Simplifying Your Code: A Deep Dive into React's useContext API

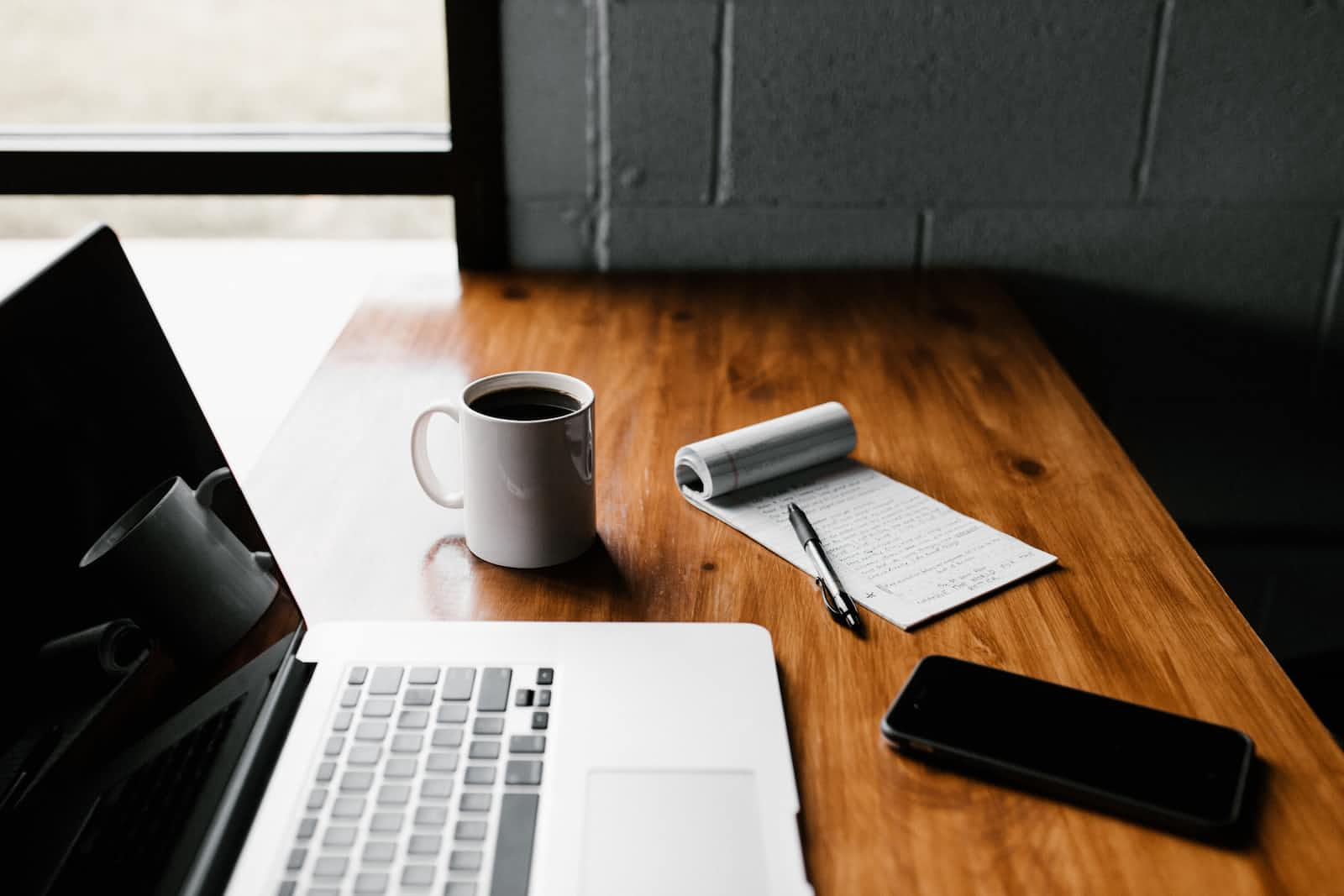
React's useContext
API allows you to share state between components without the need for props drilling. It is a powerful tool that can help simplify your code and make it more readable. In this blog post, we will go over how to use useContext
in a React project, and we will also provide code snippets to help illustrate the concepts discussed.
To begin, you will need to create a context object. A context object is an object that holds the state that you want to share between components. Here is an example of how you can create a context object:
const MyContext = React.createContext();
Once you have created a context object, you can then provide it to the components that need to access the state. To do this, you will need to use the Provider
component. The Provider
component takes the context object as a prop, and it makes the state available to all of its children. Here is an example of how you can use the Provider
component:
<MyContext.Provider value={someState}>
<App />
</MyContext.Provider>
Now that the state is being provided, we can access it in any child component using the useContext
hook. useContext
takes the context object as an argument, and it returns the current value of the context. Here is an example of how you can use the useContext
hook:
const someState = useContext(MyContext);
You can also use the context to update the state. The Provider
component accepts a value
prop that can be any type of data, and it's often an object that holds the state and a set of functions to change it.
const [state, setState] = useState({
name: "John Doe",
age: 25,
});
<MyContext.Provider value={[state, setState]}>
<App />
</MyContext.Provider>
And in any component that need to access it:
const [state, setState] = useContext(MyContext);
Here's a complete example of how to use the useContext
API in a React project:
import React, { useState, useContext } from "react";
const MyContext = React.createContext();
function App() {
const [state, setState] = useState({
name: "John Doe",
age: 25,
});
return (
<MyContext.Provider value={[state, setState]}>
<div className="App">
<header className="App-header">
<Child1 />
<Child2 />
</header>
</div>
</MyContext.Provider>
);
}
function Child1() {
const [state, setState] = useContext(MyContext);
return (
<div>
<p>Name: {state.name}</p>
<p>Age: {state.age}</p>
<button onClick={() => setState({ ...state, age: state.age + 1 })}>
Increment Age
</button>
</div>
);
}
function Child2() {
const [state, setState] = useContext(MyContext);
return (
<div>
<p>Name: {state.name}</p>
<p>Age: {state.age}</p>
<button onClick={() => setState({ ...state, name: "Jane Smith" })}>
Change Name
</button>
</div>
);
}
export default App;
In this example, we have an App component that uses the MyContext.Provider
to provide the state and setState function to all of its children components, Child1
and Child2
. Both of these components use the useContext
hook to access the state and setState function. Child1 component increases the age by 1 and Child2 component changes the name.
It's important to note that using context can have a performance impact and it's recommended to use it only when it's necessary. It's also important to keep in mind that context should be used for global or app-level state, whereas component state should be managed with useState
hook.
In conclusion, React's useContext
API is a powerful tool that allows you to share state between components without the need for props drilling. It simplifies the code and makes it more readable. With the examples and code snippets provided in this blog post, you should now have a good understanding of how to use the useContext
API in a React project.
Subscribe to my newsletter
Read articles from udoy rahman directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

udoy rahman
udoy rahman
I am a developer from Bangladesh. I love programming and circuits.