Creating A Custom Event In JavaScript: Simplest Explanation
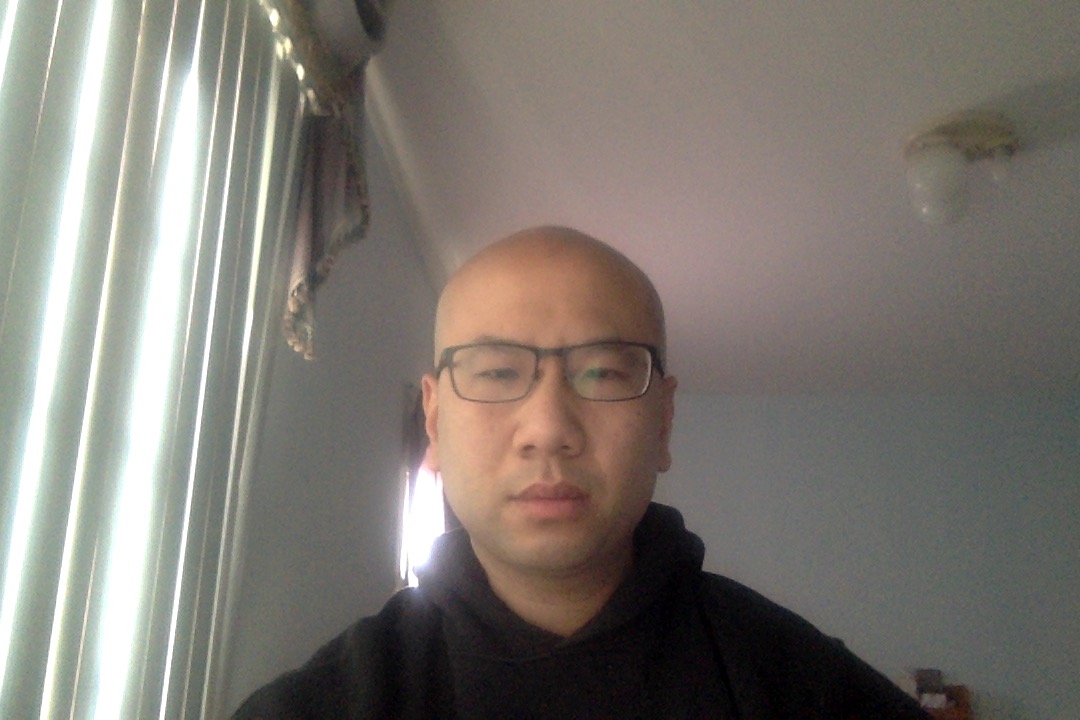
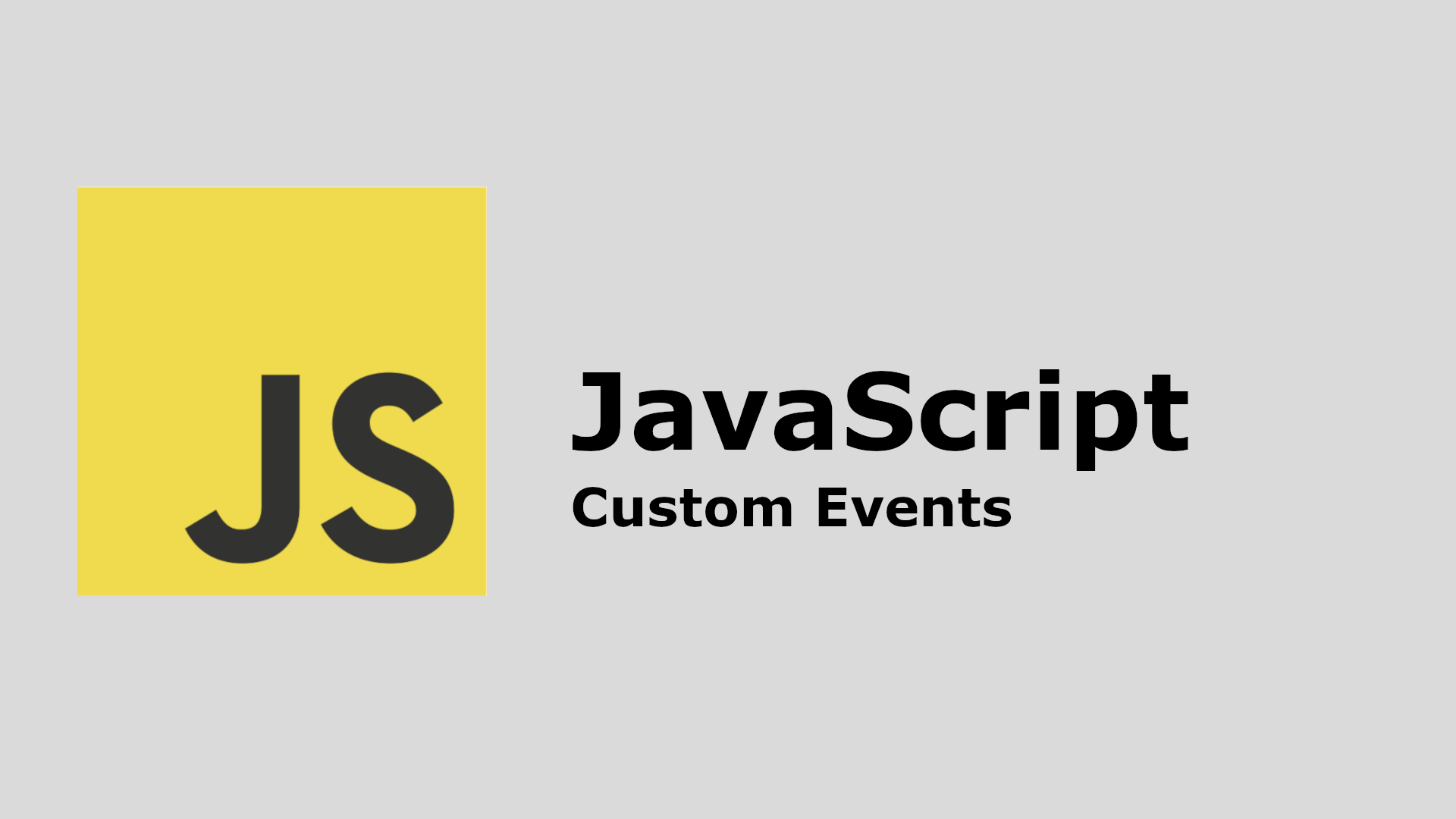
In this post, I will explain what are custom events in the simplest possible way.
In summary: it's not as complicated or cool as it sounds; it is only a one-line thing.
To create a custom event, we simply use the syntax:
const event = new Event(TheEventsName)
That's it!
You can name the event anything you want.
Does The Custom Event Do Anything?
No. :)
For something to happen during this event, you have to trigger the event and then set a function to execute when the event is triggered. The reason you want to create a custom event is for you to say: when you programmatically trigger a particular event, we want some function to execute.
Here is an example of how to do this:
Example
let boo = new Event("boo");
const thing = document.getElementById("thing");
thing.addEventListener('boo', function(){
console.log("Hello World");
});
thing.addEventListener("click", function(){
this.dispatchEvent(boo);
})
The above example says, when I click on the element with Id of thing(represented by the const thing), I will trigger the boo event(per dispatchEvent()).
And when the boo event is triggered, I will console.log "Hello World".
Subscribe to my newsletter
Read articles from Eric directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
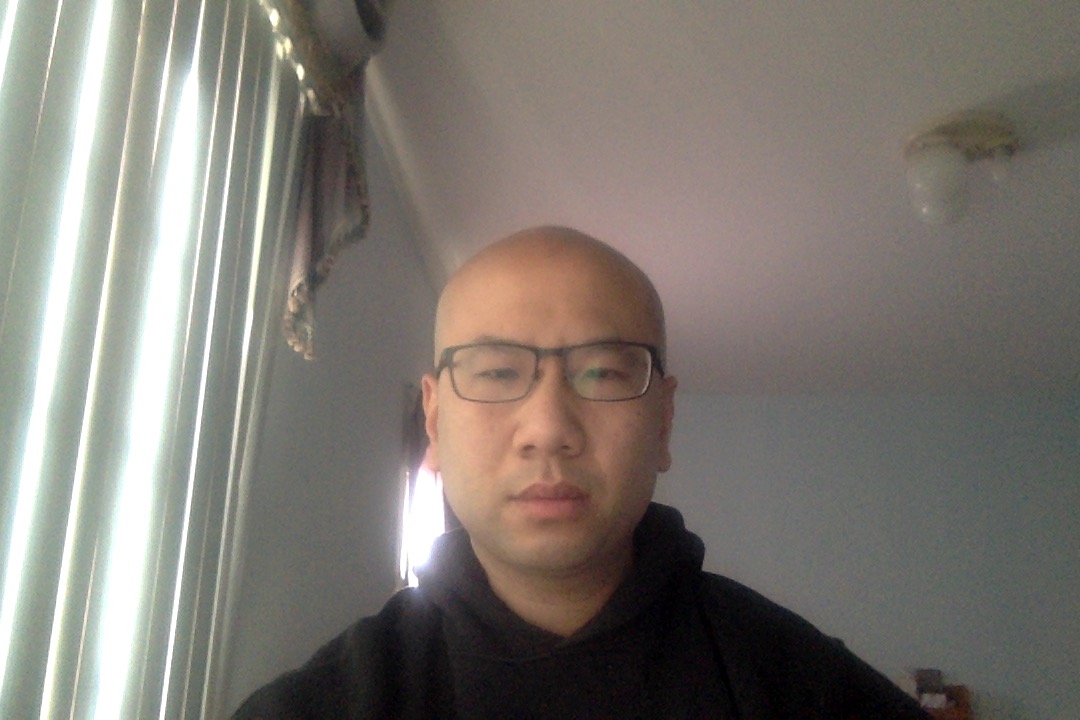
Eric
Eric
I'm a full-stack web developer who loves building new projects and learning new things It might not show from my posts, but I'm also quite funny.