Array in JavaScript

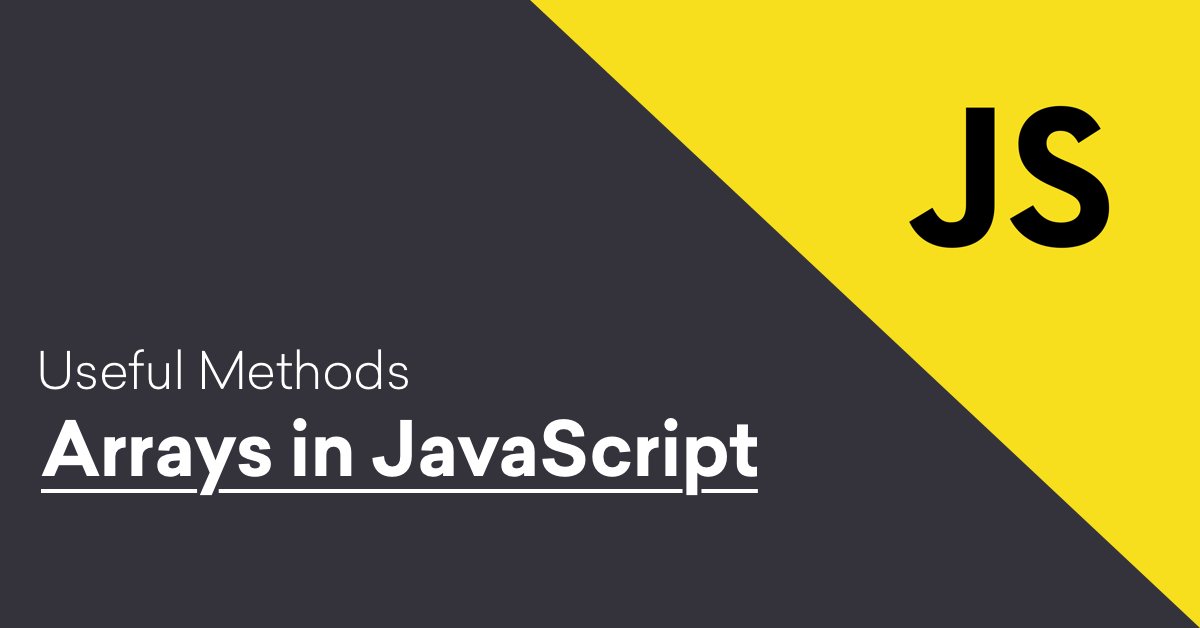
Array:
An array is a special variable, which can hold more than one value:
An array can hold many values under a single name, and you can access the values by referring to an index number.
you can create array with help of this brackets
[]
. and you can itrate array elements one by one with the help of loop.For example
we can create array by two methods like:-
- direct array diclare
```javascript
const cars = ["Saab", "Volvo", "BMW"]; //create a array
```
create array with in variable with the help of
new
keyword.const cars = new Array("Saab", "Volvo", "BMW");
Array Methods:
JavaScript provides a number of built-in methods for working with arrays, such as adding, removing, and modifying elements. Here are a few examples of commonly used array methods:
1. push()
:
This method adds one or more elements to the end of an array.
let num = [1,2,3,4,5];
num.push(6,7,8,9,10);
console.log(num); //output [1,2,3,4,5,6,7,8,9,10]
2. pop()
:
This method removes the last element of an array and returns it.
let num =[1,2,3,4,5];
console.log(num.pop()); //output [1,2,3,4]
3. shift()
:
This method removes the first element of an array and returns it.
let num = [1,2,3,4,5];
console.log(num.shift()); //output [2,3,4,5]
4. unshift()
:
This method adds one or more elements to the beginning of an array.
let num = [3,4,5]
num.unshift(1,2);
console.log(num); //output [1,2,3,4,5]
5. slice()
:
This method returns a new array that contains a subset of the original array, specified by a start and end index.
let num = [1,2,3,4,5];
let slicePart = num.slice(2,4);
console.log(num); //output [1,2,3,4,5]
console.log(slicePart); // output [3,4,5]
6. length()
:
The length
property provides an length of array:
let num = [1,2,3,4,5]
console.log(num.length());
7. concat()
:
The concat()
method creates a new array by merging (concatenating) existing arrays
const myGirls = ["riya", "sumi"];
const myBoys = ["reyan", "barks", ];
const myChildren = myGirls.concat(myBoys);
8. splice()
:
The splice()
method adds new items to an array. 2 means add these fruits after index number of 2 and 1 is remove one element after 2nd index number.
const fruits = ["Banana", "Orange", "Apple", "Mango"];
fruits.splice(2, 1, "Lemon", "Kiwi");
9. slice()
The slice()
method slices out a piece of an array into a new array.
const fruits = ["Banana", "Orange", "Lemon", "Apple", "Mango"];
const citrus = fruits.slice(1); //remove first element
10.toString()
converts an array to a comma separated string when a primitive value is expected.
const fruits = ["Banana", "Orange", "Apple", "Mango"];
let a = fruits.toString();
console.log(a);
11. sort()
The sort()
method sorts an array alphabetically:
const fruits = ["Banana", "Orange", "Apple", "Mango"];
fruits.sort();
Array itteration:-
how to use a for
loop to iterate through and print the elements of an array:
let num = [1, 2, 3, 4, 5];
for (let i = 0; i < num.length; i++) {
console.log(num[i]);
}
The for
loop iterates through the array by using the length
property of the array to determine the number of iterations. The i
variable is used as the index to access each element of the array. On each iteration, the current element is logged to the console using the console.log()
method.
Another way to iterate through an array is using forEach method which applies a function to every element of an array.
let arr =[1,2,3,4,5];
arr.forEach(function(elements){
console.log(elements);
})
or ittrate by arrow function in forEach loop:-
let arr=[1,2,3,4,5];
arr.forEach(elements => console.log(elements))
Subscribe to my newsletter
Read articles from Megha Khatri directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
