Find All Anagrams in a String
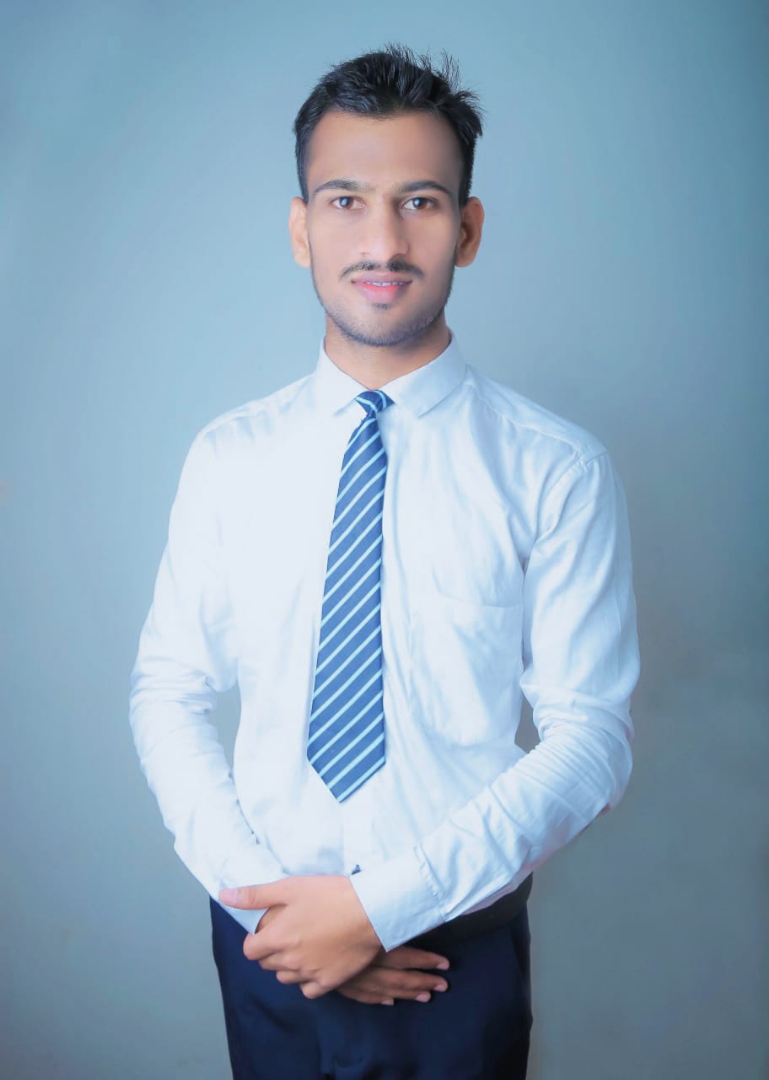
2 min read
Table of contents
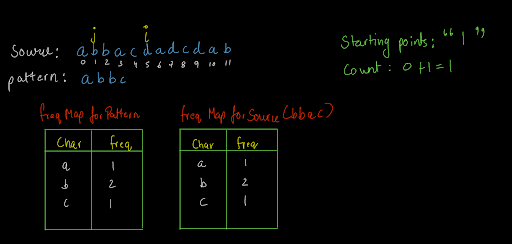
Given two strings s
and p
, return an array of all the start indices of p
's anagrams in s
. You may return the answer in any order*.*
An Anagram is a word or phrase formed by rearranging the letters of a different word or phrase, typically using all the original letters exactly once.
Example 1:
Input: s = "cbaebabacd", p = "abc"
Output: [0,6]
Explanation:
The substring with start index = 0 is "cba", which is an anagram of "abc".
The substring with start index = 6 is "bac", which is an anagram of "abc".
Example 2:
Input: s = "abab", p = "ab"
Output: [0,1,2]
Explanation:
The substring with start index = 0 is "ab", which is an anagram of "ab".
The substring with start index = 1 is "ba", which is an anagram of "ab".
The substring with start index = 2 is "ab", which is an anagram of "ab".
Constraints:
1 <= s.length, p.length <= 3 * 10<sup>4</sup>
s
andp
consist of lowercase English letters.
Solution:
Language: Java
class Solution
{
public List<Integer> findAnagrams(String s, String p)
{
List<Integer> res = new ArrayList<>();
if (p.length() > s.length())
{
return Collections.emptyList();
}
for (int i = 0; i < s.length()-p.length()+1; i++)
{
if (checkArray(s.substring(i, i + p.length()), p))
{
res.add(i);
}
}
return res;
}
public static boolean checkArray(String s, String t)
{
int[] chars = new int[26];
for (char current : s.toCharArray())
{
int z = current - 'a';
chars[z]++;
}
for (char current : t.toCharArray())
{
chars[current - 'a']--;
}
for (int current : chars)
{
if (current != 0)
{
return false;
}
}
return true;
}
}
0
Subscribe to my newsletter
Read articles from Navnath Jadhav directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
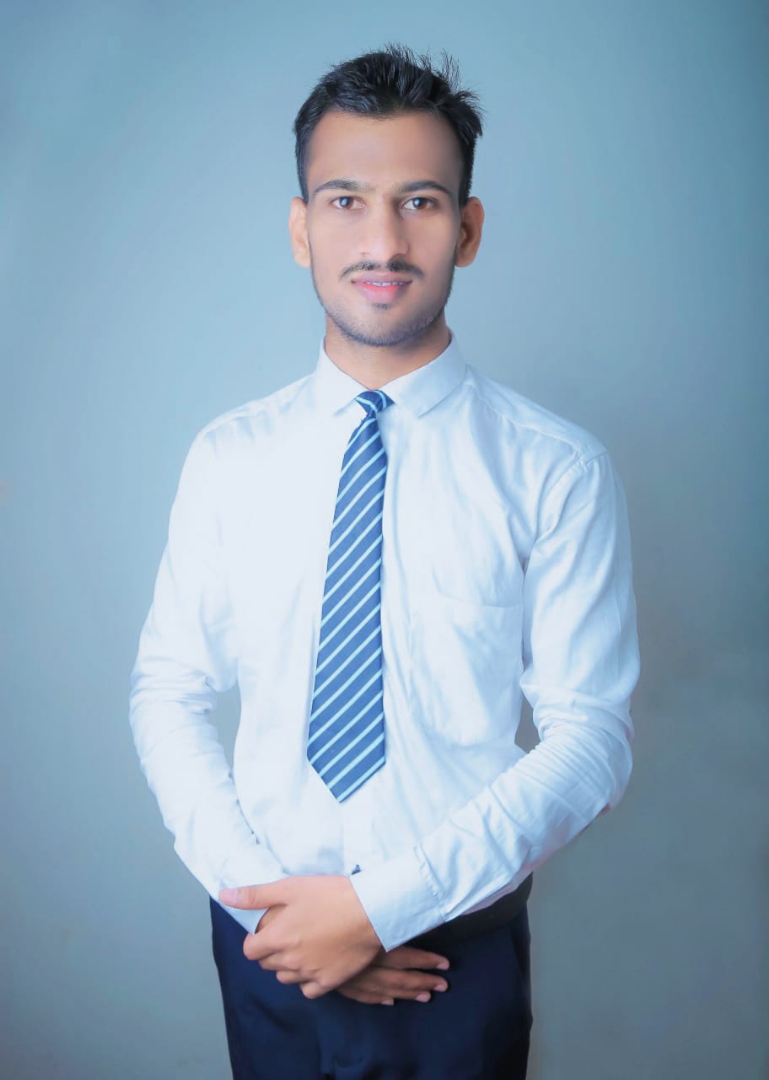
Navnath Jadhav
Navnath Jadhav
I am a Software Developer