Reverse a string or array

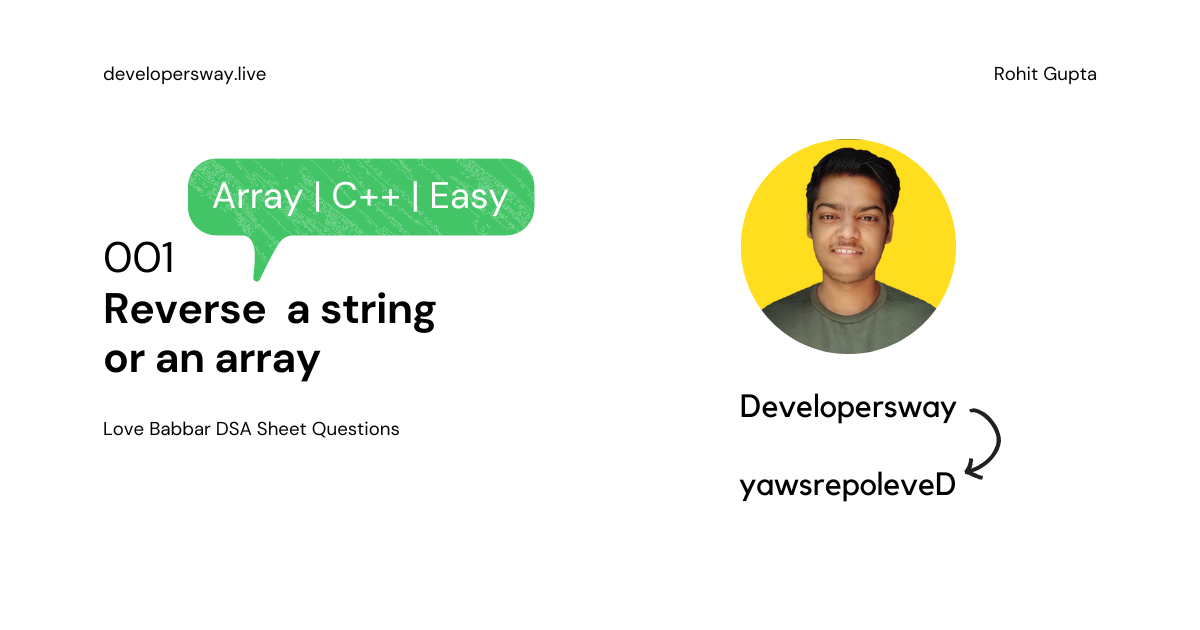
Problem Statement
You have given a string A. Your task is to reverse that string.
Example
input: Developersway
output: yawsrepoleveD
Approaches
Approach 1
Create an empty string B.
Then iterate over string A from its end to the start for each of its characters.
Concatenate each character of string A in string B.
Time Complexity : 0(n)
Space Complexity : O(n)
Approach 2
Iterate over string A up to half of its length.
if the length of the string is n then iterate till n/2
swap the ith character of string A with the ith character from last.
1st character is swapped with the last character, and the second character is swapped with the second last character. and so on till n/2.
Time Complexity : 0(n)
Space Complexity: O(1) // here space complexity is constant (lesser then previous)
Code for Approach 2
#include <iostream>
using namespace std;
string reverseWord(string str){
int n = str.length();
int start = 0;
int end = n-1;
while(start < end){
char temp = str[start];
str[start] = str[end];
str[end] = temp;
start++;
end--;
}
return str;
}
int main()
{
string A;
cin>>A;
cout<<reverseWord(A)<<endl;
return 0;
}
input: Developersway
output: yawsrepoleveD
Hope this article will help if you have any problem regarding this article feel free to comment.
#reverse a string #reverse an array #Love Babbar DSA sheet
Subscribe to my newsletter
Read articles from Rohit Gupta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
