = spawn('ls', ['-lh', '/usr']);
child.stdout.on('data', (data) => {
console.log(`stdout: ${data}`);
});
child.stderr.on('data', (data) => {
console.error(`stderr: ${data}`);
});
child.on('close', (code) => {
console.log(`child process exited with code ${code}`);
});
This code will spawn a new process that executes the ls
command with the -lh
and /usr
arguments, and it will print the output to the console.
One of the advantages of using child processes is that they can be used to execute any system command or script, not just JavaScript code. This makes them very flexible and powerful. However, they also have some limitations:
Communication between the parent and child processes is based on streams, which can be slow and cumbersome.
Spawning a new process is an expensive operation, and it can take a significant amount of time.
It is difficult to share data between child processes, as they run in separate memory spaces.
Web Workers
Another way to create a new thread in Node.js is to use the Web Workers
API, which allows you to run JavaScript code in a separate thread. Web Workers are supported by modern browsers, and they can be used in Node.js applications using the worker_threads
module.
Here is an example of how to create a Web Worker in Node.js:
const { Worker } = require('worker_threads');
const worker = new Worker('./worker.js');
worker.on('message', (message) => {
console.log(`Message from worker: ${message}`);
});
worker.on('error', (error) => {
console.error(error);
});
worker.on('exit', (code) => {
console.log(`Worker exited with code ${code}`);
});
Conclusion
Multithreading is a powerful technique for improving the performance of Node.js applications, particularly for CPU-intensive tasks. By using child processes, web workers, or clustering, you can create new threads of execution that can run concurrently with the main event loop. Each of these mechanisms has its own benefits and limitations, and the best choice will depend on the specific needs of your application.
As always, it's important to carefully evaluate the trade-offs of each approach and choose the solution that provides the best balance of performance, reliability, and maintainability. By mastering the art of multithreading in Node.js, you'll be well-equipped to build highly scalable and efficient applications that can handle any workload.
If you would like to learn more about it, here's a youtube video I found useful for myself.
Documentation
Worker threads | Node.js v19.4.0 Documentation (nodejs.org)
Child process | Node.js v19.4.0 Documentation (nodejs.org)
Subscribe to my newsletter
Read articles from Nakul Londhe directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
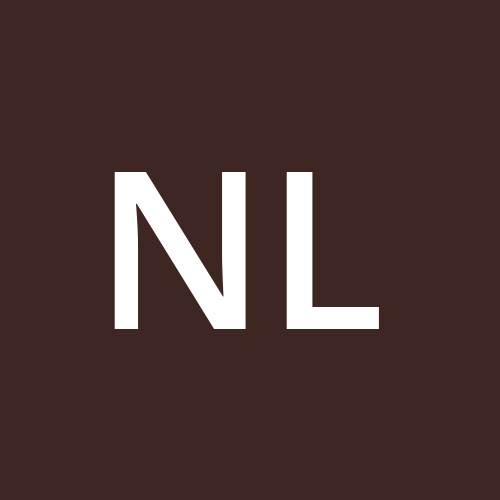