Flatlist in React Native
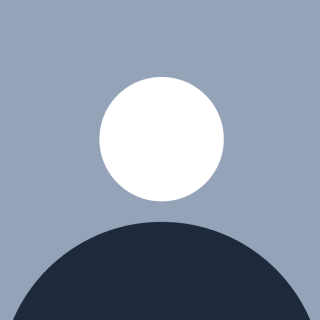
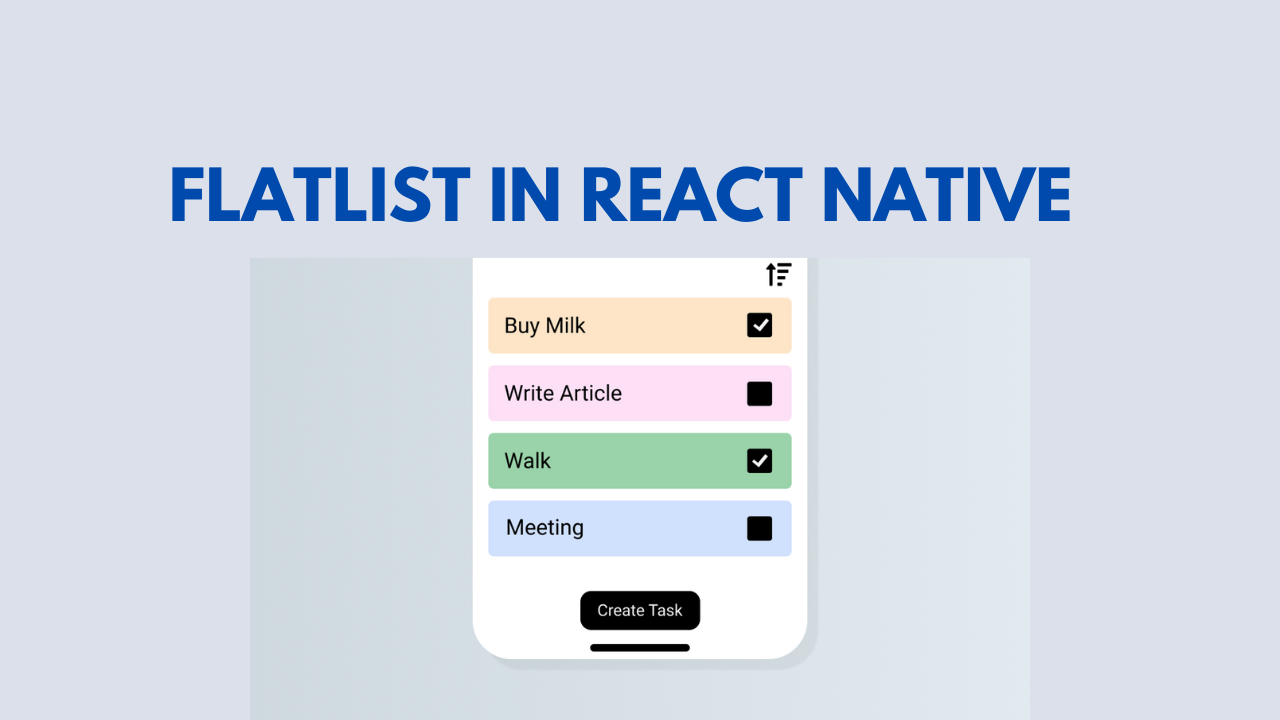
FlatList is a component in React Native that allows for the efficient rendering of large lists of items. It is an improved version of the traditional ListView component and is recommended for use in most cases.
One of the main advantages of FlatList is its ability to handle large lists of data without affecting performance. It achieves this by only rendering the items that are currently visible on the screen, rather than rendering the entire list at once. This results in a smooth and responsive user experience, even when working with thousands of items.
Another benefit of FlatList is its ability to handle changes to the data set easily. It has a built-in mechanism for detecting changes in the data and updating the list accordingly. This means that developers don't have to manually update the list when the data changes.
To use FlatList in a React Native project, you need to import it from the 'react-native' library. After that, you can use it just like any other component in your code. You need to pass the data array and a renderItem
function as props to the FlatList component. The renderItem
function is responsible for rendering each item in the list, and is passed the item data as a parameter.
Here is an example of a basic FlatList implementation:
import { FlatList } from 'react-native';
const data = [{key: 'Item 1'}, {key: 'Item 2'}, {key: 'Item 3'}];
function MyList({data}) {
return (
<FlatList
data={data}
renderItem={({item}) => <Text>{item.key}</Text>}
/>
);
}
FlatList also supports many other props like scrollToEnd, refreshing, onRefresh, showsVerticalScrollIndicator, keyExtractor
etc. These props can be used to customize the behaviour and appearance of the list as per your needs.
In conclusion, FlatList is a powerful and efficient component that should be used when working with large lists of data in React Native. Its ability to handle changes to the data set easily, along with its performance optimizations, make it the ideal choice for most use cases.
Subscribe to my newsletter
Read articles from Srishti Maurya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by