A Fast and Simple Logger dedicated to JSON Output
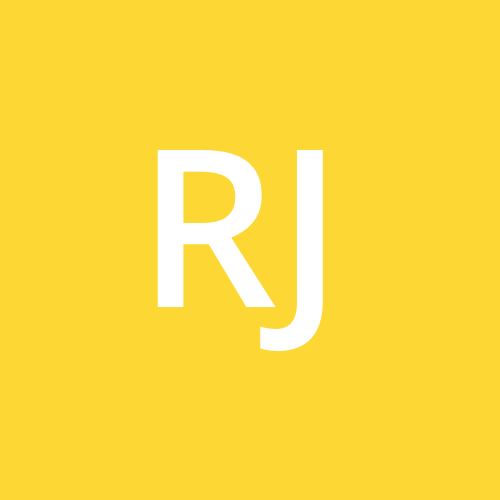
Logging is an important aspect of any application, and it is crucial for debugging, monitoring and troubleshooting. In Go, Zerolog is a popular logging library that provides a fast and easy-to-use API for logging messages. In this post, we will explore the features of Zerolog, and discuss best practices for logging with Zerolog in Go.
Zerolog is a logging library that is designed to be fast and easy to use. It is built on top of the standard Go log package and provides a number of additional features, such as JSON output and support for structured logging. Zerolog's API is designed to be simple and easy to understand, and it provides a number of functions for logging messages at different levels, such as Debug, Info, and Error.
One of the main features of Zerolog is its support for JSON output. By default, Zerolog logs messages in JSON format, which makes it easy to parse and analyze log messages using tools like Logstash and Elasticsearch. JSON output also makes it easy to send log messages to a remote log server, such as a SaaS log management service, for further analysis and storage.
Another key feature of Zerolog is its support for structured logging. Structured logging is a technique in which log messages are written in a structured format, such as JSON, which makes it easy to search, analyze, and correlate log messages. Zerolog provides a number of functions for logging structured messages, such as log.Info().Str("key", "value"). This makes it easy to add context to log messages and to search and filter log messages based on their contents.
When logging with Zerolog, it's important to follow best practices for designing and implementing the log messages. Here are some tips to keep in mind:
Use the right log level: Use the appropriate log level for the message you are logging. For example, use log.Info() for informational messages, log.Error() for error messages, and log.Debug() for debugging messages.
Add context to log messages: Use the structured logging features of Zerolog to add context to log messages, such as the request ID or the user ID.
Use the right output format: Use JSON output format to make it easy to parse and analyze log messages.
Rotate log files: Rotate log files to avoid running out of disk space.
Avoid logging sensitive information: Avoid logging sensitive information such as passwords, credit card numbers, or other personal information.
Test your logging configuration: Test your logging configuration to ensure that log messages are being written to the correct location and in the correct format.
Monitor your logs: Monitor your logs to ensure that they are being written correctly and to detect errors or performance issues.
Getting Started:
Get the library: Use the go get command to install Zerolog library.
go get -u github.com/rs/zerolog
Import the library: In your Go code, import the Zerolog library using:
import "github.com/rs/zerolog"
Initialize the logger: Initialize the Zerolog logger in your code by creating a new Zerolog logger.
logger := zerolog.New(os.Stdout)
Logging messages: Use the appropriate function to log messages at different levels, such as Info, Error, and Debug.
logger.Info().Msg("Hello World!") logger.Error().Msg("An error occurred!") logger.Debug().Msg("Debug message")
Customizing the output: Zerolog supports different outputs and encoders, you can customize your output by choosing a different output or encoder, for example:
logger := zerolog.New(os.Stdout).With().Timestamp().Logger()
Enabling/disabling log level: You can also enable or disable certain log levels by using the level function
zerolog.SetGlobalLevel(zerolog.InfoLevel)
Run your application: Now you can run your application and see the log messages in the console or in the output destination you've chosen.
Zerolog is a powerful and easy-to-use logging library for Go that provides support for JSON output and structured logging. By following best practices for designing and implementing log messages and using the right tools and frameworks, developers can take full advantage of Zerolog's features to log messages that are fast, easy to parse, and easy to analyze.
One of the great things about Zerolog is its support for multiple output destinations, it allows you to log messages to different outputs like console, file, network, and more. This allows you to customize your logging strategy based on your needs and requirements.
Another great thing about Zerolog is its ability to log messages with different levels of verbosity. This means that you can log messages at different levels, such as Debug, Info, and Error, and filter them based on their level. This allows you to control the amount of information that is logged and makes it easier to focus on the most important log messages.
When it comes to debugging and troubleshooting, Zerolog makes it easy to add context to log messages by using structured logging. This allows you to add information such as request ID, user ID, or any other relevant information to your log messages, which makes it easier to search and filter log messages based on their contents.
Conclusion
Zerolog is a powerful and easy-to-use logging library for Go that provides support for JSON output and structured logging. By following best practices for designing and implementing log messages, using the right tools and frameworks, and taking advantage of Zerolog's features, developers can log messages that are fast, easy to parse, and easy to analyze. Zerolog is a great choice for any project that needs a logging library that is fast, easy to use, and provides a lot of flexibility.
Subscribe to my newsletter
Read articles from Ritwik Jain directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
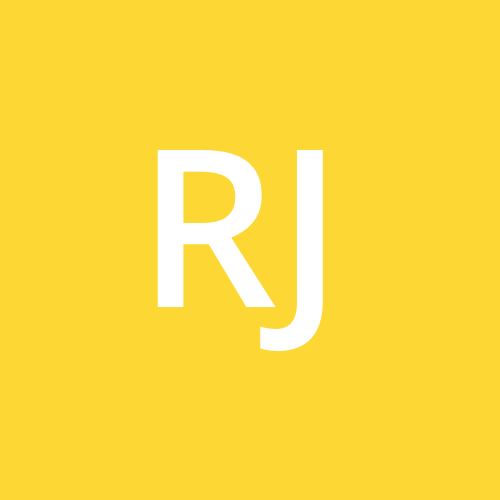