Props 101: Understanding and Using Props in React.js

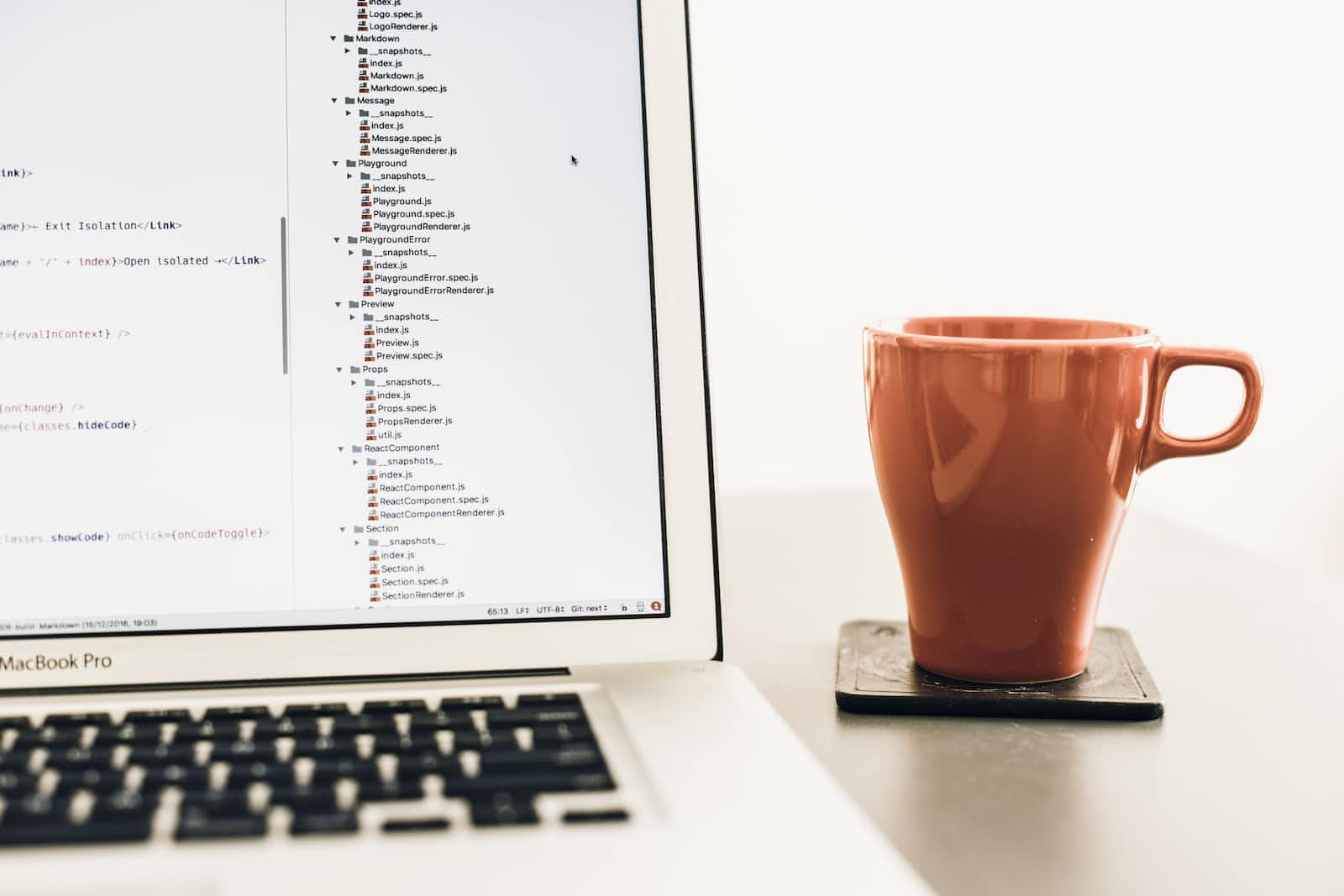
React.js is a popular JavaScript library for building user interfaces, and one of its key features is the ability to pass data between components using "props." In this blog post, we'll explore what props are, and how they work and provide some code examples to illustrate their use.
Props, short for "properties," are a way to pass data from a parent component to a child component. This allows components to be reusable and more modular, as they can receive data from their parent instead of having to manage it themselves.
To pass a prop to a child component, you simply include it as an attribute on the JSX element representing the child component. For example, if we have a simple component called "Hello" that takes a "name" prop, we can use it like this:
function Hello(props) {
return <div>Hello, {props.name}!</div>;
}
function App() {
return <Hello name="React" />;
}
In the above example, the "App" component is passing the string "React" as the value for the "name" prop to the "Hello" component. Inside the "Hello" component, we can access the value of the prop using the "props" object and use it to render the component.
Props are also used to pass callback functions down the component tree. For example, in the following code, a parent component is passing a callback function to a child component via props, and the child component is invoking the callback function:
function Child({ onClick }) {
return <button onClick={onClick}>Click me!</button>;
}
function Parent() {
const handleClick = () => console.log('Button clicked!');
return <Child onClick={handleClick} />;
}
In this example, when the button inside the "Child" component is clicked, it will invoke the "handleClick" function passed down via props, and log a message to the console.
Props are also used to pass the state of the parent component to the child component. For example, in the following code, a parent component is passing a state variable to a child component via props:
function Child({ count }) {
return <div>Count: {count}</div>;
}
class Parent extends React.Component {
state = { count: 0 };
render() {
return <Child count={this.state.count} />;
}
}
In this example, the "Parent" component is maintaining a state variable called "count", and passing its current value to the "Child" component via the "count" prop. The "Child" component is then using this prop to render the current count.
Props are also used to validate the data passed into the component. This is done using propTypes. For example, in the following code, we are validating that the "name" prop passed to the "Hello" component is a string:
import PropTypes from 'prop-types';
function Hello(props) {
return <div>Hello, {props.name}!</div>;
}
Hello.propTypes = {
name: PropTypes.string.isRequired
};
In this example, if the "name" prop passed to the "Hello" component is not a string, React will show a console warning. This can be useful for catching bugs early and ensuring that components are being used correctly.
It's also worth noting that props are read-only, meaning that a child component cannot modify the props passed to it by its parent. If a child component needs to update some data that it receives via props, it should instead use the "state" feature of React to manage its internal state.
In conclusion, props are a powerful feature of React that allows you to pass data between components and make them more modular and reusable. By using props, you can separate the concerns of different components and build more complex user interfaces with ease. With the examples and explanations provided in this blog post, you should have a good understanding of how to use props in your React projects.
Subscribe to my newsletter
Read articles from udoy rahman directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

udoy rahman
udoy rahman
I am a developer from Bangladesh. I love programming and circuits.