How to sort an array of objects by one or more of their properties
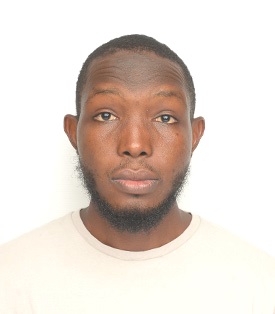
If you have an array of objects such as people, cars etc., and you wish to sort them in a given order, the sort method of the array object is handy in doing so. The sort method is not limited to primitive values like a number and a string, it is also applicable to objects. For example, below is an array of people with the name, age, and height properties.
const people = [
{name: 'Aliyu', age: 23, height: 1.4},
{name: 'Musa', age: 19, height: 1.2},
{name: 'Sani', age: 30, height: 1.3},
]
Let us suppose we want to arrange the person objects by their age property, the following code snippet will help:
let sorted = people.sort((x, y)=> (x.age > y.age)? 1 : -1)
console.log(sorted)
Output:
[
{ name: 'Aliyu', age: 19, height: 1.2 },
{ name: 'Musa', age: 23, height: 1.4 },
{ name: 'Sani', age: 30, height: 1.3 }
]
The code snippet above invokes the sort method on the “people” array and iterates through the array, passing a callback function with the next and current array items as arguments represented by x and y respectively. We then use a ternary operation to return 1 if the value of the age property of the x object is greater than that of the y object. The callback function communicates with the sort method to put the x object before y object if the return value is 1, otherwise, the order is left unchanged.
Although, it takes fewer lines to implement it using ternary operation, the same thing is achievable with if-else statement:
let sortedArr = people.sort((x, y)=>{
console.log(x, y)
if(x.age > y.age) return 1
else return -1
})
console.log(sortedArr)
Output:
[
{ name: 'Aliyu', age: 19, height: 1.2 },
{ name: 'Musa', age: 23, height: 1.4 },
{ name: 'Sani', age: 30, height: 1.3 }
]
Sorting based on two properties:
We may wish to sort our array of people based on both age and height properties. In that case, we have to make some changes to our code to do so, and it is achievable using either a ternary operation or an if-else statement. To illustrate that, we are going to modify the “people” array like so:
const people = [
{name: 'Aliyu', age: 19, height: 1.5},
{name: 'Musa', age: 23, height: 1.4},
{name: 'Sani', age: 23, height: 1.3},
]
Using ternary operation:
const sortedArr = people.sort((x, y)=> (x.age > y.age)? 1 : (x.age === y.age)?(x.height > y.height)? 1: -1 : -1)
console.log(sortedArr)
Output:
[
{ name: 'Aliyu', age: 19, height: 1.5 },
{ name: 'Sani', age: 23, height: 1.3 },
{ name: 'Musa', age: 23, height: 1.4 }
]
The above code snippet sorts the array items in descending order of their age property. However, if both x and y objects have the same age, they are sorted based on their height.
Using if-else statement:
let sortedArr = people.sort((x, y)=>{
console.log(x, y)
if(x.age > y.age) return 1
else{
if (x.height > y.height){
return 1
}else{
return -1
}
}
})
console.log(sortedArr)
Output:
[
{ name: 'Aliyu', age: 19, height: 1.5 },
{ name: 'Sani', age: 23, height: 1.3 },
{ name: 'Musa', age: 23, height: 1.4 }
]
Summary
It is possible to sort an array of objects based on their properties in either ascending or descending order with the help of the sort method. I find it very useful whenever I want to display some objects in sorted order in React applications.
Thanks for reading and I hope you enjoy it, and I will appreciate it if you give me a like and share it with your friends.
Subscribe to my newsletter
Read articles from Bello Shehu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
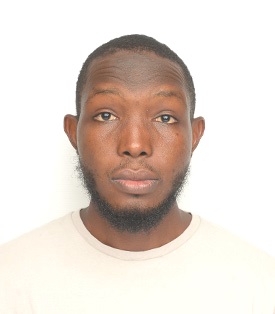