Unlocking the Power of Array Methods: A Comprehensive Guide to map, filter, and reduce in JavaScript.
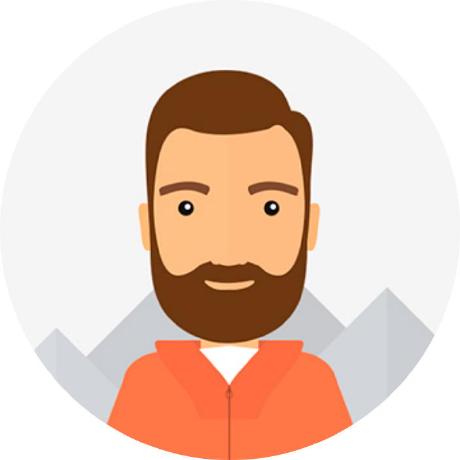
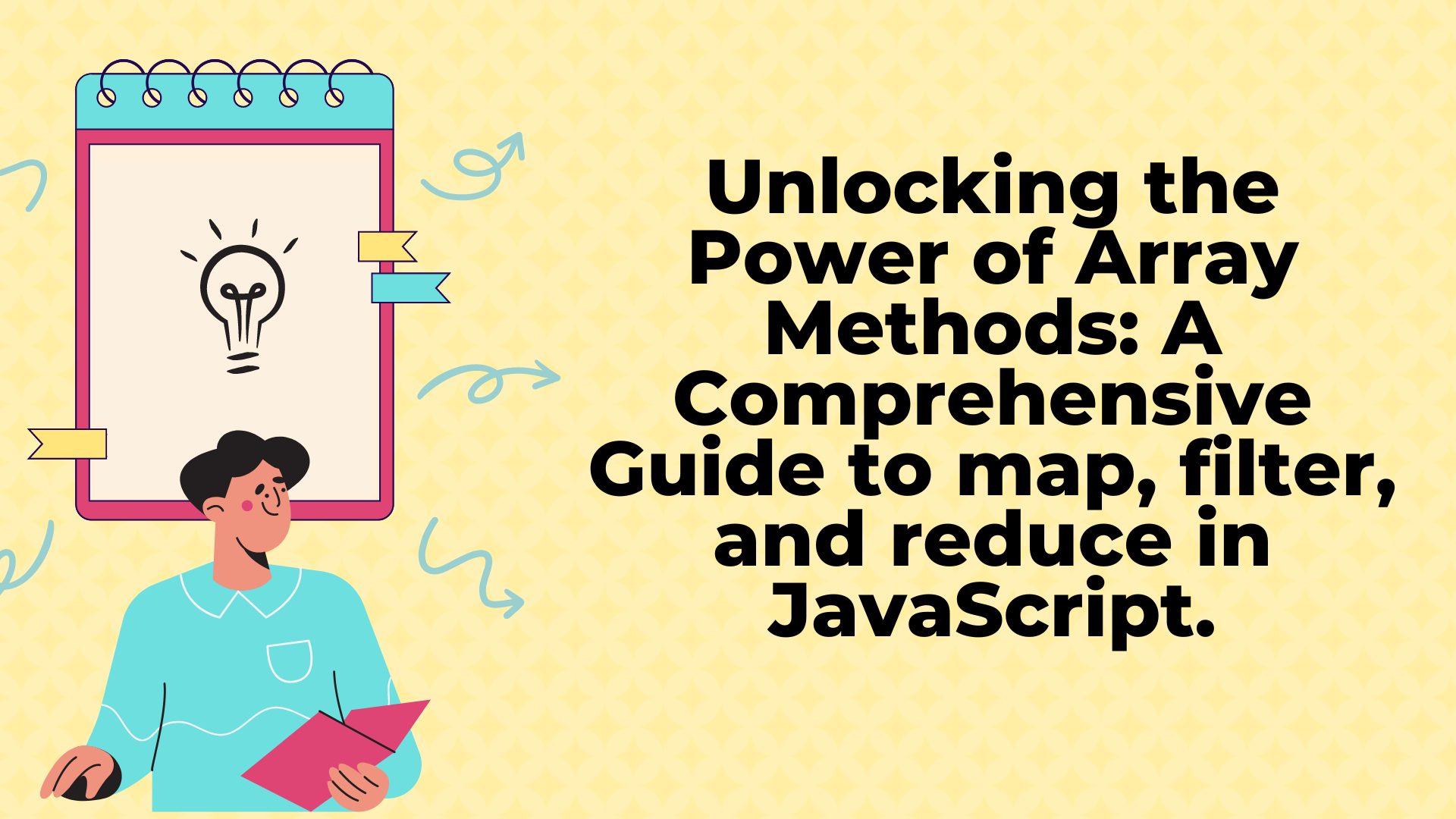
Introduction
JavaScript offers a variety of array methods that can be used to manipulate data in arrays. Three of the most powerful and versatile methods are map, filter, and reduce. These methods can be used to transform and extract data from arrays in a wide range of situations. In this blog post, we will take a comprehensive look at these methods, including how they work, when to use them, and practical examples of how to implement them in your code.
1.Map() Array Method
The map()
function in JavaScript is a way to take an array of items and transform them into a new array of items. It's like a recipe for turning one array into another
Array.map
()
updates each individual value in a given array based on a provided transformation and returns a new array of the same size. It accepts a callback function as an argument, which it uses to apply the transform.
In this example, the map()
the function is applied to the sampleArray
array, and it is set to multiply each number with 2. The map()
the function then returns a new array, modifiedArray
, which contains the numbers multiplied with 2.
Some common use cases of Map() method
Transforming an array of numbers:
The
map()
array method can be used to perform mathematical operations on an array of numbers, such as doubling or halving each element.Let's take an example of tripling an array of numbers-
Extracting properties from an array of objects :
The
map
method can be useful when you need to extract certain information from a set of data and use it in another part of your code.
Let's take an example of extracting the names of persons from array of review objects
const reviews = [
{
id: 1,
name: "susan smith",
job: "web developer",
img:
"https://res.cloudinary.com/diqqf3eq2/image/upload/v1586883334/person-1_rfzshl.jpg",
text:
"I'm baby meggings twee health goth +1. Bicycle rights tumeric chartreuse before they sold out chambray pop-up. Shaman humblebrag pickled coloring book salvia hoodie, cold-pressed four dollar toast everyday carry",
},
{
id: 2,
name: "anna johnson",
job: "web designer",
img:
"https://res.cloudinary.com/diqqf3eq2/image/upload/v1586883409/person-2_np9x5l.jpg",
text:
"Helvetica artisan kinfolk thundercats lumbersexual blue bottle. Disrupt glossier gastropub deep v vice franzen hell of brooklyn twee enamel pin fashion axe.photo booth jean shorts artisan narwhal.",
}
];
Transforming an array of strings: The
map()
method can be used to perform operations on an array of strings, such as converting them to uppercase or lowercase.*** uppercase is not a method of
map()
, it is JavaScript built-in method.****Transforming an array of dates: The
map()
function can also be used to transform an array of dates. For example, let's say you have an array of date strings in the format of "YYYY-MM-DD" and you want to convert them to JavaScript Date objects. You can use themap()
function to accomplish this:Rendering Lists in JavaScript Libraries: Rendering lists in JavaScript libraries is a common task when building web applications. The
map()
function can be used to make this process more efficient and clean.This requires JSX syntax, however, as the
.map()
method is wrapped in JSX syntax.import React from 'react'; function ItemList({ items }) { return ( <ul> {items.map(item => ( <li key={item.id}>{item.name}</li> ))} </ul> ); }
In this example, the
map()
function is applied to theitems
array and it creates a list of<li>
elements, each one containing thename
property of the corresponding item. It's important to note that the key prop is passed to each element, to help React keep track of the elements.Don't worry so much if you are not familiar with react, you can learn it later.
In summary, the map()
function is a powerful and versatile tool in JavaScript that can be used to transform arrays of data in various ways, extract specific properties from an array of objects, convert array-like objects to arrays, create a new array with modified elements, and chain multiple array methods.
2. Filter () Array Method
The filter()
method is used to create a new array that contains only the elements from the original array that pass a certain test. The test is performed by a function that is passed as an argument to the filter method. The function should return true or false, indicating whether the element should be included in the new array.
Let's understand this with some examples:
Let's say you have an array of numbers and you want to filter out all the even numbers. You can use the
filter()
method to accomplish this:
In this example, the filter()
method is applied to the array, and it is set to filter out the even numbers. The filter()
method then returns a new array, evenArray
, which contains only even numbers.
The
filter()
method can also be used to filter out objects in an array based on certain properties. For example, Return only details of those who scored more than 60 marks :let students = [ {name:"piyush", rollNumber: "31", marks:80}, {name:"jenny", rollNumber:"21", marks:34}, {name:"hansh", rollNumber="41", marks:67} ] let filteredStudents = students.filter((student)=>{ return student.marks > 60 }) console.log(filteredStudents)
It's important to note that the filter()
method does not modify the original array, it creates and returns a new array with the filtered elements.
How can you use the
filter()
method to remove duplicate elements from an array?let numbers = [1, 2, 3, 4, 2, 5, 6, 7, 8, 9, 1]; let uniqueNumbers = numbers.filter((num) => { return new Set(numbers).has(num); }); console.log(uniqueNumbers); // [1, 2, 3, 4, 5, 6, 7, 8, 9]
In this example, the
filter()
method is used to iterate through the original array and check if each number is in the set(for those who are not familiar with the set I have explained it below). If it is not, it's added to the set and returned in the new array.
A brief discussion about Set in Javascript
In JavaScript, a Set is a collection of unique values. It can store any type of value, including primitive values (such as numbers and strings) and object references. Sets are similar to arrays, but with a few key differences:
In JavaScript, a Set
is a collection of unique values. It can store any type of value, including primitive values (such as numbers and strings) and object references. Sets are similar to arrays, but with a few key differences:
Sets
only store unique values. If you try to add a value to a Set that already exists, it won't be added again.Sets
are not indexed-based like arrays, so you can't access elements by their position.Sets
provide several useful methods for adding, removing, and checking for the existence of values.
Some use cases of Set in JavaScript:
Removing duplicate values from an array
Checking if an element is in a Set:
let mySet = new Set([98, 23, 33, 114]); console.log(mySet.has(98)); // true console.log(mySet.has(52)); // false
Iterating over the elements in a Set:
let mySet = new Set([1, 2, 3, 4]); for (let value of mySet) { console.log(value); }
In conclusion, the filter()
method is a versatile and useful tool for filtering out elements from an array in JavaScript. It allows you to apply a specific function to each element in an array and returns a new array containing only the elements that pass the test implemented by the function, without modifying the original array.
3. Reduce() Array method
JavaScript's reduce method is a powerful tool for reducing an array of values to a single value. This one is an interesting and tricky one at the same time. Reduce accepts a callback function that consists of an accumulator and the current element of the array. The initial value is used as the starting value of the accumulator. The callback function should return the updated accumulator.
We will understand this method more by doing so that you can get a very clear understanding
SYNTAX :
array.reduce(callback, initialValue)
array
: The array you want to iterate over and reduce.callback
: A function that is called for each element in the array, taking four arguments:accumulator
: A value that accumulates the partial result. or in Essence, the accumulator is like a container or a variable that helps you maintain and update a value as you loop through and process elements in an array.currentValue
: The current element being processed in the array.currentIndex
(optional): The index of the current element being processed.array
(optional): The arrayreduce()
was called upon.initialValue
(optional): An initial value for the accumulator. If provided, thereduce()
function will start accumulating from this value. If not provided, the first element in the array is used as the initial accumulator value, and iteration starts from the second element.
🫡 Imagine you have an array of numbers, and you want to find their sum using reduce()
:
Step 1: Starting Point (Initial Value)
Before you begin adding up the numbers, you need a starting point, just like when you start counting from zero. In programming, we call this starting point an "accumulator." You can think of it as a variable where you'll keep adding the numbers.
In our example, let's set the accumulator to zero since we're starting from scratch:
const numbers = [1, 2, 3, 4, 5];
const initialValue = 0; // Starting from zero
Step 2: The Loop
Now, we'll go through the array one number at a time, like counting numbers on your fingers. We'll start with the first number (1) and follow these steps for each number in the array:
a. First Number (1)
Accumulator: 0 (our starting point)
Current Number: 1 (the first number in the array)
Operation: Add the current number (1) to the accumulator (0).
Result after the first step: 0 + 1 = 1
b. Second Number (2)
Accumulator: 1 (the result from the previous step)
Current Number: 2 (the second number in the array)
Operation: Add the current number (2) to the accumulator (1).
Result after the second step: 1 + 2 = 3
c. Third Number (3)
Accumulator: 3 (the result from the previous step)
Current Number: 3 (the third number in the array)
Operation: Add the current number (3) to the accumulator (3).
Result after the third step: 3 + 3 = 6
d. Fourth Number (4)
Accumulator: 6 (the result from the previous step)
Current Number: 4 (the fourth number in the array)
Operation: Add the current number (4) to the accumulator (6).
Result after the fourth step: 6 + 4 = 10
e. Fifth Number (5)
Accumulator: 10 (the result from the previous step)
Current Number: 5 (the fifth number in the array)
Operation: Add the current number (5) to the accumulator (10).
Result after the fifth step: 10 + 5 = 15
Step 3: The Final Result
After going through all the numbers in the array, you'll end up with the final result stored in the accumulator:
Final result: 15
So, in this example, the reduce()
method starts with an initial value of 0 (our starting point) and then iterates through the array, adding each number to the accumulator. At the end, the accumulator holds the sum of all the numbers, which is 15.
Here's the code to achieve this using reduce()
:
const numbers = [1, 2, 3, 4, 5];
const initialValue = 0;
const sum = numbers.reduce((accumulator, currentValue) => {
return accumulator + currentValue;
}, initialValue);
console.log(`Final result: ${sum}`);
Remember, you can use reduce()
for various tasks, not just adding numbers. You provide a function that specifies how to accumulate the values, and the accumulator keeps track of the result as you loop through the array. It's a versatile tool for working with arrays in JavaScript!
Method Chaining
It's possible to chain the map()
, filter()
and reduce()
methods together in JavaScript to perform multiple operations on an array in a single statement. This is called method chaining.
let's take an example:
let array = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
// you have to filter out the odd numbers, then doduble them and then find the sum of doubled numbers
//1.Filtering out the odd numbers
let oddNumber = array.filter((num) => num % 2 !== 0) // output = [1,3,5,7,9]
//2. Doubling the odd number
.map((num) => num * 2) // output = [2,6,10,14,18]
//3. finding out the sum of doubled numbers using reduce
.reduce((acc, num) => acc + num)
console.log(oddNumber) // output = [50]
Conclusion
In this blog, we have discussed the importance and use cases of the map()
, filter()
, and reduce()
methods in JavaScript. These methods are powerful tools that allow you to perform a variety of operations on arrays, such as transforming elements, filtering elements, and reducing an array to a single value.
We have also seen how to use these methods together, chaining them in a single statement to perform multiple operations on an array. This technique is known as method chaining, and it allows you to write more concise and readable code.
By reading this blog, you should now be able to answer interview-based questions related to these methods, such as:
Given an array of numbers, how would you use the
map()
method to create a new array with each element multiplied by 2?Given an array of strings, how would you use the
filter()
method to create a new array containing only strings that have a length greater than 5?Given an array of objects, how would you use the
reduce()
method to count the number of occurrences of a specific property value?Given an array of numbers, how would you use the
filter()
method to remove all even numbers and create a new array with only odd numbers?Given an array of objects, how would you use the
map()
method to extract an array of a specific property value from each object?Given an array of numbers, how would you use the
reduce()
method to find the largest number?Given an array of objects, how would you use the
filter()
method to create a new array containing only objects that have a specific property value?Given an array of numbers, how would you use the
map()
method to create a new array with the square root of each number?Given an array of objects, how would you use the
reduce()
method to group the objects by a specific property value?Given an array of numbers, how would you use the
filter()
method andreduce()
method together to find the sum of all the even numbers?
Subscribe to my newsletter
Read articles from Piyush Tyagi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
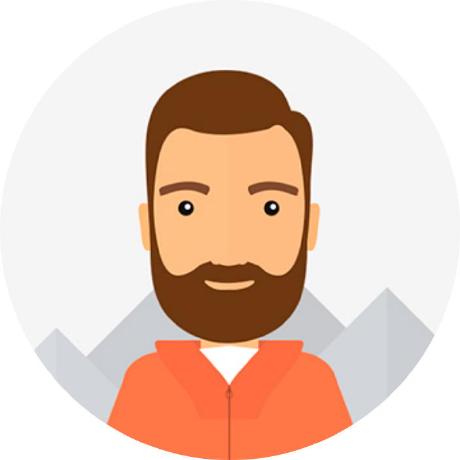
Piyush Tyagi
Piyush Tyagi
Passionate Frontend Web Developer and tech enthusiast, learning HTML, CSS, JavaScript and exploring the latest in web development. College dropout, seeking alternative ways of learning and growing in the tech industry to create stunning web experiences for users.