Prototype and Prototype Chaining

Table of contents
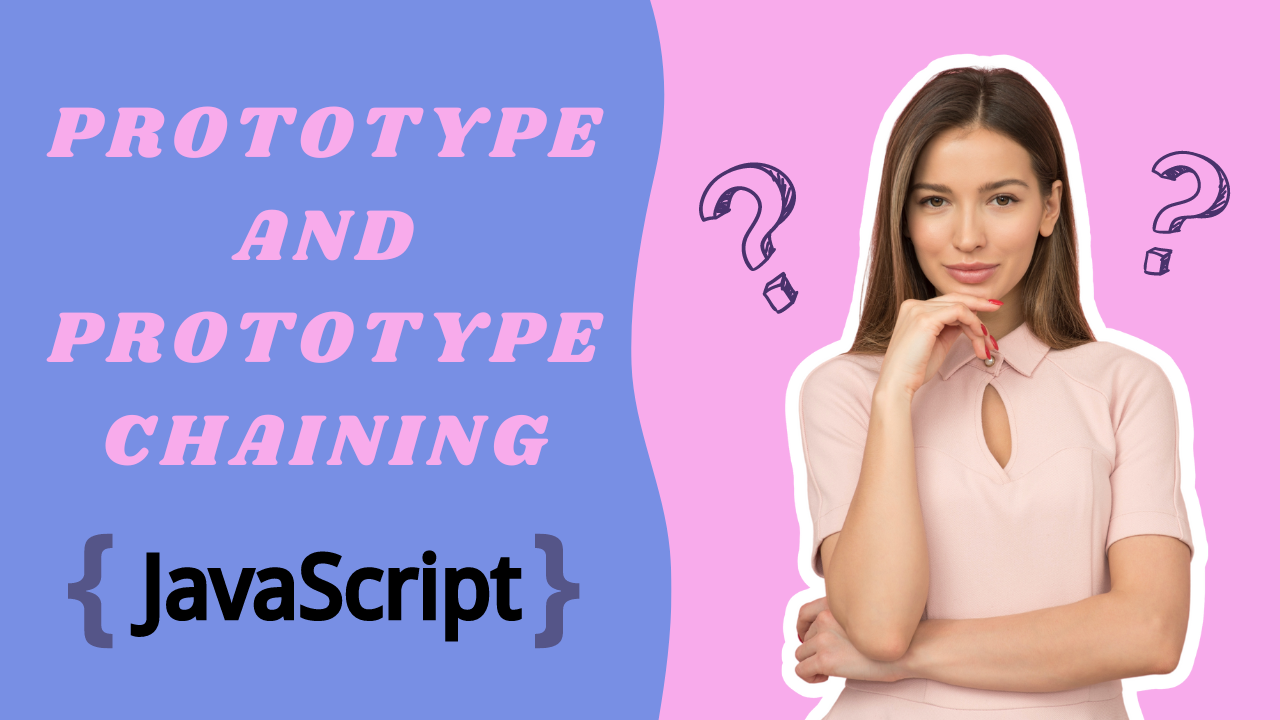
Introduction
Every object in JavaScript has certain pre-defined methods, as we have seen. I first saw the term "prototype" in JavaScript while I was using console.log() for an object in the browser console.
What is prototype?
In JavaScript, every function and object has a property named "prototype" by default.
Prototypes are the mechanism by which JavaScript objects inherit features from one another.
Whenever we create an object or function JavaScript engine adds a built-in Prototype property inside the object or function.
All JavaScript objects inherit properties and methods from a prototype.
const hero = {"fruit": "mango", "flower": "rose"}
console.log(hero.__proto__) // this is old method to get the prototype property
console.log(Object.getPrototypeOf(hero)) // we always use this method to access prototype property
In the above picture, You can see the all prototype properties of the object.
What is prototype chaining?
The Prototype of an object would also have a prototype object.
This "chain" goes continues until it reaches the top level of objects which has null prototype objects. This is called prototype chaining.
All objects in JavaScript are instances of Objects.
All the objects in JavaScript, inherit the properties and methods from Object.prototype.
lets see example:
const myHero = ["thor", "spiderman"];
Object.prototype.gaurav= function(){
console.log("gaurav is present in all objects");
}
myHero.gaurav() //gaurav is present in all objects
As you can see in the above picture the prototype of the Array object.
This Array object also contains the Object prototype you can see in the below picture.
You can have an idea now that these exist in the prototype. Indirectly, these were hidden from us that we inherit from Array.prototype.
This is known as prototype chaining because we keep on chaining the prototype, we can also call prototypal inheritance because it inherits properties and methods from another object.
As you can see "gaurav" method is available in this array of objects also.
Conclusion
I hope you like this article please give like and share. If you have any suggestion regarding this article please comment below. Thanks for reading this article. Until then Keep coding with same energy with same intensity. Stay tuned, see you in the next article.
Subscribe to my newsletter
Read articles from Gaurav Patil directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Gaurav Patil
Gaurav Patil
I am a Full stack developer.