Min and Max element of an Array

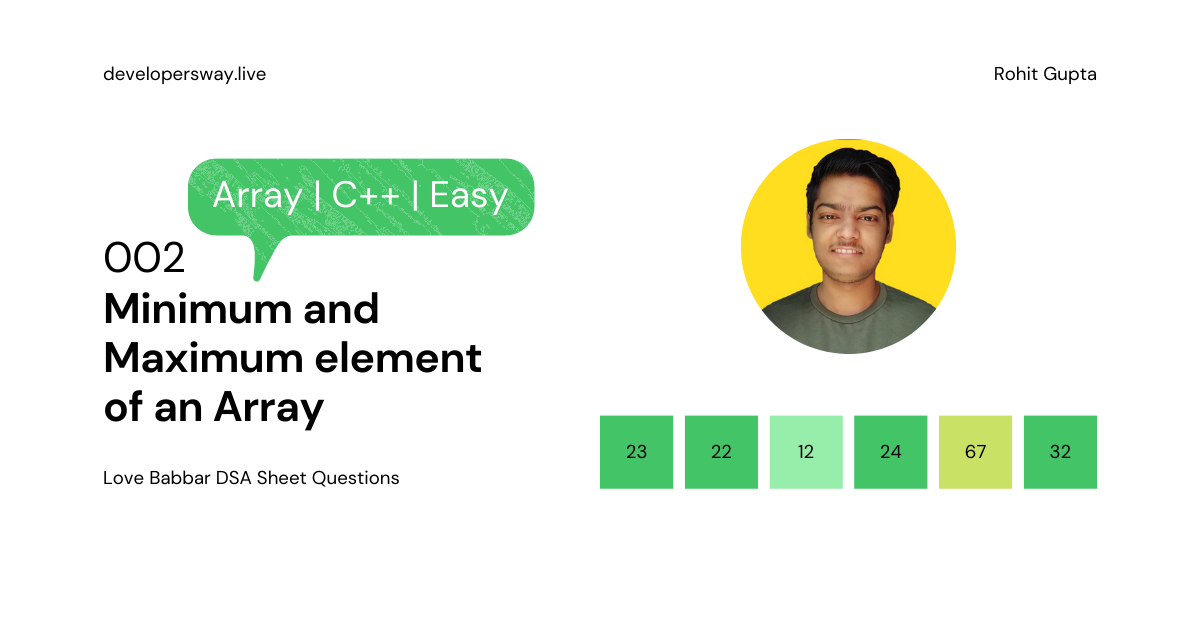
Problem Statement
You have given an array A you have to find the minimum and maximum element of that array.
Example
input: 23 22 34 12 54 21 45 67
output: 12 67
Approaches
Approach 1
you can sort the array using some sorting algorithm and return the first and last element of the array
Time Complexity : 0(n log(n)) // minimum time complexity
Space Complexity : O(1) // minimum space complexity
Approach 2
set minimumValue and maximumValue the first element of the array A
Iterate over the array and compare all the elements with minimumValue and maximumValue.
if minimumValue \> A[i] then, minimumValue \= A[i]
In the end, return the minimumValue and maximumValue from the function.
Code for Approach 2
#include <bits/stdc++.h>
using namespace std;
pair<long long, long long> getMinMax(int A[], int n) {
long long minimumValue = A[0];
long long maximumValue = A[0];
for(int i = 0 ; i < n ; i++){
if(a[i] < minimumValue) minimumValue = a[i];
if(a[i] > maximumValue) maximumValue = a[i];
}
return make_pair(minimumValue , maximumValue);
}
int main()
{
int n;
cin>>n;
int A[n];
for(int i = 0 ; i < n ; i++){
cin>>A[i];
}
pair<int , int> ans = getMinMax(A , n);
cout<<ans.first<<" "<<ans.second<<endl;
return 0;
}
input: 8
23 22 34 12 54 21 45 67
output: 12 67
Hope this article will help if you have any problem regarding this article feel free to comment.
#reverse a string #reverse an array #Love Babbar DSA sheet
Subscribe to my newsletter
Read articles from Rohit Gupta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
