Bash Shell Scripting

Table of contents
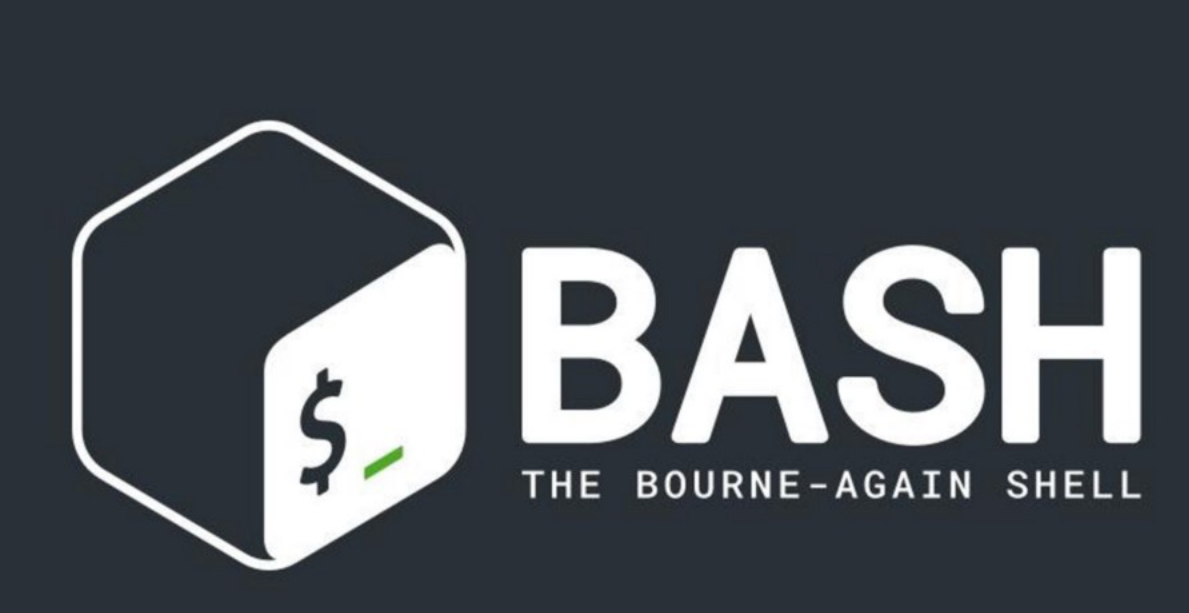
What is shell scripting?
Shell Script is a series of commands written in a plain text file. Shell scripting allows us to use commands we already use at the command line. We are familiar with the interactive mode of the shell. Almost anything can be done in a script which can be done at the command line.
When to write Shell Script?
Repeated Tasks: Necessity is the mother of invention. The first candidates for shell scripts will be manual tasks that are done on a regular basis.
ex: Backups , Log monitoring , Check disk space
Occasional Tasks: Tasks that are performed rarely enough that their method or even their need may be forgotten.
ex: Periodic business related reports(monthly/quarterly/yearly/daily) , Offsite backups, Purging old data
Complex Manual Tasks: Some tasks must be performed manually but may be aided by scripting.
ex: Checking for database locks , Killing runaway processes
Special Tasks: These are tasks that would not be possible without a programming language.
Ex: Storing OS information (performance stats, disk usage, etc.) into the database , High frequency monitoring (several times a day or more)
First Shell Script:
#!/bin/bash
echo "Hello World"
Create a file named helloworld.sh
Add above mentioned command in file.
#!/bin/bash
echo "Hello World"
Give execute permission on helloworld.sh
Execute script using below command
/full/path/script-name
./script-name
bash script-name
How to execute Bash Shell Script?
In the above image, we have executed HelloWorld.sh script.
\==================================================
#!/bin/bash <== This first line indicates what interpreter to use when running this script
The Shebang (#!)
The "shebang" is a special comment. Since it is a comment it will not be executed when the script is run. Instead, before the script is run, the shell calling the script will check for the #! pattern. If found it will invoke the script using that interpreter. f no #! is found most shells will use the current shell to run the script.
Since the shells are installed in different locations on different systems you may have to alter the #! line. For example, the bash shell may be in /bin/bash, /usr/bin/bash or /usr/local/bin/bash.
Setting the shell explicitly like this assures that the script will be run with the same interpreter regardless of who executes it (or what their default shell may be.)
Shell Variable:
The variable is a place holder for storing data. The value of the variable can be changed during the program execution.
ex: var_name=kishor, no=10
[root@ip-172-31-0-141 shellScript]# cat var.sh
#!/bin/bash
no=10
var_name="kishor"
echo $no
echo $var_name
[root@ip-172-31-0-141 shellScript]# ./var.sh
10
kishor
How to access the value of a variable.
Use a $ to access the value of a variable.
How to capture command output in variables?
# Use back quote (`)
[root@ip-172-31-0-141 shellScript]# user=`whoami`
[root@ip-172-31-0-141 shellScript]# echo $user
root
# Use $
[root@ip-172-31-0-141 shellScript]# user=$(whoami)
[root@ip-172-31-0-141 shellScript]# echo $user
root
Variable is not declared:
[root@server1 ~]# echo $b
[root@server1 ~]# b=20
[root@server1 ~]# echo $b
20
In above snap we can varible b is not declared first so its giving us blank line. Below that we assigned 20 value to variable b and then we can see output of echo $b is 20.
Arithmetic operations on variables:
As we know shell variable of type string. We cant perform an arithmetic operation on a string variable. To perform the arithmetic operation we have to use the expr command.
Syntax: expr operand1 operator operand2
addition ---> +
substraction ---> -
mulitplication ---> # must be written with \\ before the *
division ---> /
modules ---> %
Alternative way to perform arithmetic operations.
[root@server1 ~]# no1=10
[root@server1 ~]# no2=20
[root@server1 ~]# echo $((no1 + no2))
30
[root@server1 ~]# echo $((no1 * no2))
200
[root@server1 ~]# echo $((no1 / no2))
0
[root@server1 ~]# echo $((no1 % no2))
10
[root@server1 ~]# echo $((no1 - no2))
-10
System Variables:
PS1 - Command prompt
HOME - your home directory
PWD - your present working directory
LOGNAME - your login name
USER - same as login name
HISTSIZE - number of history commands to be stored in the history file
HOSTNAME - your hostname
SHELL - your login shell
PPID - Process id of the parent process
[root@server1 ~]# echo $HOME
/root
[root@server1 ~]# echo $USER
root
[root@server1 ~]# echo $PWD
/root
[root@server1 ~]# echo $SHELL
/bin/bash
PATH Variable:
[root@server1 ~]# echo $PATH
/usr/local/sbin:/usr/local/bin:/usr/sbin:/usr/bin:/root/bin
[root@server1 ~]# which ls
alias ls='ls --color=auto'
/usr/bin/ls
[root@server1 ~]# which cd
/usr/bin/cd
PATH variable simply hold the path of your system.When we type any command on prompt cheks the executable in path of the system for excution of the command.If it doesn't get the path it displays error as command not found.
[root@server1 ~]# PATH=/usr/local/sbin:/usr/local/bin:/usr/sbin:/root/bin
[root@server1 ~]# echo $PATH
/usr/local/sbin:/usr/local/bin:/usr/sbin:/root/bin
[root@server1 ~]# ls
-bash: ls: command not found
[root@server1 ~]#
A backslash (\): We can continue the long command using a backslash. Below is an example for the same.
tar -cvpzf /share/Recovery/Snapshots/$HOSTNAME_$DATE.tar.gz \
--exclude=/proc \
--exclude=/lost+found \
--exclude=/sys \
--exclude=/mnt \
--exclude=/media \
--exclude=/dev \
--exclude=/share/Archive \
/
$? : To check the exit status of the command
[root@server1 ~]# ls
dead.letter diskspace.sh helloworld.sh
[root@server1 ~]# echo $?
0
[root@server1 ~]# cat file1
cat: file1: No such file or directory
[root@server1 ~]# echo $?
1
In the above command snap we can see ls command is successfully executed hence $? status is 0.
Subscribe to my newsletter
Read articles from Kishor Aswar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
