Golang Basics II: If/Else Statements and Arrays
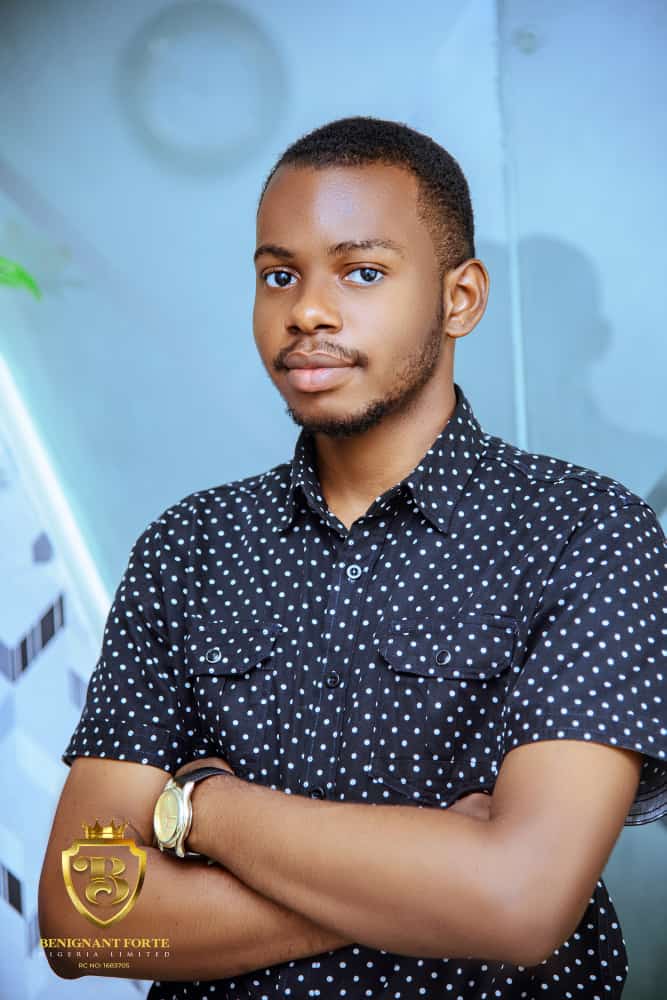
Like in the previous section, I drop the snippets, and we code along and run.
package main
import "fmt"
func main() {
if condition {
expression...
} else {
expression...
}
}
Straight to If/Else statements. These are conditional statements used to manage different possible outcomes of a solution. for example;
If Ade is 18 years old and above let the car door open, Else set the car Alarm on.
This can be represented in codes like this;
package main
import "fmt"
func main() {
ade := 12
if ade >= 18 {
fmt.Println("Car is Open")
} else {
fmt.Println("Alert!! Intruder! Intruder!")
}
}
There's also a case of "Else if " when there are many possible expected results. eg.
If Chima is 10 years old let him get a toy car as a birthday gift, Else if Chima is 16 years old let him get a phone as a gift, Else if Chima is 22years old let him get a car as a gift, Else he doesn't get a gift because his age doesn't fall to any of the gift categories.
package main
import "fmt"
func main() {
ade := 12
if chima == 10 {
fmt.Println("Happy Birthday Chima, you get a toy Car as gift")
} else if chima == 16{
fmt.Println("Happy Birthday Chima, you get a Phone as gift")
} else if chima == 18 {
fmt.Println("Happy Birthday Chima, you get a Car as gift")
} else {
fmt.Println("You don't fall into any the age category")
}
Lastly, you could also do multiple condtions using "AND (&&) " or "OR ( || ) ". eg
package main
import "fmt"
func main() {
bami := 15
//if Bami is equal to 12 and Bami is equal to 15
if bami == 18 && bami == 15 {
// Both conditions MUST be true for the below to be printed
fmt.Println("You get a Phone and a Car as gift")
// else if Bami is equal to 15 or Bami is equal to 18
} else if bami == 15 || bami == 18{
// One condition MUST be met for the below to be printed
fmt.Println("You get a Car as gift")
}
}
What do you think would be printed? Type the code and run it to see if you're correct.
ARRAYS
an array is a collection of items or elements that are of the same type.
var arrayName datatype = arrayDataType {array Expression}. hope that didn't confuse you?
package main
import "fmt"
func main() {
var names []string = [4]string {"Ade", "Chima", "Bunmi", "Ene"}
var ages []int = [3]int {12, 23, 43}
//or
names := [4]string {"Ade", "Chima", "Bunmi", "Ene"} // the 4 represents number of items to be contained in that array
ages := [3]int {12, 23, 43} // the 3 represents number of items to be contained in that array
}
Using their index you could access an item. eg
package main
import "fmt"
func main() {
0 1 2 3
names := [4]string {"Ade", "Chima", "Bunmi", "Ene"}
fmt.Println("The third item is: ", names[2])
fmt.Println("The first item is: ", names[0])
// To replace an item you do;
names[3] = "Mariam" //replacing the fourth item
fmt.Println(names) // print and see the result
}
You could also access more than one item in an array at the same time. eg
package main
import "fmt"
func main() {
0 1 2 3
names := [4]string {"Ade", "Chima", "Bunmi", "Ene"}
neededNames1 := names[0:] //from index 0 to the end
neededNames2 := names[0:2] //from index 0 to the item before index 2
neededNames3 := names[1:3] //from index 1 to the item before index 3
neededNames4 := names[:2] //from the beginning to the item before index 2
//you could guess the answers before checking the solutions
// With this examples you should be able to navigate how to access different elements in an array
}
Subscribe to my newsletter
Read articles from MIchael Akor directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
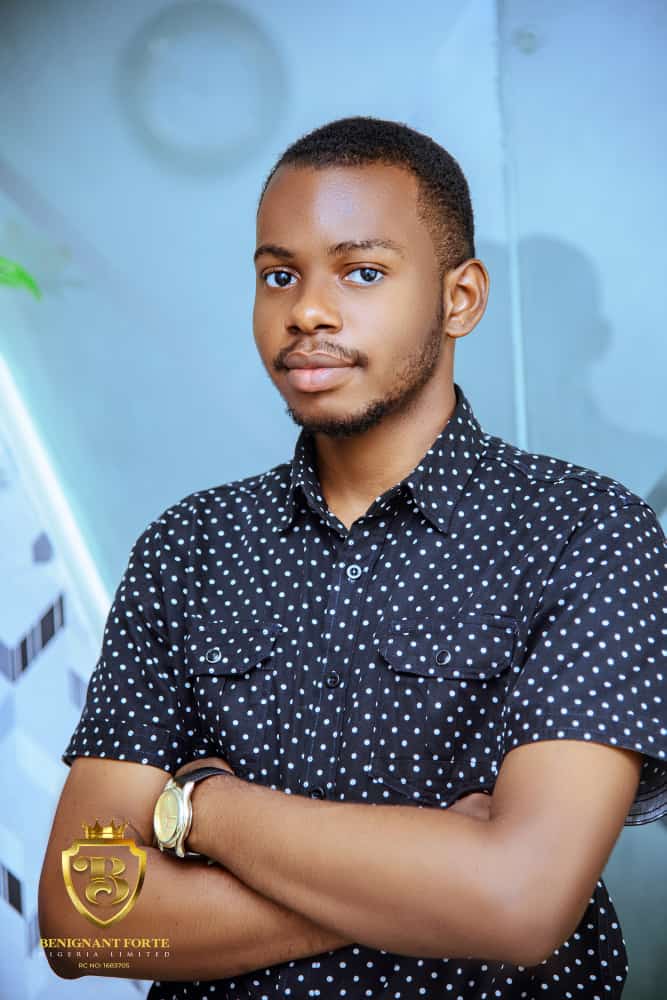