Exploring the Reflection API in Java

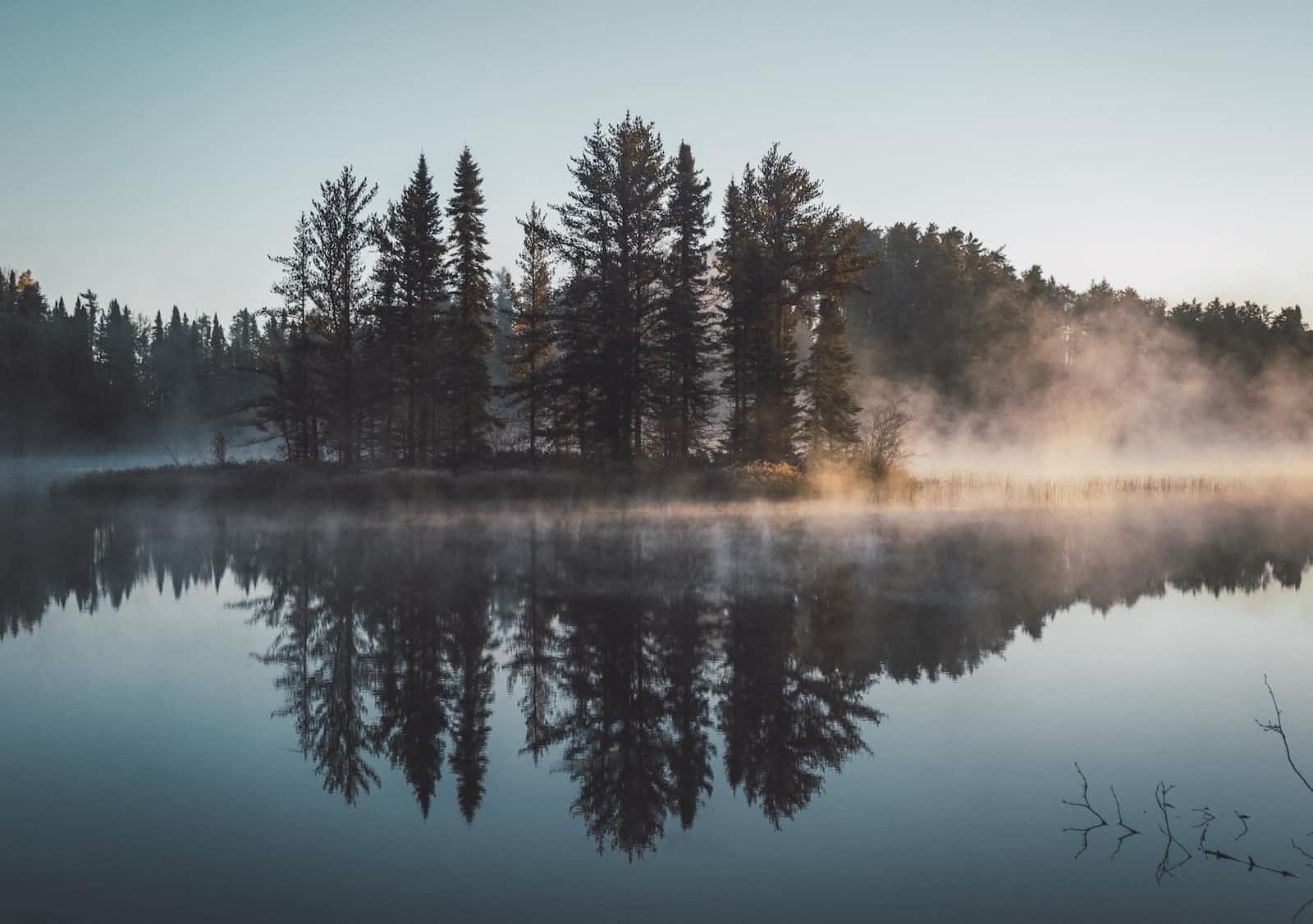
The Java Reflection API is a powerful tool that allows developers to examine and manipulate the runtime behavior of Java code. It enables you to access and modify the attributes of classes, interfaces, fields, and methods at runtime, making it a valuable tool for a wide range of tasks.
In this blog, we'll explore what the Reflection API is, how to use it, and the pros and cons of using it in your Java projects, with examples to illustrate each concept.
What is the Reflection API?
The Reflection API is a set of classes and interfaces in the java.lang.reflect
package that provide information about the structure of Java classes at runtime. With the Reflection API, you can discover the names, types, and annotations of a class's fields and methods, as well as access and modify their values.
Here's an example of using the Reflection API to discover the fields and methods of a class:
Class cls = MyClass.class;
// Get the public fields of the class
Field[] fields = cls.getFields();
for (Field field : fields) {
System.out.println("Field: " + field.getName() + ", Type: " + field.getType().getName());
}
// Get all methods of the class (including private methods)
Method[] methods = cls.getDeclaredMethods();
for (Method method : methods) {
System.out.println("Method: " + method.getName() + ", Return Type: " + method.getReturnType().getName());
}
How to Use the Reflection API
Using the Reflection API is relatively straightforward. To get started, you'll need to obtain a java.lang.Class
object that represents the class you want to examine or modify. There are several ways to do this, including using the getClass()
method of an instance of the class, the .class
syntax, or the forName()
method of the Class
class.
Once you have a Class
object, you can use its various methods to learn about the structure of the class and its members. Some useful methods include getFields()
, getDeclaredFields()
, getMethods()
, getDeclaredMethods()
, getConstructors()
, and getDeclaredConstructors()
. These methods will return arrays of Field
, Method
, and Constructor
objects that you can use to access or modify the values of fields, invoke methods, and create new instances of the class.
Here's an example of using the Reflection API to modify the value of a private field:
Class cls = MyClass.class;
// Get the private field "fieldName" of the class
Field field = cls.getDeclaredField("fieldName");
// Make the field accessible (necessary for private fields)
field.setAccessible(true);
// Get the value of the field
Object fieldValue = field.get(myObject);
// Modify the value of the field
field.set(myObject, "new value");
It's also possible to use the Reflection API to obtain information about the class itself, such as its modifiers (e.g. public, private) and annotations. You can use the getModifiers()
and getAnnotations()
methods of the Class
object to get this information.
Here's an example of using the Reflection API to get the annotations applied to a method:
Class cls = MyClass.class;
// Get the method "myMethod" of the class
Method method = cls.getDeclaredMethod("myMethod");
// Get the annotations applied to the method
Annotation[] annotations = method.getAnnotations();
for (Annotation annotation : annotations) {
System.out.println("Annotation: " + annotation.toString());
}
Pros and Cons of Using the Reflection API
There are several advantages to using the Reflection API in your Java projects. It allows you to write code that is more flexible and adaptable, as you can use it to modify the behavior of existing code at runtime. It can also be useful for testing, as it allows you to access and modify private fields and methods that are not normally accessible.
Here's an example of using the Reflection API to invoke a private method for testing purposes:
Class cls = MyClass.class;
// Get the private method "myPrivateMethod" of the class
Method method = cls.getDeclaredMethod("myPrivateMethod");
// Make the method accessible (necessary for private methods)
method.setAccessible(true);
// Invoke the method
method.invoke(myObject, "arg1", 123);
However, there are also some drawbacks to using the Reflection API. It can have a negative impact on performance, as it operates at runtime and is slower than accessing fields and methods directly. It can also make it easier to write code that is prone to bugs or security vulnerabilities, as it bypasses the access control checks that are enforced by the Java language.
In summary, the Java Reflection API is a powerful tool that can be useful in a variety of situations. It allows you to examine and manipulate the runtime behavior of Java code, but it should be used with caution to avoid negative impacts on performance and security.
Subscribe to my newsletter
Read articles from Rahul Age directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
