Getting Started With .NET
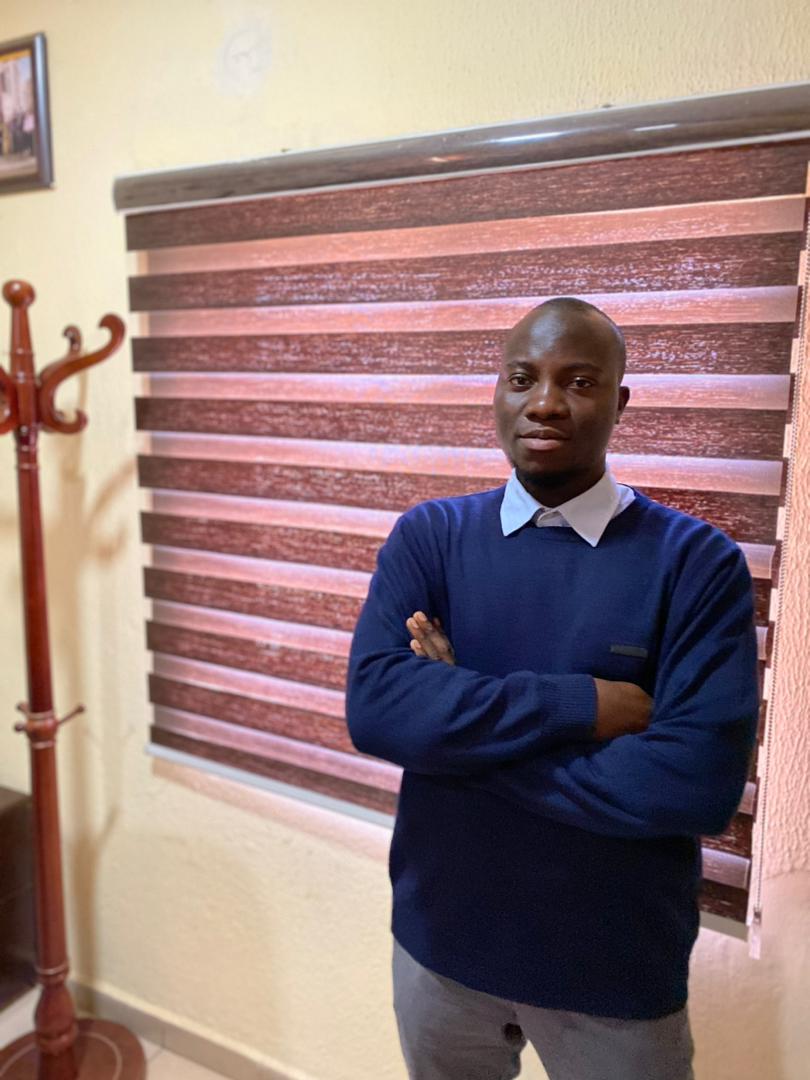
A lot of newbie developers often get confused when they see terms like C#.NET, VB.NET, F#.NET, .NET Framework, .NET Core, and .NET Standard, etc. All these technologies are related to each other even though they are not the same. They all make up the more extensive Microsoft Ecosystem.
In this tutorial, I will try to demystify what these terms mean and how you can get started building applications using . NET.
What is .NET?
.NET is an open-source, cross-platform project developed by Microsoft for building different types of applications. The .NET platform consists of tools to build web applications, mobile applications, desktop applications, game and cloud applications, etc.
.NET FRAMEWORK, .NET CORE, AND .NET STANDARD.
.NET Framework and .NET Core are two different implementations of .NET.
.NET FRAMEWORK
The .NET Framework is the original and earliest implementation of .NET. Microsoft released the first version of the .NET Framework in the year 2002.It was used to develop applications for the Windows operating system only, although Microsoft had the long-term idea of making it cross-platform. Cross-platform software is any software application that works on multiple operating systems i.e., Windows, Mac, Linux, etc.
Essentially, .NET Framework was used to develop applications that ran on the Windows operating system alone, it doesn’t support another operating system.
MONO
Mono is an attempt at making.NET Framework cross-platform. It's a third-party implementation of the .NET Framework. Mono seeks to allow .NET developers to create and develop cross-platform versions of their applications, but, it fell behind the official implementation of the .NET Framework. There were some functionalities in the official Microsoft .NET Framework implementation that were not implemented.e.g Windows Presentation Foundation(WPF).
.NET CORE
Remember that, .NET Framework runs on the Windows Operating System only? In a bid to make the .NET platform available everywhere, Microsoft released another implementation of .NET called .NET Core in 2016. The .NET Core is a cross-platform implementation of .NET runtime. It was completely written from scratch.
.NET STANDARD
.NET Standard is not another implementation of . NET. It is a formal specification of .NET that are available on multiple .NET implementations. It was meant to establish greater uniformity in the .NET ecosystem because of its different implementations.
In other words, .NET Standard defines a set of APIs, that a particular .NET implementation has to implement to be compliant with that particular .NET Standard.
THE JOURNEY TO ONE .NET
In 2019, Microsoft announced that,.NET5.0 would be its first major attempt at unifying all .NET frameworks(Note the use of .NET “framework,” lowercase, referring to all .NET frameworks in general while .NET “Framework,” uppercase, referring to the Windows .NET Framework, such as .NET Framework 4.8.).
.NET Core has been renamed .NET without Core, and the major version number has skipped 4 to avoid confusion with .NET Framework 4. x.
.NET5.0 is an important step forward for developers working across desktop, web, mobile, cloud, and device platforms. It's a unification of several key frameworks-.NET Framework,.NET Core, and Xamarin(used for mobile development). It seeks to provide developers with one target framework for their work.
One of the benefits of unifying the frameworks, runtimes and developer toolsets is the reduction in the amount of duplicate code that developers will need to maintain and expand.
Read more about the unification project here.
From my earlier explanation of .NET frameworks, you would understand that .NET is not a programming language, it’s a platform that you build on top of.
It has two components, Base Class Library(BCL) and the Command Line Runtime, (CLR) which allows it to run applications written in any. NET-supported programming language. There are many programming languages built on top of . NET.Some of them are C#, F#, Iron Python, PowerShell, etc. So basically, C#.NET,F#.NET and VB.NET are terms developers use to depict the implementation of these programming languages on top of the .NET platform.
During compilation, the compiler converts the source code into an Intermediate Language(IL) code, and stores it in an assembly. At runtime, the .NET Command Line Runtime(CLR) loads the Intermediate Language code from the assembly and compiles it again into the native CPU instructions which would ultimately be executed by the CPU of the machine. Through this process, the .NET platform can execute any program written in either C#, F#, and VB.NET or any other programming language. Essentially, the .NET compilation process is a two-way process.
The two-step compilation implies that the same Intermediate Language generated in the first compilation can run everywhere because of the second compilation which generates code for the native operating system be it Windows OS, or Linux.
WRITING YOUR FIRST APPLICATION
To write a .NET application, you need to install a code editor or an Integrated Development Editor(IDE), and some other tools. Fortunately for us, Microsoft provides us with a family of code editors and Integrated Development Environments (IDEs).
You can download your choice here. While some of the IDEs are free some are paid versions. Download the one you are most comfortable with.
For this tutorial, I will use Visual Studio Code as my code editor and C# extension in Visual Studio Code to develop the application.
Let’s now download and install Visual Studio Code,.NET SDK and C#.
Download and install Visual Studio Code from the following link: https://code.visualstudio.com/.
Download and install the .NET SDKs for versions 6.0 and 7.0 from the following link:www.microsoft.com/net/download.
To install the C# extension, you must first launch the Visual Studio Code application.
In Visual Studio Code, click the View icon and then click the Extensions icon.
Enter C# in the search box and click on the first item in the search result.
Click Install and wait for supporting packages to download and install.
Let’s get started writing code!
Create a folder on your desktop where you want to save your application.
Start Visual Studio Code.
Click on Open Folder in the Visual Studio start page to navigate to the folder you created in step 1.
Click on the Terminal icon in Visual Studio Code and then click on the New Terminal icon.
In the Terminal, make sure that you are in the folder you created, then use the .NET CLI to create a new console app, as shown in the following command.
dotnet new console -o HelloCS
You will see that .NET command-line tool creates a new Console App project for you in the current folder, and the Explorer window shows the two files created, HelloCS.csproj and Program. cs, and the obj folder, as shown in fig1.0
In EXPLORER, click on the file named Program. cs to open it in the editor window. The first time that you do this, Visual Studio Code may have to download and install C# dependencies like OmniSharp, .NET Core Debugger, and Razor Language Server, if it did not do this when you installed the C# extension or if they need updating. Visual Studio Code will show progress in the Output window and eventually the message Done, as shown in the following output:
Folders named obj and bin would have been created and when you see a notification saying that required assets are missing, click Yes, as shown below:
Modify the code in the Program.cs file with the code snippets below. The code requests two numbers from the user through the console and outputs the sum of the two numbers.
Console.WriteLine("Enter your first number"); int firstNumber = Converts.ToInt32(Console.ReadLine()); Console.WriteLine("Enter your second number"); int secondNumber = Converts.ToInt32(Console.ReadLine()); int sum = firstNumber + secondNumber; Console.ReadLine(sum);
Type the following command in your terminal to run your code.
dotnet run
The output in the Terminal window will show the result of running your application as shown below.
Congratulations on writing your first application!
You can learn more about the .NET here
See ya later!
Subscribe to my newsletter
Read articles from Rasheed Yusuf directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
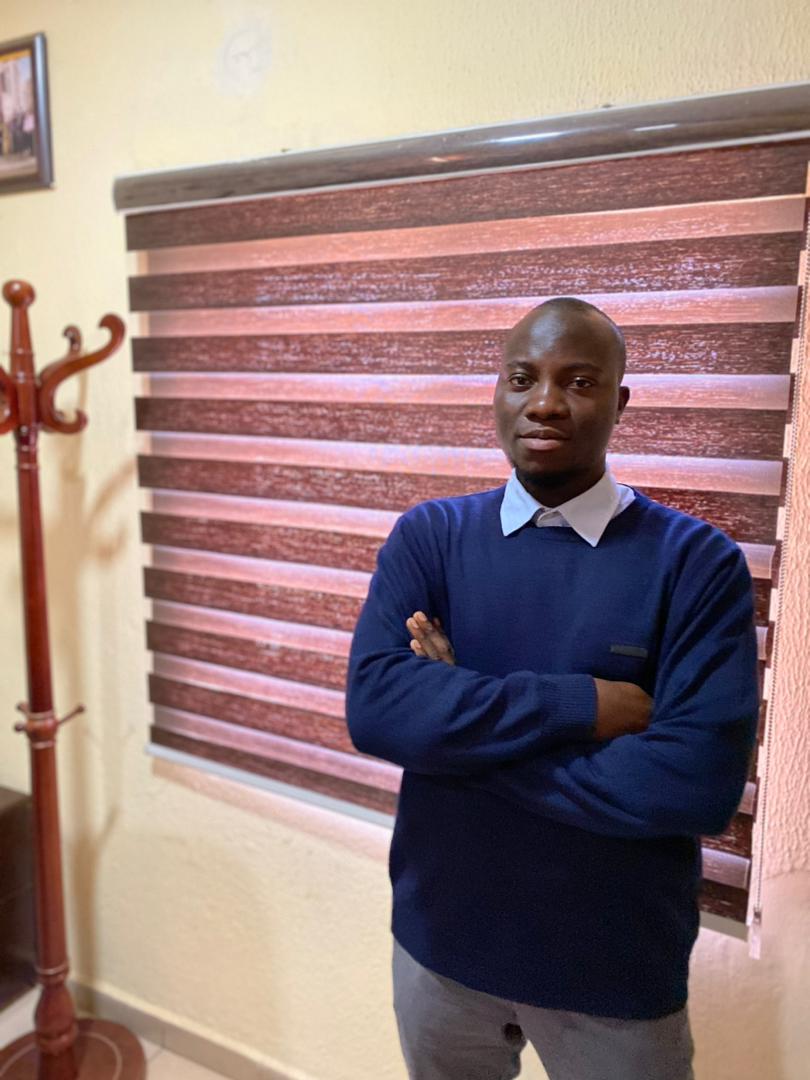
Rasheed Yusuf
Rasheed Yusuf
I'm a budding software engineer who loves to share as I learn new things.