Using React Portals to Render Components Outside the Main DOM Tree
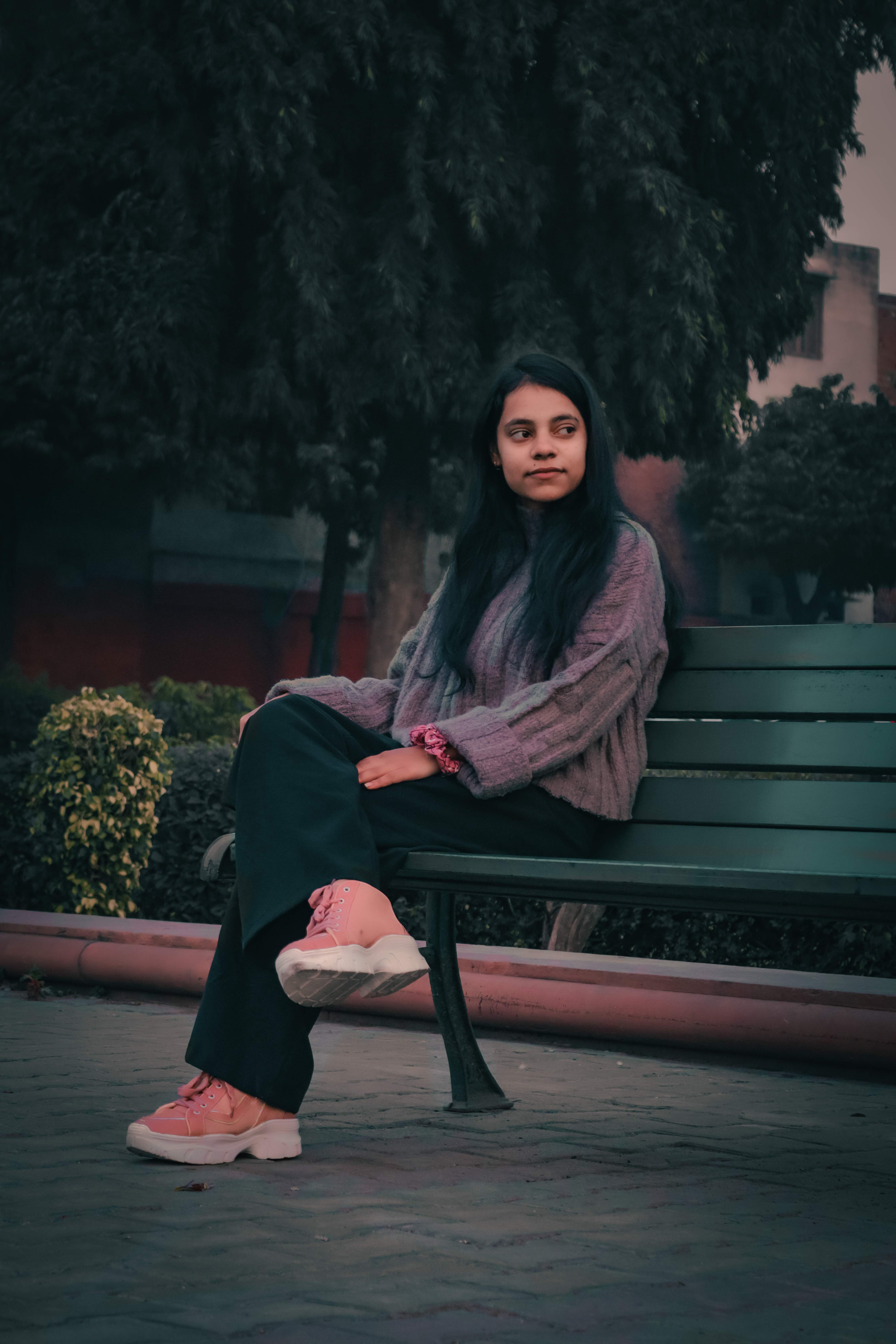
Table of contents
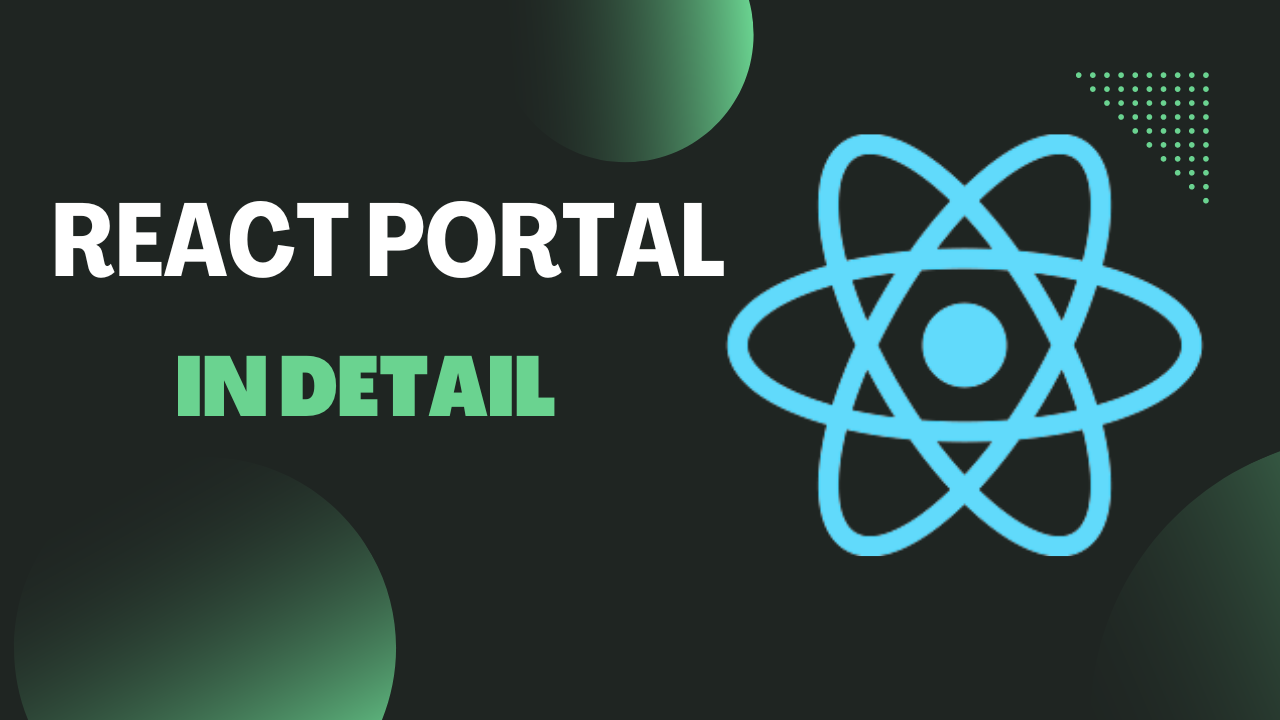
React portals are a way to render a component outside of its parent component’s DOM tree. This can be useful in situations where you want to render a component over multiple parts of the page, or when you want to render a component outside of the current component’s DOM hierarchy
Here’s an example of how to use a portal in a functional component:
import { createPortal } from 'react-dom';
function Modal({ children }) {
return createPortal(children, document.getElementById('modal-root'));
}
function App() {
return (
<div>
<button onClick={() => setShowModal(true)}>Show Modal</button>
{showModal && (
<Modal>
<div>This is the modal content</div>
</Modal>
)}
</div>
);
}
Now you might error because of this:
document.getElementById('modal-root')
let’s add this to our index.html
<body>
<noscript>
You need to enable JavaScript to run this app.
</noscript>
<div id="root"></div>
<div id="modal-root"></div>
<!--
This HTML file is a template.
If you open it directly in the browser, you will see an empty page.
You can add webfonts, meta tags, or analytics to this file.
The build step will place the bundled scripts into the <body> tag.
To begin the development, run `npm start` or `yarn start`.
To create a production bundle, use `npm run build` or `yarn build`.
-->
</body>
In this example, we have two divs: root
and modal-root
. The root
div is where the React application is rendered, and the modal-root
div is where the portal will render the modal. In the React code, we use document.getElementById('modal-root')
to specify that the modal should be rendered inside the modal-root
div.
When the button is clicked, the Modal
component will render its children (in this case, a div with the text "This is the modal content") inside the modal-root
div, which is outside of the root
div. This allows the modal to appear on top of the other content, even though it is rendered outside of the root
div.
It is important that the modal-root
div is placed in the appropriate location in the HTML, and that the id is correctly passed to the createPortal
function in the React code.
That’s it.
hope you find it help full.
Happy coding!
want to give suggestions:-
Email me at nidhisharma639593@gmail.com
Subscribe to my newsletter
Read articles from nidhi sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
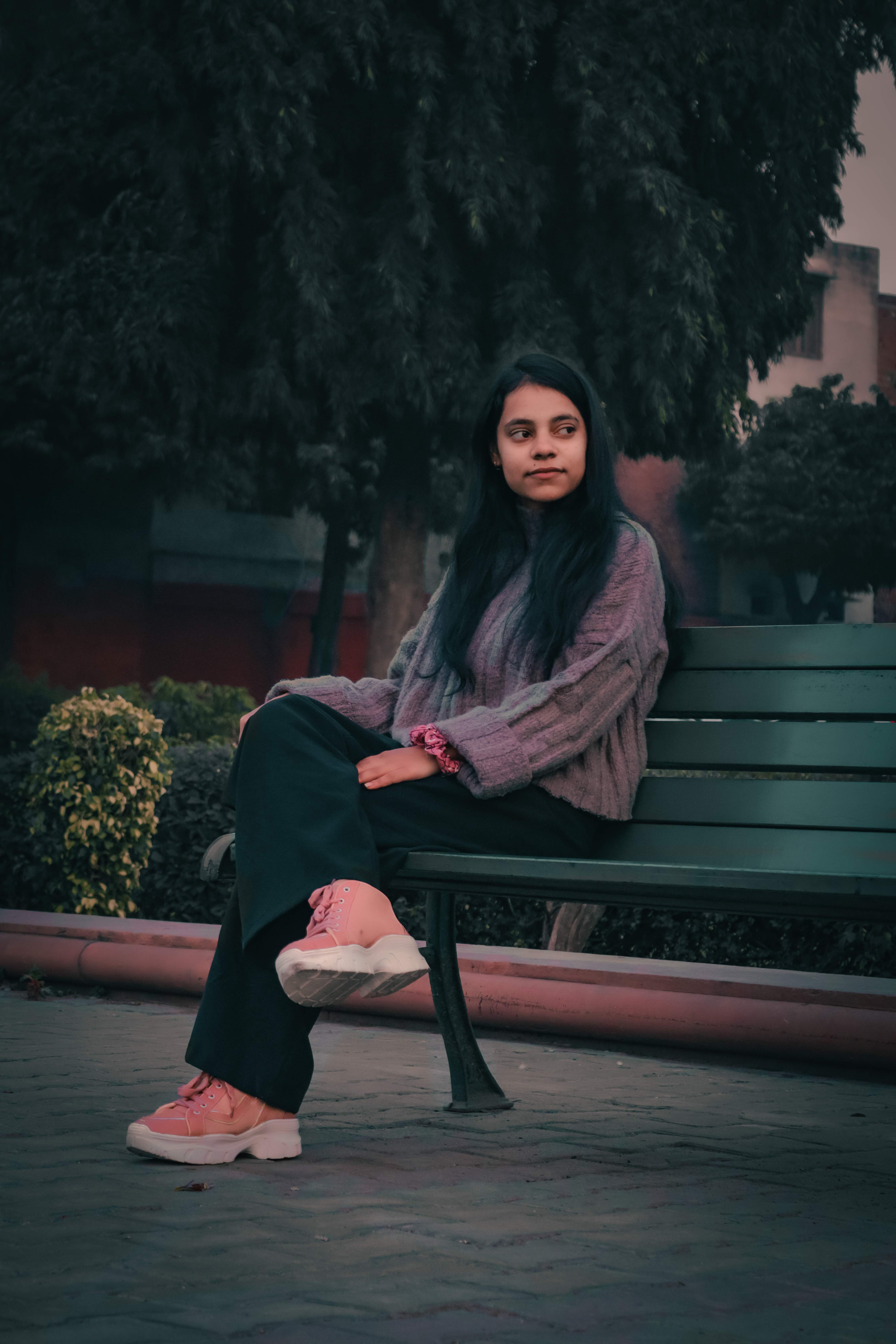
nidhi sharma
nidhi sharma
Hi there! My name is Nidhi sharma and I am a frontend developer currently working as an intern. I have a strong passion for creating user-friendly and visually appealing websites and applications, and I am always looking to learn and improve my skills. As a frontend developer, I use a variety of programming languages and tools, such as HTML, CSS, and JavaScript, React, to bring my designs to life and make them interactive and responsive. I am excited to have the opportunity to learn from and contribute to a team of experienced developers. I am eager to take on new challenges and to apply my skills and knowledge to real-world projects.