Creating a Search Your Github Repo website using React.js.

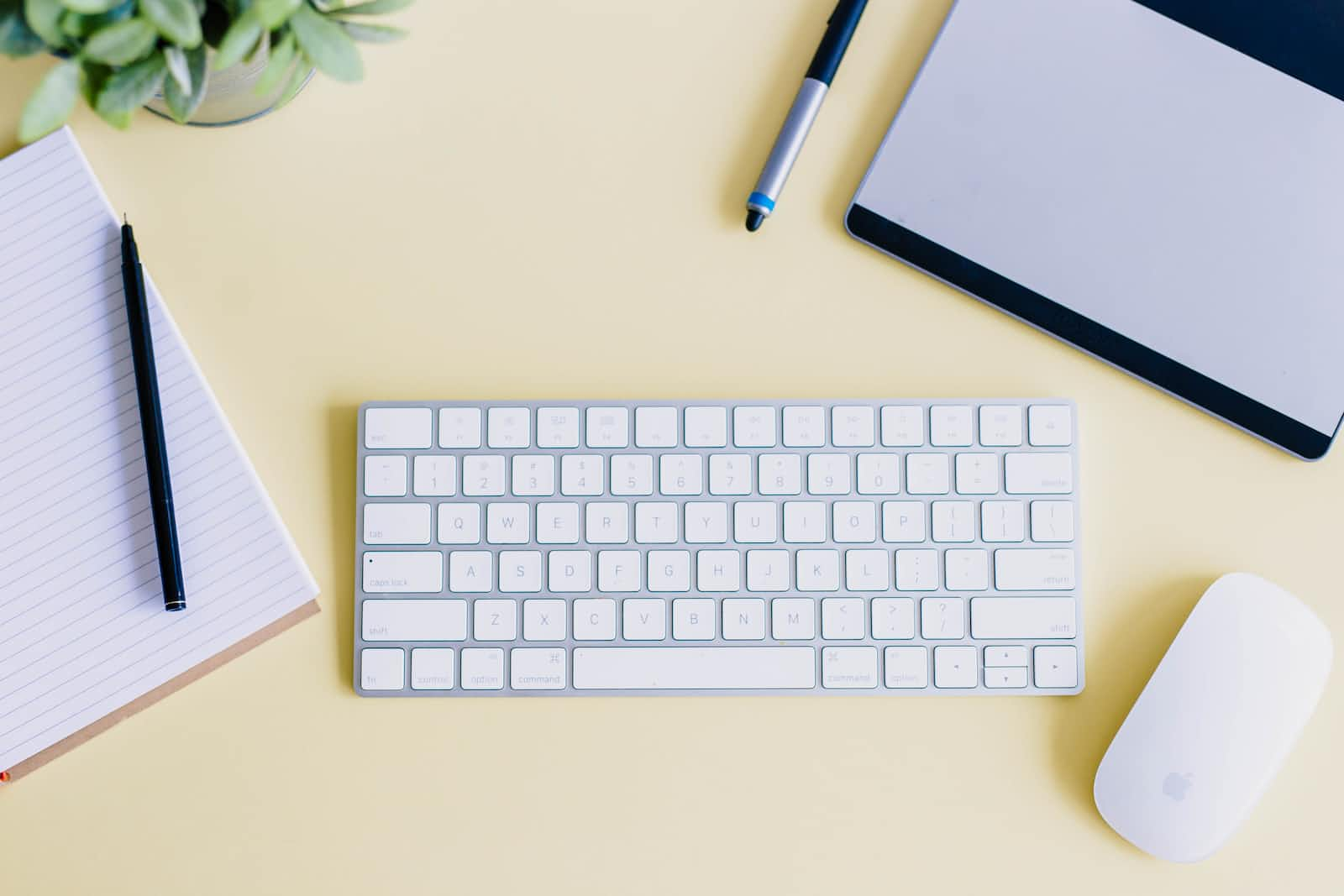
An article with a procedure on how functions were implemented in the necessary project.
Creating the project using React.js, some functions were implemented.
An API fetch of a GitHub Portfolio
Error Boundary
404 Pages
A proper SEO
Starting up the project.
Open up your terminal and run:
npx create-react-app my-app cd my-app npm start
After the react app has been created you can start up with components to be used alongside the necessary route's path to be used.
Implementing the Fetch API for a Github Portfolio.
API fetch would be placed into one of the components (Repos.js) created.
const handleChange = (e) => { setSearch(e.target.value); }; const handleClick = async () => { console.log(search); try { const result = await axios( `https://api.github.com/users/${search}/repos` ); setRepos(result); console.log(result); } catch (error) { console.log(error); } };
This should be implemented with a search bar and a search button, so when a username is being inputted and the "search button" is being clicked it fetches for the user's repository.
Implementing the Error Boundary.
Error boundaries are React components that catch JavaScript errors anywhere in their child component tree, log those errors, and display a fallback UI instead of the component tree that crashed.
The Error boundary component should look like this:
```javascript
import React, { Component } from 'react';
export class ErrorBoundary extends Component {
constructor(props) {
super(props);
this.state = {
hasError: true,
};
}
static getDerivedStateFromError(error) {
return { hasError: false };
}
render() {
if (this.state.hasError)
return (
<div>
<strong>Error!!!</strong> This alert box indicates ErrorBoundary at
work. Kindly ignore the alert box.
</div>
);
return this.props.children;
}
}
export default ErrorBoundary;
```
ErrorBoundary has been implemented successfully.
### Implementing the 404 pages.
A section on the app would be used to showcase an error page when a link attached to it is clicked.
Here's how to call for an error in the app:
```javascript
import React from 'react'
import { useNavigate } from "react-router-dom";
function PageError() {
const navigate = useNavigate();
const ReturnHome = () => {
navigate("/");
};
return (
<>
<h1>ERROR PAGE</h1>
<h2>Page not found</h2>
</>
);
}
export default PageError;
```
You have your Error page displayed!!!
### Implementing a proper SEO for the app.
SEO stands for ***search engine optimization***, which helps your page rank higher on Google and other search engines to drive more traffic to your site.
SEO should be done before deploying the app to an active server.
To start up, react-helmet-async needs to be installed through your terminal
```plaintext
npm i react-helmet-async
```
After installing it, the <App /> in the index.js file should be wrapped in a <HelmetProvider> tag.
```javascript
import React from 'react';
import ReactDOM from 'react-dom/client';
import './index.css';
import App from './App';
import reportWebVitals from './reportWebVitals';
import { HelmetProvider } from 'react-helmet-async';
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(
<React.StrictMode>
<HelmetProvider>
<App />
</HelmetProvider>
</React.StrictMode>
);
reportWebVitals();
```
Next, you add (data-rh) in the <meta> tag:
```xml
<meta
name="description"
content="Web site created using create-react-app"
data-rh="true"
/>
```
After this, you configure the <Helmet> tag in the Homepage component including the webpage title, meta and link:
```javascript
import React from "react";
import { Helmet } from "react-helmet-async";
import { Link } from "react-router-dom";
import SearchBar from "./SearchBar";
function Home() {
return (
<>
<Helmet>
<title>Github User Repository Search ReactApp</title>
<meta name="description" content="Search for a Github User Repository using the search bar." />
<link rel="canonical" href="/.." />
</Helmet>
<nav class="navbar navbar-expand-sm bg-dark navbar-dark">
<ul class="navbar-nav">
<li class="nav-item active">
<Link class="nav-link" to="..">
Home
</Link>
</li>
<li class="nav-item">
<Link class="nav-link" to="/MyRepos">
MyRepos
</Link>
</li>
<li class="nav-item">
<Link class="nav-link" to="/ErrorPage">
ERRORPAGES
</Link>
</li>
<li class="nav-item">
<Link class="nav-link " to="/ErrorB">
ErrorB
</Link>
</li>
</ul>
</nav>
<SearchBar />
</>
);
}
export default Home;
```
After this, a sitemap.txt file will be created:
```plaintext
https://portfolio-rowa.netlify.app/
```
The sitemap.txt file should contain the web page's link, to enable Google to crawl through it.
Lastly, the site can be verified through
[Google Search Console](https://search.google.com/search-console/about).
This should help with enhancing searches made relating to your webpage.
With these steps, your site should be up and running with a good SEO after being deployed.
Subscribe to my newsletter
Read articles from Tomiwa Aborowa directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
